The automotive industry is rapidly evolving, and with the integration of technology into vehicles, the concept of Android Automotive has gained significant traction. Android Automotive is an operating system designed to run directly on vehicles’ infotainment systems, providing a seamless user experience. In this comprehensive blog, we’ll delve into the core components of Android Automotive, focusing on the Car Service and Car Manager aspects. Additionally, we’ll explore the opportunities and challenges in developing third-party apps for this platform.
The Vehicle Hardware Abstraction Layer (HAL)
At the core of the Car Data Framework lies the Vehicle Hardware Abstraction Layer (HAL), a foundational native service layer. The HAL acts as a bridge between the hardware of the vehicle and the software framework. Its primary role is to implement communication plugins tailored to collect specific vehicle data and map them to predefined Vehicle property types defined in types.hal. Notably, the types.hal file establishes a standardized list of property IDs recognized by the Google framework.
Customizing the HAL
The flexibility of the Car Data Framework allows customization of the HAL to accommodate unique hardware configurations. This involves extending or modifying the types.hal file to introduce product-specific property IDs. These custom property IDs are marked as VENDOR, indicating that they are subject to VENDOR level policy enforcement. In essence, this facilitates the management and access control of data that is specific to a particular product.
For example, Let’s define a new property VENDOR_FOO as a VENDOR Property with property id as 0xf100.
enum VehicleProperty: @2.0::VehicleProperty {
VENDOR_FOO= (
0xf100
| VehiclePropertyGroup:VENDOR
| VehiclePropertyType:STRING
| VehicleArea:GLOBAL),
};
// types.hal
In this code snippet, we’re defining a custom vehicle property named VENDOR_FOO
within the VehicleProperty
enumeration. Let’s break down each component:
enum VehicleProperty: @2.0::VehicleProperty { ... }
: This line declares an enumeration namedVehicleProperty
with a version annotation of@2.0::VehicleProperty
. It suggests that this enumeration is part of version 2.0 of the Vehicle HAL (Hardware Abstraction Layer).VENDOR_FOO = (...)
: This defines a specific property within the enumeration namedVENDOR_FOO
.0xf100
: This hexadecimal value,0xf100
, is the unique identifier assigned to theVENDOR_FOO
property. It distinguishes this property from others and can be used to reference it programmatically.| VehiclePropertyGroup:VENDOR
: This component indicates that the property belongs to theVENDOR
group. Vehicle property groups are used to categorize properties based on their purpose or functionality.| VehicleArea:GLOBAL
: This indicates that the property is applicable to the entire vehicle, encompassing all areas. It suggests that the property’s relevance is not limited to a specific part of the vehicle.| VehiclePropertyType:STRING
: This part specifies that the data type of the property isSTRING
. This suggests that the property holds text-based information.
In short, this code snippet defines a custom vehicle property named
VENDOR_FOO
. This property has a unique identifier of0xf100
, belongs to theVENDOR
group, holds text-based data of typeSTRING
, and is applicable to the entire vehicle.
Diverse Car-Related Services
Sitting atop the HAL is the framework layer, which provides a comprehensive set of services and APIs for applications to access vehicle data efficiently. The android.car package plays a pivotal role in this layer by offering the car service, which acts as a conduit to the Vehicle HAL.
The framework encompasses a diverse range of car-related services, each catering to specific subsystems within the vehicle:
- CarHVACManager: This service manages HVAC-related properties, allowing applications to interact with heating, ventilation, and air conditioning systems.
- CarSensorManager: Facilitates the handling of various sensor-related data, providing insights into the vehicle’s environment.
- CarPowerManager: Manages power modes and states, enabling applications to optimize power consumption.
- CarInputService: Captures button events, making it possible for applications to respond to user inputs effectively.
In addition to subsystem-specific services, the VehiclePropertyService offers a generic interface for querying and altering data associated with PropertyIDs.
Understanding Android’s Car Service
At its core, Android’s Car Service is a system service that encapsulates vehicle properties and exposes them through a set of APIs. These APIs serve as valuable resources for applications to access and utilize vehicle-related data seamlessly. Whether it’s information about the vehicle’s speed, fuel consumption, or tire pressure, the Car Service provides a standardized way for apps to interact with these metrics.
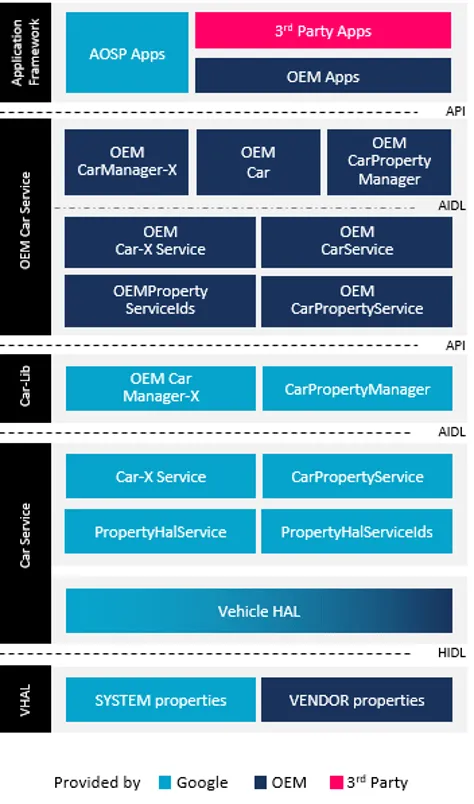
Implementation and Naming Conventions
The Car Service is implemented as a system service within the Android framework. It resides in a persistent system application named “com.android.car.” This naming convention ensures that the service is dedicated to handling vehicle-related functionalities without getting mixed up with other system or user apps.
To interact with the Car Service, developers can use the “android.car.ICar” interface. This interface defines the methods and communication protocols that allow applications to communicate with the Car Service effectively. By adhering to this interface, developers can ensure compatibility and seamless integration with the Android ecosystem.
Exploring the Inner Workings
To gain deeper insights into the Car Service’s functioning, the “dumpsys car_service” command proves to be invaluable. This command provides a detailed snapshot of the service’s current state, including active connections, APIs in use, and various operational metrics. Developers and enthusiasts can utilize this command to diagnose issues, monitor performance, and optimize their applications’ interactions with the Car Service.
The “-h” option of the “dumpsys car_service” command provides a list of available options, unlocking a plethora of diagnostic tools and information. This empowers developers to fine-tune their app’s interactions with the Car Service, ensuring a smooth user experience and efficient resource utilization.
Enhancing User Experience through Car Service APIs
The Car Service’s APIs offer a wide range of possibilities for enhancing the user experience within the vehicle. Applications can tap into these APIs to provide real-time information, create interactive dashboards, and even integrate voice commands for hands-free control. For instance, navigation apps can utilize the Car Service to display turn-by-turn directions on the vehicle’s infotainment system, while music apps can use it to provide a seamless playback experience.
Car Manager Interfaces: A Brief Overview
The Car Manager encompasses an array of 23 distinct interfaces, each tailored to manage specific aspects of the vehicle’s digital infrastructure. These interfaces serve as pathways through which different services and applications communicate, collaborate, and coexist harmoniously. From input management to diagnostic services, the Car Manager interfaces span a spectrum of functionalities that collectively enhance the driving experience.
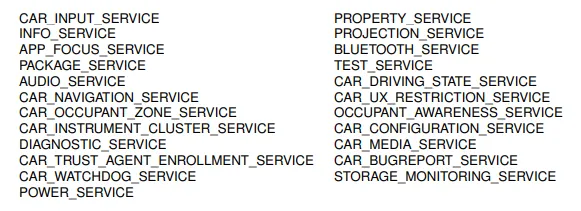
PROPERTY_SERVICE
The PROPERTY_SERVICE interface plays a crucial role in the Car Manager ecosystem. It serves as a gateway to access and manage various vehicle properties. These properties encompass a wide range of information, including vehicle speed, fuel level, engine temperature, and more. Applications and services can tap into this interface to gather real-time data, enabling them to offer users valuable insights into their vehicle’s performance.
Developers can utilize the PROPERTY_SERVICE interface to create engaging dashboard applications, present personalized notifications based on vehicle conditions, and even optimize driving behaviors by leveraging real-time data.
INFO_SERVICE
The INFO_SERVICE interface serves as an information hub within the Car Manager framework. It facilitates the exchange of data related to the vehicle’s status, health, and performance. This interface enables applications to access diagnostic information, maintenance schedules, and any potential issues detected within the vehicle.
By leveraging the INFO_SERVICE interface, developers can design applications that provide proactive maintenance reminders, offer detailed insights into the vehicle’s health, and assist drivers in making informed decisions about their vehicle’s upkeep.
CAR_UX_RESTRICTION_SERVICE
As safety and user experience take center stage in the automotive industry, the CAR_UX_RESTRICTION_SERVICE interface emerges as a critical player. This interface is designed to manage and enforce user experience restrictions while the vehicle is in motion. It ensures that applications adhere to safety guidelines, preventing distractions that could compromise the driver’s focus on the road.
By integrating the CAR_UX_RESTRICTION_SERVICE interface, developers can create applications that seamlessly adapt to driving conditions. This ensures that drivers are presented with relevant and non-distracting information, enhancing both safety and user experience.
Diving Deeper: Exploring PROPERTY_SERVICE Car Manager Interfaces
Let’s dive deep into the functionality of the PROPERTY_SERVICE interface, exploring its role, capabilities, and underlying mechanisms.
Understanding PROPERTY_SERVICE (CarPropertyManager)
The PROPERTY_SERVICE, also known as CarPropertyManager, plays a pivotal role in the Car Manager ecosystem. It acts as a simple yet powerful wrapper for the Vehicle Hardware Abstraction Layer (HAL) properties. This interface offers developers a standardized way to enumerate, retrieve, modify, and monitor vehicle properties. These properties encompass a wide range of data, including vehicle speed, fuel level, engine status, and more.
The key methods provided by the CarPropertyManager include:
- Enumerate: Developers can use this method to obtain a list of all available vehicle properties. This enables them to explore the diverse range of data points they can access and utilize within their applications.
- Get: The “get” method allows applications to retrieve the current value of a specific vehicle property. This real-time data access empowers developers to provide users with accurate and up-to-date information about their vehicle’s performance.
- Set: Developers can utilize the “set” method to modify the value of a vehicle property, facilitating the execution of specific commands or actions within the vehicle’s systems.
- Listen: The “listen” method enables applications to register listeners for specific vehicle properties. This functionality is particularly useful for creating real-time monitoring and notification systems.
Permissions and Security
One crucial aspect of the PROPERTY_SERVICE interface is its robust permission system. Access to vehicle properties is regulated, ensuring that applications adhere to strict security measures. Each property is associated with specific permissions that must be granted for an app to access it.
For instance, vendor-specific properties may require apps to possess the “PERMISSION_VENDOR_EXTENSION” permission at the “signature|privileged” level. This layered approach to permissions ensures that sensitive vehicle data remains protected and is only accessible to authorized applications.
Code and Implementation
The core functionality of the PROPERTY_SERVICE (CarPropertyManager) is implemented in the “CarPropertyManager.java” file, which resides within the “packages/services/Car/car-lib/src/android/car/hardware/property/” directory. This file encapsulates the methods, data structures, and logic required to facilitate seamless communication between applications and vehicle properties.
Diving Deeper: Exploring INFO_SERVICE Car Manager Interfaces
Let’s dive deep into the functionality of the INFO_SERVICE interface, exploring its role, capabilities, and underlying mechanisms.
Understanding INFO_SERVICE (CarInfoManager)
The INFO_SERVICE, more formally known as CarInfoManager, is a pivotal component within the Car Manager ecosystem. Its primary function is to facilitate the retrieval of static vehicle information, offering applications access to a wealth of data that encompasses various aspects of the vehicle’s identity and characteristics.
Key functionalities provided by the CarInfoManager include:
- Vehicle Identification (VID): The CarInfoManager enables applications to obtain a unique identifier for the vehicle. This identifier, known as the Vehicle Identification Number (VIN), plays a crucial role in differentiating individual vehicles and accessing specific information related to them.
- Model and Year: Developers can retrieve detailed information about the vehicle’s model and manufacturing year. This data provides context about the vehicle’s design, technology, and vintage.
- Fuel Type: The CarInfoManager allows applications to access information about the type of fuel the vehicle utilizes. This data is essential for creating applications that offer insights into fuel efficiency, emissions, and sustainability.
- Additional Static Details: Beyond the aforementioned attributes, the CarInfoManager can provide a plethora of additional static information, such as the vehicle’s make, body type, engine specifications, and more.
Permissions and Security
To ensure the security and privacy of vehicle information, the CarInfoManager enforces a robust permission system. Access to static vehicle information is governed by the “PERMISSION_CAR_INFO” permission, granted at the “normal” level. This approach guarantees that only authorized applications can access critical data about the vehicle.
Code and Implementation
The core functionality of the CarInfoManager is encapsulated within the “CarInfoManager.java” file. This file resides in the “packages/services/Car/car-lib/src/android/car/” directory and contains the methods, structures, and logic necessary for retrieving and presenting static vehicle information to applications.
Diving Deeper: Exploring CAR_UX_RESTRICTION_SERVICE Car Manager Interfaces
Let’s know more about CAR_UX_RESTRICTION_SERVICE interface, exploring its role, capabilities, and underlying mechanisms.
Understanding the CAR_UX_RESTRICTION_SERVICE
The CAR_UX_RESTRICTION_SERVICE
, represented by the CarUxRestrictionsManager
, is an integral part of the Android Automotive ecosystem. Its primary function is to provide a mechanism for assessing and communicating the level of distraction optimization required for the driving experience. Distraction optimization involves tailoring the in-car interactions to minimize distractions and cognitive load on the driver, thus enhancing safety.
Key Features and Functions:
- Distraction Optimization Indication: The
CarUxRestrictionsManager
utilizes information from theCarDrivingStateManager
to determine whether the driving conditions necessitate a higher level of distraction optimization. It then communicates this information to relevant components and applications. - Integration with CarDrivingStateManager: The
CarDrivingStateManager
provides crucial input to theCarUxRestrictionsManager
. By analyzing factors such as vehicle speed, driving mode, and other contextual cues, the manager determines the appropriate level of distraction optimization required. - Promoting Safe Driving Practices: The primary aim of the
CAR_UX_RESTRICTION_SERVICE
is to promote safe driving practices by limiting potentially distracting activities when the driving conditions warrant it. This can include restricting certain in-car interactions or presenting information in a way that minimizes cognitive load. - Enhancing Driver Focus: By dynamically adjusting the user experience based on the current driving context, the
CarUxRestrictionsManager
ensures that drivers can focus on the road while still accessing essential information and functionalities.
Implementation and Code: CarUxRestrictionsManager.java
The core functionality of the CarUxRestrictionsManager
is implemented in the CarUxRestrictionsManager.java
file. This file can be found in the following directory: packages/services/Car/car-lib/src/android/car/drivingstate/
. Within this file, you\’ll find the logic, methods, and data structures that facilitate the communication between the CarDrivingStateManager
and other relevant components.
Design Structure of CarService
The CarService plays a crucial role in the Android Car Data Framework, providing a structured and organized approach to accessing a range of car-specific services. Here we aim to dissect the architecture and design of the CarService, focusing on its implementation and the interaction of various components. We’ll use the CarProperty service as an example to illustrate the design pattern, recognizing that a similar approach is adopted for other CarServices within the CarImpl.
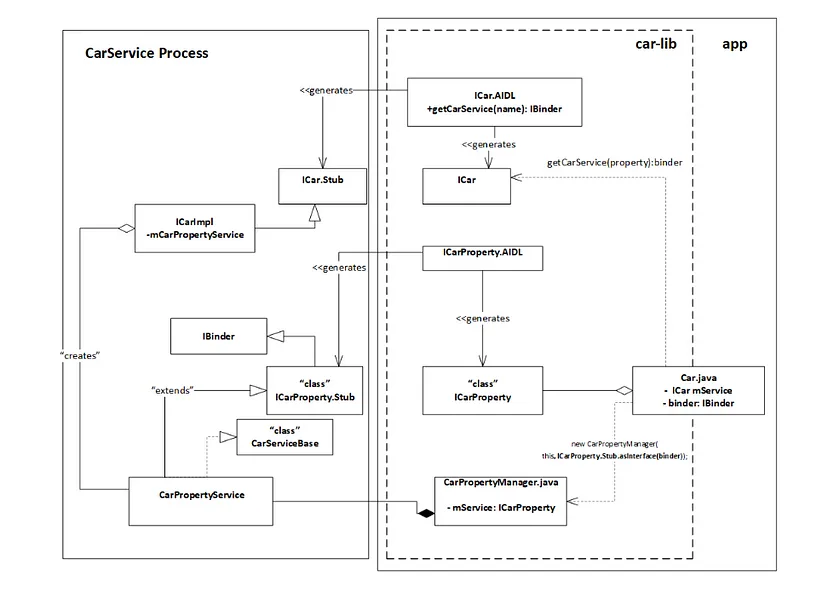
The car-lib makes use of the reference to the CarProperty Android service by calling the getCarServices(“property”) AIDL method, as provided by ICar. This very generic and simple method is implemented by the CarService in ICarImpl to return the specific service requested through the getCarService method, specified with the name of the service as its parameter. Thus, ICarImpl follows the Factory pattern implementation, which returns the IBinder object for the requested service. Within the car-lib, Car.Java will obtain the service reference by calling the specific client interface using ICarProperty.Stub.asInterface(binder). With the returned service reference, the CarPropertyManager will access the methods as implemented by the CarPropertyService. As a result, the car service framework-level service access is abstracted following this implementation pattern, and applications will include car-lib and utilize Car.Java to return respective Manager class objects.
Here is a short summary of the flow:
- Your application (
car-lib
) uses the Car service framework to access specific vehicle functionalities. - You request a specific service (e.g., CarProperty) using the
getCarService
method provided byICarImpl
. ICarImpl
returns a Binder object representing the requested service.- You convert this Binder object into an interface using
.asInterface(binder)
. - This interface allows your application to interact with the service (e.g., CarPropertyService) in a more abstract and user-friendly manner.
Understanding the pattern of classes and their relationships is important when adding new services under CarServices or making modifications to existing service implementations, such as extending CarMediaService to add new capabilities or updating CarNavigationServices to enhance navigation information data.
Car Properties and Permissions
Accessing car properties through the Android Car Data Framework provides developers with a wealth of vehicle-specific data, enhancing the capabilities of automotive applications. However, certain properties are protected by permissions, requiring careful consideration and interaction with user consent. Let’s jump into the concepts of car properties, permissions, and the nuanced landscape of access within the CarService framework.
Understanding Car Properties
Car properties encapsulate various aspects of vehicle data, ranging from basic information like the car’s VIN (Vehicle Identification Number) to more intricate details.
String vin = propertyManager.getProperty<String>(INFO_VIN, VEHICLE_AREA_TYPE_GLOBAL)?.value
All of the car properties are defined in the VehiclePropertyIds file. They can be read with CarPropertyManager. However, when trying to read the car VIN, a SecurityException is thrown. This means the app needs to request user permission to access this data.
Car Permissions
Just like a bouncer at a club, Android permissions control which apps can access specific services. This ensures that only the right apps get the keys to the digital kingdom. When it comes to the Car Service, permissions play a crucial role in determining which apps can tap into its features.
However, the Car Service is quite selective about who gets what. Here are a few permissions that 3rd party apps can ask for and possibly receive:
- CAR_INFO: Think of this as your car’s digital diary. Apps with this permission can access general information about your vehicle, like its make, model, and year.
- READ_CAR_DISPLAY_UNITS: This permission lets apps gather data about your car’s display units, such as screen size and resolution. It’s like letting apps know how big the stage is.
- CONTROL_CAR_DISPLAY_UNITS: With this permission, apps can actually tweak your car’s display settings. It’s like allowing them to adjust the stage lighting to set the perfect ambiance.
- CAR_ENERGY_PORTS: Apps with this permission can monitor the energy ports in your car, like charging points for electric vehicles. It’s like giving them the backstage pass to your car’s energy sources.
- CAR_EXTERIOR_ENVIRONMENT: This permission allows apps to access data about the external environment around your car, like temperature and weather conditions. It’s like giving them a sensor to feel the outside world.
- CAR_POWERTRAIN, CAR_SPEED, CAR_ENERGY: These permissions grant apps access to your car’s powertrain, speed, and energy consumption data. It’s like letting them peek under the hood and see how your car performs.
Now, here’s the twist: some permissions are VIP exclusive. They’re marked as “signature” or “privileged,” and only apps that are built by the original equipment manufacturer (OEM) and shipped with the platform can get them. These are like the golden tickets reserved for the chosen few — they unlock advanced features and deeper integrations with the Car Service.
Vehicle Property Permissions
In a vehicle system, properties are defined in a way that groups them as either SYSTEM or VENDOR properties. For example, as mentioned initially, consider the property VENDOR_FOO in the code snippet below. This property is assigned to the VENDOR group.
enum VehicleProperty: @2.0::VehicleProperty {
VENDOR_FOO = (
0xf100
| VehiclePropertyGroup:VENDOR
| VehiclePropertyType:STRING
| VehicleArea:GLOBAL
),
};
// types.hal
For properties in the VENDOR group, specific permissions are applied using the VENDOR_EXTENSION permissions, which can be of type Signature or System. This allows applications to access a special channel for vendor-specific information exchange.
<!-- Allows an application to access the vehicle vendor channel to exchange vendor-specific information. --> <!-- <p>Protection level: signature|privileged --> <permission android:name="android.car.permission.CAR_VENDOR_EXTENSION" android:protectionLevel="signature|privileged" android:label="@string/car_permission_label_vendor_extension" android:description="@string/car_permission_desc_vendor_extension" />
For properties not associated with the VENDOR group, permissions are set based on the property’s group. This is managed by adding the property to a permission group, as shown in the code snippet below.
/*
Permissions are defined in other files:
packages/services/Car/service/src/com/android/car/hal/PropertyHalServiceIds.java
Helper class to define which property IDs are used by PropertyHalService.
This class binds the read and write permissions to the property ID.
*/
mProps.put(VehicleProperty.INFO_VIN, new Pair<>(
Car.PERMISSION_IDENTIFICATION,
Car.PERMISSION_IDENTIFICATION));
mProps.put(VehicleProperty.INFO_MAKE, new Pair<>(
Car.PERMISSION_CAR_INFO,
Car.PERMISSION_CAR_INFO));
In simpler terms, permissions control access to different vehicle properties. To access the property INFO_VIN, you need the PERMISSION_IDENTIFICATION permission. Similarly, INFO_MAKE requires PERMISSION_CAR_INFO permission. There’s a clear connection between the application service layer (VehiclePropertyIds.java) and the HAL layer (PropertyHalServiceIds.java) for all properties.
Navigating Permissions
Permissions serve as a gatekeeper, regulating access to sensitive car property data. The association between properties and permissions is defined through multiple layers:
VehiclePropertyIds: This file links properties to specific permissions using comments like:
/**
* Door lock
* Requires permission: {@link Car#PERMISSION_CONTROL_CAR_DOORS}.
*/
public static final int DOOR_LOCK = 371198722;
Car.java: Permissions are defined as strings within Car.java
. For example:
/** * Permission necessary to control the car's door. * @hide */ @SystemApi public static final String PERMISSION_CONTROL_CAR_DOORS = "android.car.permission.CONTROL_CAR_DOORS";
car.service.AndroidManifest.xml: Permissions are declared in the AndroidManifest.xml file, specifying protection levels and attributes:
<permission android:name="android.car.permission.CONTROL_CAR_DOORS" android:protectionLevel="signature|privileged" android:label="@string/car_permission_label_control_car_doors" android:description="@string/car_permission_desc_control_car_doors" />
Permission Types
Three levels of permissions exist:
- Normal: Default permissions granted without user intervention.
- Dangerous: Permissions requiring user consent at runtime.
- Signature|Privileged: Restricted to system apps only.
Gaining Access to Signature|Privileged Properties
Properties protected by signature|privileged
permissions are accessible solely to system apps. To emulate this access for your application, consider these steps:
Build Keys: Sign your application with the same build keys as the system apps. This method effectively disguises your app as a system app, enabling access to signature|privileged
properties.
It’s essential to exercise caution when attempting to gain access to restricted properties, as this might lead to security risks and unintended consequences. Ensure that your intentions align with best practices and adhere to privacy and security principles.
ADB Commands for Car-Related Services
The Car System Service is a pivotal component within the Android Car Data Framework, providing a comprehensive platform for managing and interacting with various car-related services. Leveraging the power of Android Debug Bridge (ADB), developers gain the ability to access and manipulate car properties directly through the command line.
Accessing Car System Service via ADB
The Car System Service can be accessed through ADB commands, providing a direct line of communication to car-related services. The command structure follows the pattern:
adb shell dumpsys car_service <command> [options]
Let’s explore how this works in practice by querying a car property, specifically the door lock property:
adb shell dumpsys car_service get-property-value 16200B02 1
In the above command:
get-property-value
specifies the action to retrieve the value of a car property.16200B02
is the hex value corresponding to the door lock property (371198722 in decimal).1
indicates the vehicle area type, which could beVEHICLE_AREA_TYPE_GLOBAL
in this case.
For further insights into available commands and options, you can utilize the -h
flag:
adb shell dumpsys car_service -h
Car Apps
Here we will look into the diverse world of car apps, taking a closer look at their functionalities and the exciting possibilities they offer. We’ll explore a selection of these apps, each contributing to a seamless and immersive driving journey.
Diverse Range of Car Apps
Car apps form an integral part of the connected car ecosystem, enabling drivers and passengers to access a wide variety of features and services. These apps cater to different aspects of the driving experience, from entertainment and communication to navigation and vehicle control. Let’s explore some noteworthy examples of car apps:
- CarLauncher: Serving as the car’s home screen, CarLauncher provides an intuitive interface that allows users to access various apps and features seamlessly. It serves as the digital command center for interacting with different functionalities within the vehicle.
- CarHvacApp: This app takes control of the vehicle’s heating, ventilation, and air conditioning systems, ensuring optimal comfort for all occupants. Users can adjust temperature settings and airflow preferences to create a pleasant driving environment.
- CarRadioApp: CarRadioApp brings the traditional radio experience to the digital realm, allowing users to tune in to their favorite radio stations and enjoy a wide range of content while on the road.
- CarDialerApp: Designed specifically for in-car communication, CarDialerApp offers a safe and convenient way to make and receive calls while driving. Its user-friendly interface ensures that drivers can stay connected without compromising safety.
- CarMapsPlaceholder: While not specified in detail, CarMapsPlaceholder hints at the integration of navigation services, providing drivers with real-time directions and ensuring they reach their destinations efficiently.
- LocalMediaPlayer: This media player app allows users to enjoy their favorite music, podcasts, and audio content directly from their vehicle’s infotainment system, providing entertainment during their journeys.
- CarMessengerApp: Keeping drivers informed and connected, CarMessengerApp handles messages and notifications, ensuring that essential communications are accessible without distractions.
- CarSettings: CarSettings brings customization to the forefront, enabling users to tailor their driving experience by configuring various vehicle settings, preferences, and options.
- EmbeddedKitchenSinkApp: As its name suggests, EmbeddedKitchenSinkApp is a comprehensive demo app that showcases a wide range of features, serving as a platform for testing and experimentation.
Third-Party Apps
In the dynamic realm of Android Automotive, third-party apps have emerged as a significant avenue for innovation and enhanced driving experiences. However, these apps operate within a carefully orchestrated ecosystem, designed to ensure driver safety and minimize distractions. Here, we delve into the intricate landscape of third-party apps for Android Automotive, exploring their access restrictions, design considerations, and the pivotal role they play in enhancing driver safety.
Access Restrictions and the Play Store for Auto
Unlike traditional Android apps, third-party apps for Android Automotive do not have direct access to the system APIs. This approach is a deliberate design choice aimed at maintaining system stability and safeguarding against potential security vulnerabilities. Apps available on the Play Store for Android Automotive OS and Android Auto undergo thorough scrutiny to ensure compliance with stringent design requirements. This ensures that apps meet specific standards of quality, functionality, and safety before being listed for users.
Minimizing Driver Distraction: A Core Principle
Driver distraction is a paramount concern in the development of third-party apps for Android Automotive. Given the potential risks associated with diverting a driver’s attention from the road, Google places significant emphasis on creating a distraction-free environment. Apps must adhere to strict guidelines to minimize any potential interference with the driver’s focus.
Key principles for minimizing driver distraction include:
- Design Consistency: Apps must follow consistent design patterns that prioritize clarity and ease of use. Intuitive navigation and minimalistic interfaces ensure that users can interact with the app without confusion.
- Voice Interaction: Voice commands are a pivotal aspect of reducing distraction. Apps should integrate voice-based interactions to allow drivers to perform tasks without taking their hands off the wheel.
- Limited Visual Engagement: Apps should limit the frequency and complexity of visual interactions. Displaying large amounts of information or requiring frequent glances can divert the driver’s attention from the road.
- Contextual Relevance: App content and notifications should be contextually relevant to the driving experience. This ensures that only essential and non-distracting information is presented.
- Appropriate Testing and Evaluation: Developers are encouraged to rigorously test and evaluate their apps in simulated driving scenarios to identify potential distractions and address them before deployment.
Supported App Categories: Revolutionizing the Drive
Third-party apps for Android Automotive have expanded the possibilities of in-car technology, offering users diverse functionalities that seamlessly integrate into the driving experience. The following app categories are supported, each adding a layer of convenience and engagement to the road:
- Media (Audio) Apps: These apps turn vehicles into personalized entertainment hubs, allowing users to enjoy their favorite music, podcasts, and audio content while on the go. The integration of media apps ensures a dynamic and enjoyable driving experience.
- Messaging Apps: Messaging apps take communication to the next level by using text-to-speech and voice input technologies. Drivers can stay connected and informed through voice-enabled interactions, minimizing distraction and enhancing safety.
- Navigation, Parking, and Charging Apps: These apps provide valuable support for drivers. Navigation apps offer real-time directions, while parking apps help locate available parking spaces. Charging apps aid electric vehicle drivers in finding charging stations, adding a layer of convenience to sustainable travel.
Impact on the Driving Experience
Third-party apps wield the power to reshape the driving experience, infusing it with innovation and convenience. Media apps transform mundane journeys into immersive musical experiences, while messaging apps ensure that communication remains seamless and hands-free. Navigation, parking, and charging apps not only guide drivers efficiently but also contribute to a greener and more sustainable travel ecosystem.
Guidelines for Quality and Safety
Google places paramount importance on quality and safety when it comes to third-party apps for Android Automotive. Google has provided a set of references and guidelines for developers:
- Getting Started with Car Apps: https://developer.android.com/training/cars/start
- Quality Guidelines for Car Apps: https://developer.android.com/docs/quality-guidelines/car-app-quality
- Navigation Guidelines for Car Apps: https://developer.android.com/training/cars/navigation
Developers are encouraged to adhere to the quality guidelines outlined in the Android documentation. These guidelines ensure that apps are user-friendly, visually consistent, and minimize driver distraction.
Developing for Android Automotive
The realm of Android development has extended its reach beyond smartphones and tablets, embracing the automotive landscape with open arms. Developers now have the opportunity to create apps that enhance the driving experience, making vehicles smarter, safer, and more connected. In this context, let’s delve into exploring the tools, requirements, and considerations that drive this exciting endeavor.
Android Studio: The Gateway to Automotive Development
For developers venturing into the world of Android Automotive, Android Studio serves as an indispensable companion. This development environment provides dedicated Software Development Kits (SDKs) for Android versions R/11 and beyond, empowering developers to craft innovative applications tailored to vehicles’ unique needs.
Key highlights of developing for Android Automotive include:
- SDK Availability: Android Studio offers automotive SDKs for Android versions R/11, S/12, and T/13. These SDKs extend their capabilities to the automotive domain, providing developers with the tools and resources they need to create engaging and functional automotive apps.
- Minimum Android Studio Version: To develop automotive apps, developers need Android Studio version 4.2 or higher. This version includes the necessary tools and resources for automotive development, such as the Automotive Gradle plugin and the Automotive SDK.
- Transition to Stability: Android Studio version 4.2 transitioned to a stable release in May 2021. This means that it is the recommended version for automotive development. However, developers can also use the latest preview versions of Android Studio, which include even more features and improvements for automotive development.
Automotive AVD for Android Automotive Car Service Development
The Automotive AVD (Android Virtual Device) provides developers with a platform to emulate Android Automotive systems, facilitating the refinement of apps and services before deployment to physical vehicles. Let’s explore the key components and aspects of the Automotive AVD.
SDK and System Image
The Automotive AVD operates within the Android 10.0 (Q) (latest T/13) software development kit (SDK). This SDK version is specifically tailored to the needs of Android Automotive Car Service. The AVD utilizes the “Automotive with Google Play Intel x86 Atom” system image, replicating the architecture and features of an Android Automotive environment on Intel x86-based hardware.
AVD Configuration
The AVD configuration is structured around the “Automotive (1024p landscape) API 29” and in the latest “Automotive (1024p landscape) API 32” setup. This configuration mimics a landscape-oriented 1024p (pixels) display, which is representative of the infotainment system commonly found in vehicles. This choice of resolution and orientation ensures that developers can accurately assess how their apps will appear and function within the context of an automotive display.
Additional Features
The Automotive AVD also includes a number of additional features that can be helpful for developers, such as:
- Support for multiple displays: The Automotive AVD can be configured to support multiple displays, which is useful for developing apps that will be used in vehicles with large infotainment systems.
- Support for sensors: The Automotive AVD can be configured to simulate a variety of sensors, such as the accelerometer, gyroscope, and magnetometer. This allows developers to test how their apps will behave in response to changes in the environment.
- Support for connectivity: The Automotive AVD can be configured to connect to a variety of networks, such as Wi-Fi, cellular, and Bluetooth. This allows developers to test how their apps will behave when connected to the internet or other devices.
Testing and Experimentation
Developers can utilize the Automotive AVD for a range of purposes:
- App Development: The AVD allows developers to test how their apps interact with the Android Automotive Car Service interface, ensuring compatibility and optimal performance.
- User Experience: User interface elements, such as touch controls and voice interactions, can be evaluated in a simulated automotive environment.
- Feature Integration: Developers can experiment with integrating their apps with Android Automotive Car Service features like navigation, voice commands, and media playback.
Advantages of Automotive AVD
- Cost-Efficient: The Automotive AVD eliminates the need for dedicated physical hardware for testing, reducing costs and resource requirements.
- Efficiency: Developers can rapidly iterate and debug apps within a controlled virtual environment.
- Realistic Testing: The AVD closely emulates the behavior and constraints of an actual Android Automotive system, providing a realistic testing environment.
- Customization: AVD configurations can be fine-tuned to match specific hardware and software requirements.
Embracing the Future: Considerations for Automotive Development
Developing for Android Automotive requires a strategic approach that takes into account the unique context of the driving environment. While the tools and SDKs provide a solid foundation, developers must also consider:
- Driver Safety: Safety is paramount in the automotive domain. Apps should be designed with minimal driver distraction in mind, favoring voice interactions and intuitive interfaces that prioritize safe driving.
- Contextual Relevance: The driving experience is distinct from other contexts. Apps should deliver information and services that are relevant to the road, such as navigation guidance, vehicle status, and communication functionalities.
- User-Centric Design: User experience is key. Design apps that align with drivers’ needs, making interactions seamless and intuitive even in a dynamic and ever-changing driving environment.
Conclusion
Android Automotive represents a transformative leap in the automotive industry, seamlessly integrating technology into vehicles. The Car Service and Car Manager components facilitate communication between applications and the vehicle’s hardware, enhancing the user experience. As developers, exploring this ecosystem opens doors to innovative in-car applications while adhering to strict guidelines to ensure driver safety. With Android Automotive’s rapid advancement, the future promises even more exciting opportunities for both developers and car enthusiasts alike.