Jetpack Compose, the modern Android UI toolkit, has revolutionized the way developers build user interfaces for Android applications. One of the powerful features that Compose provides is the getApplicationContext
function, commonly referred to as “Get Context.” In this blog post, we will delve into the intricacies of Get Context in Jetpack Compose and explore how it can be harnessed to enhance the development experience.
Understanding Get Context
In Android development, the application context serves as a global context for an application. It allows components to access resources, services, and other application-related information. In Jetpack Compose, the getApplicationContext
function provides a straightforward way to obtain the application context within the Composable functions.
Usage of Get Context
In Android Jetpack Compose, you can get the context by using LocalContext, but it should be called from the composable function only or within its scope.
val context = LocalContext.current
Let’s see one example to clarify the things
@Composable
fun ToastDisplay(name: String) {
val ctx = LocalContext.current
Column(
Modifier
.fillMaxHeight()
.fillMaxWidth(), verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(text = "Hello $name", color = Color.Red,
modifier = Modifier
.background(Color.Green)
.clickable {
Toast
.makeText(ctx, "Welcome to the Compose World", Toast.LENGTH_SHORT)
.show()
})
}
}
In the above code snippet, we are retrieving the context and showing a toast message inside the composable.
But if we use the LocalContext.current directly inside the clickable function results in the compilation error “@composable invocations can only happen from the context of an @composable function”.
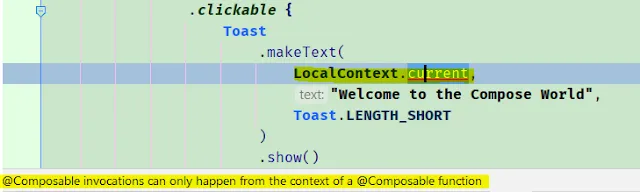
Since the LocalContext.current is composable, you can’t invoke it within the non-composable function. i.e. clickable function is not a composable function and so can’t accept other composable functions.
Alternatively, you can get the context outside the clickable function scope and use it, as shown in the above code snippet.
Common Use Cases
Accessing Resources:
val stringResource = stringResource(id = R.string.app_name)
This example demonstrates how to use the context to access string resources defined in the res/values/strings.xml
file.
Retrieving System Services:
val connectivityManager = context.getSystemService(Context.CONNECTIVITY_SERVICE) as ConnectivityManager?
Here, we use the context to retrieve the ConnectivityManager
system service, enabling us to check the device’s network connectivity.
Launching Activities:
context.startActivity(Intent(context, AnotherActivity::class.java))
Get Context allows Compose developers to initiate activities, facilitating navigation within the application.
Using SharedPreferences:
val sharedPreferences = PreferenceManager.getDefaultSharedPreferences(context)
val storedValue = sharedPreferences.getString("key", "default")
Accessing shared preferences becomes seamless with Get Context, allowing for the retrieval of user-specific data.
Benefits of Get Context in Jetpack Compose
- Simplified Resource Access: Get Context streamlines the process of accessing resources, reducing boilerplate code and making the development workflow more efficient.
- Improved Code Organization: By obtaining the application context directly within Composable functions, the code becomes more organized and cohesive. Developers can access necessary resources without passing them through function parameters.
- Integration with Android Ecosystem: Leveraging the application context through Get Context ensures seamless integration with the broader Android ecosystem, enabling Compose applications to interact with system services and components.
Conclusion
Get Context in Jetpack Compose plays a pivotal role in enhancing the development experience by providing a simple and effective way to access the application context within Composable functions. Whether you’re retrieving resources, interacting with system services, or launching activities, Get Context simplifies these tasks, making your code more concise and readable. As you continue exploring Jetpack Compose, consider leveraging Get Context to unlock its full potential and streamline your UI development process.