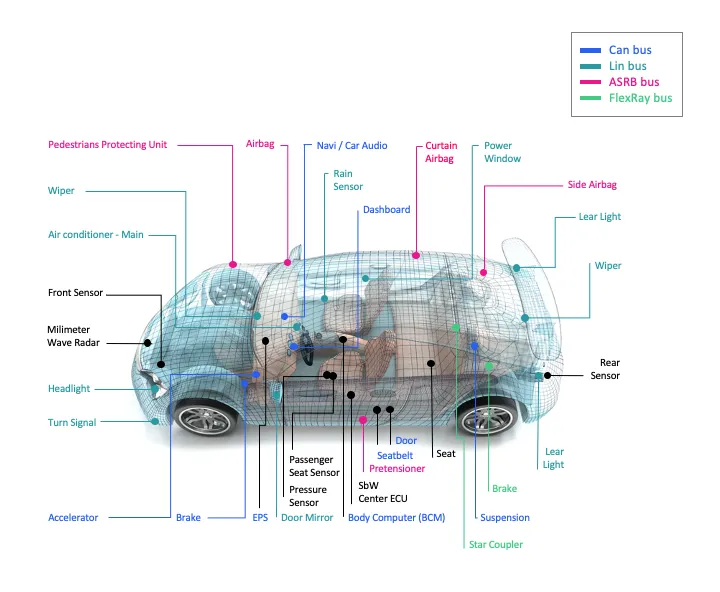
Android’s Vehicle Hardware Abstraction Layer (HAL) is a crucial component that facilitates communication between Android applications and the various sensors and signals within a vehicle. The Vehicle HAL stores information in the form of Vehicle Properties, which are often associated with signals on the vehicle bus. This blog will delve into the fundamental aspects of the Vehicle HAL, including Vehicle Properties, System Property Identifiers, extending VehicleProperty, and the essential functions defined in IVehicle.
Vehicle Hardware Abstraction Layer (Vehicle HAL / VHAL)
The Vehicle Hardware Abstraction Layer (VHAL) is like a bridge between Android software and the hardware inside a vehicle. It helps Android applications communicate with the different sensors and functions in a standardized way.
Think of the VHAL as a set of rules that the vehicle follows, telling it how to communicate with Android. These rules are called “properties.” Each property represents a specific function or piece of information inside the vehicle.
For example, one property could be the vehicle’s speed, another property could be the temperature setting for the heating system, and so on.
Properties have certain characteristics, like whether they contain whole numbers (integers) or decimal numbers (floats) and how they can be changed or accessed.
There are different ways to interact with properties:
- Read: You can ask the vehicle for the current value of a property. For instance, you can read the speed property to know how fast the vehicle is going.
- Write: You can set the value of a property programmatically. For example, you can write a new temperature setting to control the vehicle’s heating system.
- Subscribe: You can subscribe to changes in a property, which means you will get notified whenever that property’s value changes. For instance, if you subscribe to the speed property, you will receive updates whenever the vehicle’s speed changes.
The VHAL interface provides a list of properties that vehicle manufacturers (OEMs) can implement in their vehicles. It also contains information about each property, such as what type of data it holds (int or float) and what change modes are allowed (e.g., reading, writing, or subscribing).
By following the VHAL rules and using properties, Android applications can communicate with the vehicle’s hardware without needing to know the specific details of each vehicle model. This abstraction makes it easier for developers to create apps that work across different types of vehicles, improving compatibility and user experience.
Vehicle properties
Vehicle Properties are pieces of information that represent various aspects of a vehicle’s hardware and functionality. Each property is uniquely identified by an integer (int32) key, which acts as its unique identifier.
Read-Only Properties
- Definition: Read-only properties are those from which you can only retrieve information; you cannot change their values.
- Usage: These properties provide information about the vehicle’s state or status, such as its current speed or fuel level.
- Examples: The vehicle’s speed (FLOAT type), engine status (BOOLEAN type), or current timestamp (EPOCH_TIME type) are read-only properties.
Write-Only Properties
- Definition: Write-only properties are used to send information to the Vehicle HAL; you cannot read their values.
- Usage: These properties allow applications to pass data to the vehicle’s hardware or control certain functionalities.
- Examples: Sending a command to turn on the headlights or adjusting the HVAC(Heating, Ventilation and Air Conditioning)system’s fan speed are actions facilitated by write-only properties.
Read-Write Properties
- Definition: Read-write properties support both reading and writing operations, allowing you to both retrieve and change their values.
- Usage: These properties enable two-way communication between applications and the vehicle’s hardware.
- Examples: Setting the target temperature for the HVAC system (FLOAT type), adjusting the volume of the audio system (INT32 type), or configuring custom preferences (STRING type) are read-write properties.
Value Types
Vehicle Properties can have different value types, indicating the data format they use to store information. Some common value types include:
- BYTES: Represents a sequence of raw binary data.
- BOOLEAN: Represents a true/false value.
- EPOCH_TIME: Represents a timestamp in the Unix Epoch time format (number of seconds since January 1, 1970).
- FLOAT: Represents a single decimal number.
- FLOAT[]: Represents an array of decimal numbers.
- INT32: Represents a single whole number (integer).
- INT32[]: Represents an array of whole numbers (integers).
- INT64: Represents a single large whole number (long integer).
- INT64[]: Represents an array of large whole numbers (long integers).
- STRING: Represents a sequence of characters, such as text or words.
- MIXED: Represents a combination of different data types within a single property.
Zoned Properties
Some properties are zoned, meaning they can have multiple values based on the number of zones supported. For example, a zoned property related to tire pressure may have different values for each tire. Zoned properties help account for variations across different parts of the vehicle.
Vehicle Properties in the Vehicle HAL can be read-only, write-only, or read-write, and each property has a specific value type associated with it. Zoned properties allow for multiple values based on the number of zones supported, catering to various parts or areas of the vehicle. Together, these properties facilitate seamless communication between Android applications and the vehicle’s hardware, enabling better control and monitoring of vehicle functionalities.
Area Types
Area Types in the Vehicle HAL define different areas within the vehicle, such as windows, mirrors, seats, doors, and wheels. Each area type serves as a category to organize and address specific parts of the vehicle.
The available Area Types are as follows:
- GLOBAL: This area type represents a singleton area, which means it is a single entity with no multiple sub-areas.
- WINDOW: This area type is based on windows and uses the VehicleAreaWindow enumeration to identify different window areas, such as front and rear windows.
- MIRROR: This area type is based on mirrors and uses the VehicleAreaMirror enumeration to identify different mirror areas, such as left and right mirrors.
- SEAT: This area type is based on seats and uses the VehicleAreaSeat enumeration to identify different seat areas, such as the front and rear seats.
- DOOR: This area type is based on doors and uses the VehicleAreaDoor enumeration to identify different door areas, such as front and rear doors.
- WHEEL: This area type is based on wheels and uses the VehicleAreaWheel enumeration to identify different wheel areas, such as the front-left and rear-right wheels.
Each zoned property must use a pre-defined area type, and each area type has a set of bit flags defined in its respective enum (e.g., VehicleAreaSeat has flags like ROW_1_LEFT, ROW_1_CENTER, ROW_1_RIGHT, etc.).
For example, the SEAT
area defines VehicleAreaSeat
enums:
ROW_1_LEFT = 0x0001
ROW_1_CENTER = 0x0002
ROW_1_RIGHT = 0x0004
ROW_2_LEFT = 0x0010
ROW_2_CENTER = 0x0020
ROW_2_RIGHT = 0x0040
ROW_3_LEFT = 0x0100
- …
Area IDs
Zoned properties are addressed through Area IDs, which represent specific combinations of flags from their respective enum. Each zoned property may support one or more Area IDs to define the relevant areas within the vehicle.
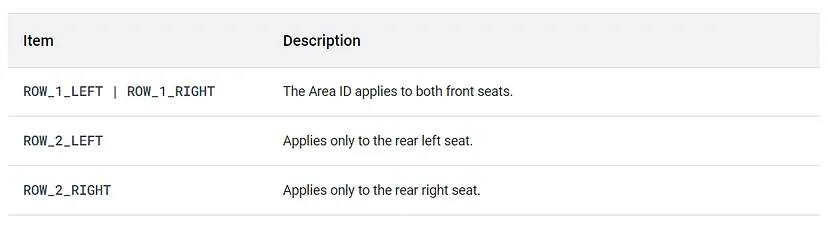
For example, if a property uses the VehicleAreaSeat area type, it might use the following Area IDs:
- ROW_1_LEFT | ROW_1_RIGHT: This Area ID applies to both front seats, combining the flags for the left and right seats in the first row.
- ROW_2_LEFT: This Area ID applies only to the rear left seat in the second row.
- ROW_2_RIGHT: This Area ID applies only to the rear right seat in the second row.
By using Area IDs, zoned properties can target specific areas within the vehicle, allowing for more granular control and monitoring of different parts of the vehicle separately.
Property Status
Property Status in the Vehicle HAL indicates the current condition of a property’s value. Each property value is accompanied by a VehiclePropertyStatus value, which informs about the property’s availability and validity.
Every property value comes with a VehiclePropertyStatus
value. This indicates the current status of the property:
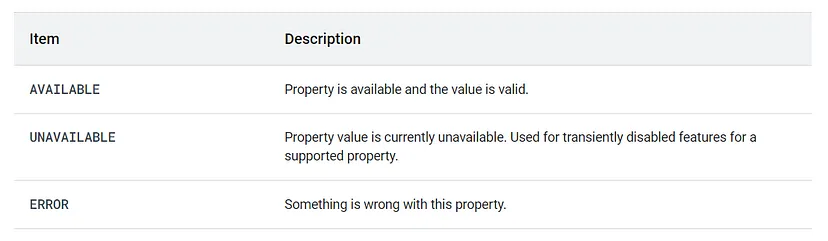
Available Values of VehiclePropertyStatus:
- AVAILABLE: This status indicates that the property is supported by the vehicle and the current value is valid and accessible. It means the property is ready to be read or written to.
- UNAVAILABLE: When a property has the UNAVAILABLE status, it means the property value is currently unavailable or not accessible. This status is typically used for transient conditions, where a supported property might be temporarily disabled or unavailable. It is not meant to indicate that the property is unsupported in general.
- ERROR: The ERROR status indicates that something is wrong with the property. This status might be used when there is a problem with retrieving or setting the property’s value, such as a communication issue or an internal error.
Important Note: It is essential to remember that if a property is not supported by the vehicle, it should not be included in the Vehicle HAL at all. In other words, unsupported properties should not be part of the VHAL interface. It is not acceptable to set the property status to UNAVAILABLE permanently just to denote an unsupported property.
Configuring a property
Configuring a property in the Vehicle HAL involves using the VehiclePropConfig structure to provide important configuration information for each property. This information includes various variables that help define how the property can be accessed, monitored, and controlled.
Use VehiclePropConfig
to provide configuration information for each property. Information includes:
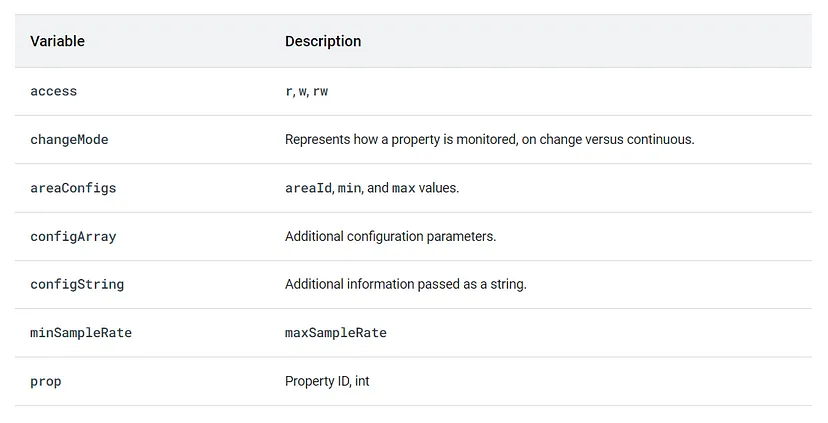
Below are the details of the variables used in the configuration:
access
- Description: The ‘access’ variable specifies the type of access allowed for the property.
- Values: It can be set to one of the following:
1. Read-only access (Value: ‘r’):
- Description: The property can be read but not modified.
- Access Type: Read-only.
2. Write-only access (Value: ‘w’):
- Description: The property can be written but not read.
- Access Type: Write-only.
3. Read-write access (Value: ‘rw’):
- Description: The property supports both reading and writing.
- Access Type: Read-write.
changeMode
- Description: The ‘changeMode’ variable represents how the property is monitored for changes.
- Values: It can be set to either ‘ON_CHANGE’ or ‘CONTINUOUS.’
- ‘ON_CHANGE’: The property triggers an event only when its value changes.
- ‘CONTINUOUS’: The property is constantly changing, and the subscriber is notified at the sampling rate set.
areaConfigs
- Description: The ‘areaConfigs’ variable contains configuration information for different areas associated with the property.
- Information: It includes areaId, min, and max values.
- areaId: Represents the Area ID associated with the property (e.g., ROW_1_LEFT, ROW_2_RIGHT).
- min: Specifies the minimum valid value for the property.
- max: Specifies the maximum valid value for the property.
configArray
- Description: The ‘configArray’ variable is used to hold additional configuration parameters for the property.
- Information: It can store an array of specific data related to the property.
configString
- Description: The ‘configString’ variable is used to provide additional information related to the property as a string.
- Information: It can hold any extra details or specifications for the property.
minSampleRate, maxSampleRate
- Description: These variables specify the minimum and maximum sampling rates (measurement frequency) for the property when monitoring changes.
- Information: They define how often the property values are checked for updates.
prop
- Description: The ‘prop’ variable is the Property ID, an integer that uniquely identifies the property in the Vehicle HAL.
- Information: Each property in the Vehicle HAL is assigned a specific Property ID, which acts as its unique identifier. This ID is used to access, configure, and interact with the property within the Vehicle HAL interface. It ensures that each property can be referenced uniquely, even if there are multiple properties with similar or related functionalities.
System Property Identifiers
System Property Identifiers in the Vehicle HAL are unique labels used to categorize and identify specific properties. They are marked with the tag “VehiclePropertyGroup:SYSTEM” to distinguish them from other types of properties.
In Android 12, there are more than 150 such identifiers. Each identifier represents a different property related to the vehicle’s system and functionalities. For example, one of these identifiers is “HVAC_TEMPERATURE_SET,” which stands for the target temperature set for the vehicle’s HVAC system.
Let’s break down the details of the “HVAC_TEMPERATURE_SET” identifier:
- Property Name: HVAC_TEMPERATURE_SET
- Description: Represents the target temperature set for the HVAC (Heating, Ventilation, and Air Conditioning) system in the vehicle.
- Change Mode: The property is monitored in the “ON_CHANGE” mode, which means an event is triggered whenever the target temperature changes.
- Access: The property can be both read and written, allowing applications to retrieve the current target temperature and update it programmatically.
- Unit: The temperature values are measured in Celsius (°C).
System Property Identifiers in the Vehicle HAL are unique labels that categorize different properties related to the vehicle’s system. They provide standardized access to various functionalities, such as setting the target temperature for the HVAC system. By using these identifiers, Android applications can seamlessly interact with the vehicle’s hardware, enhancing user experience and control over various vehicle features.
Handling zone properties
Handling zone properties involves dealing with collections of multiple properties, where each part can be accessed using a specific Area ID value. Here’s how the different calls work:
Get Calls:
- When you make a “get” call for a zoned property, you must include the Area ID in the request.
- As a result, only the current value for the requested Area ID is returned.
- If the property is global (applies to all zones), the Area ID is set to 0.
Set Calls:
- For a “set” call on a zoned property, you need to specify the Area ID.
- This means that only the value for the requested Area ID will be changed.
Subscribe Calls:
- A “subscribe” call generates events for all Area IDs associated with the property.
- This means that whenever there is a change in any Area ID’s value, the subscribed function will be notified.
When dealing with zoned properties, using the Area ID allows you to access specific parts of the collection. “Get” calls return the current value for a specified Area ID, “Set” calls change the value for a requested Area ID, and “Subscribe” calls generate events for all Area IDs associated with the property.
Now, let’s look at specific scenarios for Get and Set calls:
Get Calls
- During initialization, the value of the property may not be available yet due to pending vehicle network messages. In this case, the “get” call should return a special code, -EAGAIN, indicating that the value is not available yet.
- Some properties, like HVAC (Heating, Ventilation, and Air Conditioning), have separate power properties to turn them on/off. When you “get” a property like HVAC Temperature and it’s powered off, it should return a status of UNAVAILABLE instead of an error.
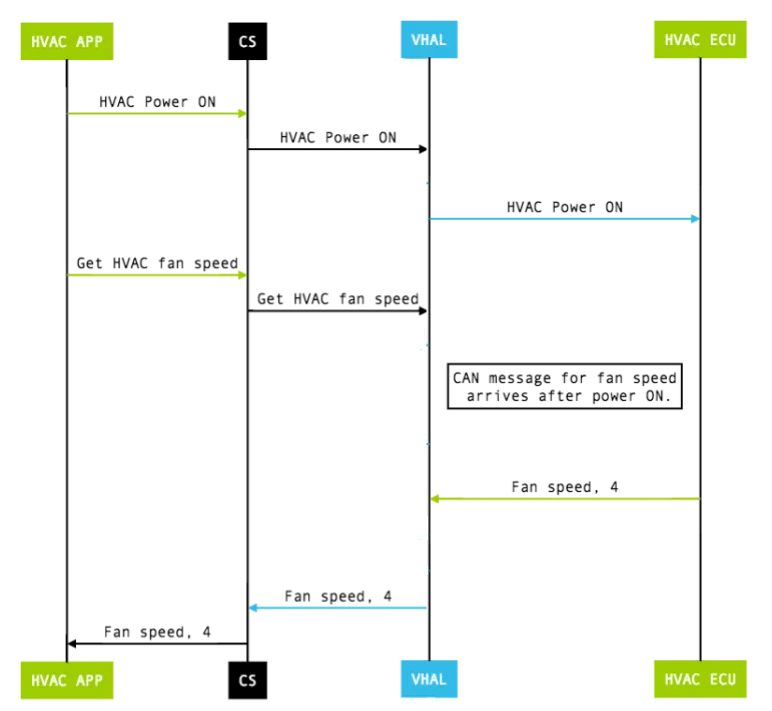
Set Calls
- A “set” call usually triggers a change request across the vehicle network. It’s ideally an asynchronous operation, returning as soon as possible, but it can also be synchronous if needed.
- In some cases, a “set” call might require initial data that isn’t available during initialization. In such situations, the “set” call should return StatusCode#TRY_AGAIN to indicate that you should try again later.
- For properties with separate power states (on and off), if the property is powered off and the “set” can’t be done, it should return StatusCode#NOT_AVAILABLE or StatusCode#NOT_AVAILABLE_DISABLED.
- Until the “set” operation is complete and effective, the “get” call might not necessarily return the same value as what was set. For example, if you “set” the HVAC Temperature, the “get” call might not immediately reflect the new value until the change takes effect.
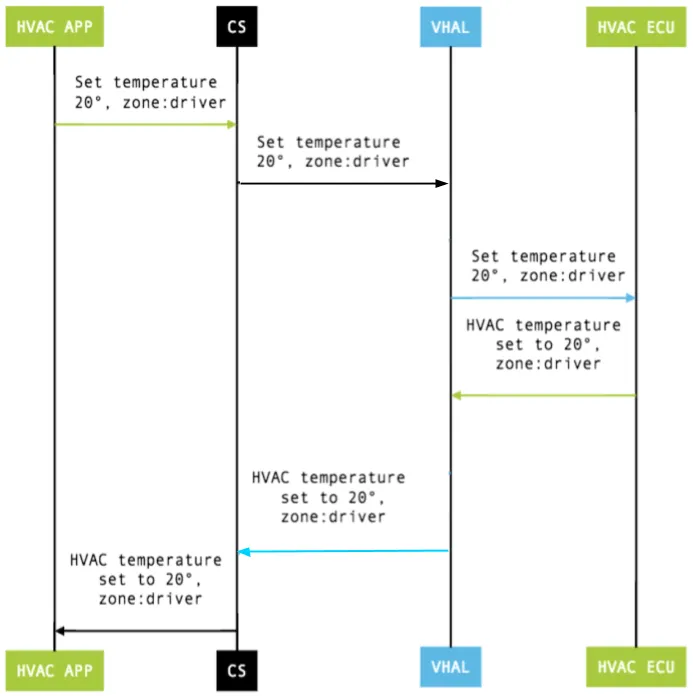
Handling custom properties
To support partner-specific needs, the VHAL allows custom properties that are restricted to system apps. Use the following guidelines when working with custom properties:
Property ID Generation:
- Use the following format to generate the Property ID: VehiclePropertyGroup:VENDOR.
- The VENDOR group should be used exclusively for custom properties.
Vehicle Area Type:
- Select an appropriate area type(VehicleArea type) that best represents the scope of the custom property within the vehicle.
Vehicle Property Type:
- Choose the proper data type for the custom property.
- For most cases, the BYTES type is sufficient, allowing the passing of raw data.
- Be cautious when adding a big payload, as frequently sending large data through custom properties can slow down the entire vehicle network access.
Property ID Format:
- Choose a four-nibble ID for the custom property.
- The format should consist of four hexadecimal characters.
Avoid Replicating Existing Vehicle Properties:
- To prevent ecosystem fragmentation, do not use custom properties to replicate vehicle properties that already exist in the VehiclePropertyIds SDK.
- In the VehiclePropConfig.configString field, provide a short description of the custom property. This helps sanity check tools flag accidental replication of existing vehicle properties. For example, you can use a description like “hazard light state.”
Accessing Custom Properties:
- Access custom properties through CarPropertyManager for Java components or through the Vehicle Network Service API for native components.
- Avoid modifying other car APIs to prevent future compatibility issues.
Permissions for Vendor Properties:
- After implementing vendor properties, select only the permissions list in the VehicleVendorPermission enum for vendor properties.
- Avoid mapping vendor permissions to system properties to prevent breaking the Compatibility Test Suite (CTS) and Vendor Test Suite (VTS).
By following these guidelines, you can create and manage custom properties in the VHAL effectively while ensuring compatibility and preventing fragmentation within the ecosystem.
Handling HVAC properties
Handling HVAC properties in the VHAL (Vehicle Hardware Abstraction Layer) involves controlling various aspects of the HVAC system in a vehicle. Most HVAC properties are zoned properties, meaning they can be controlled separately for different zones or areas in the vehicle. However, some properties are global, affecting the entire vehicle’s HVAC system.
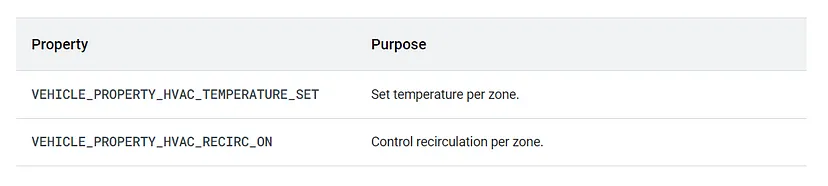
Above two sample-defined HVAC properties are:
VEHICLE_PROPERTY_HVAC_TEMPERATURE_SET
: This property is used to set the temperature per zone in the vehicle.VEHICLE_PROPERTY_HVAC_RECIRC_ON
: This property is used to control recirculation per zone.
To see a complete list of available HVAC properties, you can search for properties starting with VEHICLE_PROPERTY_HVAC_*
in the types.hal
file.
When the HVAC property uses VehicleAreaSeat
, there are additional rules for mapping a zoned HVAC property to Area IDs. Each available seat in the car must be part of an Area ID in the Area ID array.
Let’s take two examples to better understand how to map HVAC_TEMPERATURE_SET to Area IDs:
Example One:
- Car Configuration: The car has two front seats (ROW_1_LEFT, ROW_1_RIGHT) and three back seats (ROW_2_LEFT, ROW_2_CENTER, ROW_2_RIGHT).
- HVAC Units: The car has two temperature control units: one for the driver side and one for the passenger side.
A valid mapping set of Area IDs for HVAC_TEMPERATURE_SET is:
- Driver side temperature control: ROW_1_LEFT | ROW_2_LEFT
- Passenger side temperature control: ROW_1_RIGHT | ROW_2_CENTER | ROW_2_RIGHT
An alternative mapping for the same hardware configuration is:
- Driver side temperature control: ROW_1_LEFT | ROW_2_LEFT | ROW_2_CENTER
- Passenger side temperature control: ROW_1_RIGHT | ROW_2_RIGHT
Example Two:
- Car Configuration: The car has three seat rows with two seats in the front row (ROW_1_LEFT, ROW_1_RIGHT), three seats in the second row (ROW_2_LEFT, ROW_2_CENTER, ROW_2_RIGHT), and three seats in the third row (ROW_3_LEFT, ROW_3_CENTER, ROW_3_RIGHT).
- HVAC Units: The car has three temperature control units: one for the driver side, one for the passenger side, and one for the rear.
A reasonable way to map HVAC_TEMPERATURE_SET to Area IDs is as a three-element array:
- Driver side temperature control: ROW_1_LEFT
- Passenger side temperature control: ROW_1_RIGHT
- Rear temperature control: ROW_2_LEFT | ROW_2_CENTER | ROW_2_RIGHT | ROW_3_LEFT | ROW_3_CENTER | ROW_3_RIGHT
Keep in mind that the exact mapping of HVAC properties to Area IDs may vary based on the vehicle’s hardware configuration and the HVAC system’s design. The examples provided above demonstrate how different seat configurations and HVAC units can influence the mapping of HVAC properties to specific zones in the vehicle.
Handling sensor properties
VHAL sensor properties are a way for apps to access real sensor data or policy information from the vehicle. Some sensor information, such as driving status and day/night mode, is accessible by any app without restriction. This is because this data is mandatory to build a safe vehicle application. Other sensor information, such as vehicle speed, is more sensitive and requires specific permissions that users can manage.
The supported sensor properties are defined in the types.hal
file. This file lists all of the available sensor properties, along with their type, access permissions, and other metadata.
To access a VHAL sensor property, an app must first obtain a reference to the IVehicle
interface. This interface provides methods for reading, writing, and subscribing to sensor properties.
Once the app has a reference to the IVehicle
interface, it can use the get
method to read the value of a sensor property. The get
method takes the property ID as an argument and returns the value of the property.
The app can also use the set
method to write the value of a sensor property. The set
method takes the property ID and the new value as arguments.
To subscribe to a sensor property, the app can use the subscribe
method. The subscribe
method takes the property ID and a callback as arguments. The callback will be invoked whenever the value of the property changes.
Here is an example of how to access a VHAL sensor property:
// Get a reference to the IVehicle interface.
IVehicle vehicle = VehicleManager.getVehicle();
// Get the value of the driving status property.
int drivingStatus = vehicle.get(VehiclePropertyIds.DRIVING_STATUS);
// If the vehicle is driving, turn on the headlights.
if (drivingStatus == 1) {
vehicle.set(VehiclePropertyIds.HEADLIGHTS, 1);
}
HAL interfaces
The Vehicle Hardware Abstraction Layer (VHAL) is a HAL interface that allows apps to access vehicle properties. The VHAL provides a number of interfaces that can be used to read, write, and subscribe to vehicle properties.
The getAllPropConfigs()
interface returns a list of all the properties that are supported by the VHAL. The getPropConfigs()
interface returns the configuration of a specific property. The set()
interface allows you to write a value to a property. The subscribe()
interface allows you to subscribe to a property so that you are notified when its value changes.
The VHAL also provides two callback interfaces: onPropertyEvent()
and onPropertySetError()
. The onPropertyEvent()
interface is called whenever the value of a property that you are subscribed to changes. The onPropertySetError()
interface is called if an error occurs when you try to set the value of a property.
Here is just a recap of the above example of how to use the VHAL to read the value of the driving status property:
// Get a reference to the IVehicle interface.
IVehicle vehicle = VehicleManager.getVehicle();
// Get the value of the driving status property.
int drivingStatus = vehicle.get(VehiclePropertyIds.DRIVING_STATUS);
Here is a brief explanation of the HAL interfaces:
VHAL Interfaces:
IVehicle.hal file
Please note that the below .hal files are not Java, C++ or scss files (I selected auto mode so it will take Java, C++, or scss)
BTW, What is .hal file?
A .hal file is a Hardware Abstraction Layer (HAL) file that defines the interface between a hardware device and the Android operating system. HAL files are written in the Hardware Interface Description Language (HIDL), which is a language for describing hardware interfaces in a platform-independent way.
package [email protected]; import IVehicleCallback; interface IVehicle { /** * Returns a list of all property configurations supported by this vehicle * HAL. */ getAllPropConfigs() generates (vec<VehiclePropConfig> propConfigs); /** * Returns a list of property configurations for given properties. * * If requested VehicleProperty wasn't found it must return * StatusCode::INVALID_ARG, otherwise a list of vehicle property * configurations with StatusCode::OK */ getPropConfigs(vec<int32_t> props) generates (StatusCode status, vec<VehiclePropConfig> propConfigs); /** * Get a vehicle property value. * * For VehiclePropertyChangeMode::STATIC properties, this method must always * return the same value always. * For VehiclePropertyChangeMode::ON_CHANGE properties, it must return the * latest available value. * * Some properties like AUDIO_VOLUME requires to pass additional data in * GET request in VehiclePropValue object. * * If there is no data available yet, which can happen during initial stage, * this call must return immediately with an error code of * StatusCode::TRY_AGAIN. */ get(VehiclePropValue requestedPropValue) generates (StatusCode status, VehiclePropValue propValue); /** * Set a vehicle property value. * * Timestamp of data must be ignored for set operation. * * Setting some properties require having initial state available. If initial * data is not available yet this call must return StatusCode::TRY_AGAIN. * For a property with separate power control this call must return * StatusCode::NOT_AVAILABLE error if property is not powered on. */ set(VehiclePropValue propValue) generates (StatusCode status); /** * Subscribes to property events. * * Clients must be able to subscribe to multiple properties at a time * depending on data provided in options argument. * * @param listener This client must be called on appropriate event. * @param options List of options to subscribe. SubscribeOption contains * information such as property Id, area Id, sample rate, etc. */ subscribe(IVehicleCallback callback, vec<SubscribeOptions> options) generates (StatusCode status); /** * Unsubscribes from property events. * * If this client wasn't subscribed to the given property, this method * must return StatusCode::INVALID_ARG. */ unsubscribe(IVehicleCallback callback, int32_t propId) generates (StatusCode status); /** * Print out debugging state for the vehicle hal. * * The text must be in ASCII encoding only. * * Performance requirements: * * The HAL must return from this call in less than 10ms. This call must avoid * deadlocks, as it may be called at any point of operation. Any synchronization * primitives used (such as mutex locks or semaphores) must be acquired * with a timeout. * */ debugDump() generates (string s); };
getAllPropConfigs()
:
This interface returns a list of all the properties that are supported by the VHAL. This list includes the property ID, property type, and other metadata.
- Generates
(vec<VehiclePropConfig> propConfigs)
. - Lists the configuration of all properties supported by the VHAL.
- CarService uses supported properties only.
getPropConfigs(vec<int32_t> props)
:
This interface returns the configuration of a specific property. The configuration includes the property ID, property type, access permissions, and other metadata.
- Generates
(StatusCode status, vec<VehiclePropConfig> propConfigs)
. - Returns the configuration of selected properties.
- Allows querying the configuration of specific properties.
set(VehiclePropValue propValue)
:
This interface allows you to write a value to a property. The value that you write must be of the correct type for the property.
- Generates
(StatusCode status)
. - Writes a value to a property.
- The result of the write operation is defined per property.
subscribe(IVehicleCallback callback, vec<SubscribeOptions> options)
:
This interface allows you to subscribe to a property so that you are notified when its value changes. The callback that you provide will be called whenever the value of the property changes.
- Generates
(StatusCode status)
. - Starts monitoring a property value change.
- For zoned properties, there is an additional
unsubscribe(IVehicleCallback callback, int32_t propId)
method to stop monitoring a specific property for a given callback.
VHAL Callback Interfaces:
IVehicleCallback.hal
package [email protected]; interface IVehicleCallback { /** * Event callback happens whenever a variable that the API user has * subscribed to needs to be reported. This may be based purely on * threshold and frequency (a regular subscription, see subscribe call's * arguments) or when the IVehicle#set method was called and the actual * change needs to be reported. * * These callbacks are chunked. * * @param values that has been updated. */ oneway onPropertyEvent(vec<VehiclePropValue> propValues); /** * This method gets called if the client was subscribed to a property using * SubscribeFlags::SET_CALL flag and IVehicle#set(...) method was called. * * These events must be delivered to subscriber immediately without any * batching. * * @param value Value that was set by a client. */ oneway onPropertySet(VehiclePropValue propValue); /** * Set property value is usually asynchronous operation. Thus even if * client received StatusCode::OK from the IVehicle::set(...) this * doesn't guarantee that the value was successfully propagated to the * vehicle network. If such rare event occurs this method must be called. * * @param errorCode - any value from StatusCode enum. * @param property - a property where error has happened. * @param areaId - bitmask that specifies in which areas the problem has * occurred, must be 0 for global properties */ oneway onPropertySetError(StatusCode errorCode, int32_t propId, int32_t areaId); };
After seeing this file you might be wondering about, what is a oneway method.
A oneway method in a HAL file is a method that does not require a response from the hardware device. Oneway methods are typically used for asynchronous operations, such as sending a command to the hardware device or receiving a notification from the hardware device.
Here is an example of a oneway method in a HAL file:
oneway void setBrightness(int brightness);
This method sets the brightness of the hardware device to the specified value. The method does not require a response from the hardware device, so the caller does not need to wait for the method to complete before continuing.
Oneway methods are often used in conjunction with passthrough HALs. Passthrough HALs are HALs that run in the same process as the calling application. This means that oneway methods in passthrough HALs can be invoked directly by the calling application, without the need for a binder call.
onPropertyEvent(vec<VehiclePropValue> propValues)
:
This callback is called whenever the value of a property that you are subscribed to changes. The callback will be passed a list of the properties that have changed and their new values.
- A one-way callback function.
- Notifies vehicle property value changes to registered callbacks.
- This function should be used only for properties that have been subscribed to for monitoring.
onPropertySetError(StatusCode errorCode, int32_t propId, int32_t areaId)
:
This callback is called if an error occurs when you try to set the value of a property. The callback will be passed the error code and the property ID that was being set.
- A one-way callback function.
- Notifies errors that occurred during property write operations.
- The error can be related to the VHAL level or specific to a property and an area (in the case of zoned properties).
These interfaces and callbacks form the core communication mechanism between the VHAL and other components, such as CarService and applications, allowing for the configuration, querying, writing, and monitoring of vehicle properties. The usage of these interfaces may vary depending on the specific implementation of the VHAL in different systems or platforms.
Properties Monitoring and Notification
In the context of the Vehicle Hardware Abstraction Layer (VHAL) and its properties, the IVehicle::subscribe
method and IVehicleCallback::onChange
callback are used for monitoring changes in vehicle properties. Additionally, there is a ChangeMode
enum that defines how the properties behave in terms of their update frequency.
IVehicle::subscribe
- The
IVehicle::subscribe
method is used to register a callback (implementingIVehicleCallback
) to receive updates when the subscribed properties change. - This method allows applications to start monitoring specific vehicle properties for value changes.
IVehicleCallback::onChange
- The
IVehicleCallback::onChange
callback function is invoked when there are updates to the subscribed properties. - When a property changes and the VHAL detects the change, it notifies all registered callbacks using this callback function.
ChangeMode
Enum
- The
ChangeMode
enum defines how a particular property behaves in terms of its update frequency. It has the following possible values: STATIC
: The property never changes.ON_CHANGE
: The property only signals an event when its value changes.CONTINUOUS
: The property constantly changes and is notified at a sampling rate set by the subscriber.
These definitions allow applications to subscribe to properties with different update behaviors based on their specific needs. For example, if an application is interested in monitoring the vehicle speed, it may subscribe to the speed property with the CONTINUOUS
change mode to receive a continuous stream of speed updates at a certain sampling rate. On the other hand, if an application is interested in the vehicle’s daytime/nighttime mode, it may subscribe with the ON_CHANGE
change mode to receive updates only when the mode changes from day to night or vice versa.
The use of these definitions and methods allows for efficient monitoring and notification of changes in vehicle properties, ensuring that applications can stay up-to-date with the latest data from the vehicle’s sensors and systems.
Conclusion
The Vehicle HAL is a critical component of the Android operating system that facilitates seamless communication between Android applications and a vehicle’s hardware and sensors. By utilizing Vehicle Properties and the various functions defined in the IVehicle interface, developers can access and control essential aspects of a vehicle’s state and functioning. Furthermore, the ability to extend Vehicle Properties using custom identifiers offers developers the flexibility to tailor their applications to specific vehicle hardware and functionalities, thereby enhancing the overall user experience. As Android continues to evolve, the Vehicle HAL is expected to play an even more significant role in shaping the future of automotive technology.