When it comes to design patterns, some are pretty straightforward, while others might seem a bit more complicated at first glance. One such pattern is the Interpreter design pattern. While it may sound like something you’d use only in very specific contexts, the Interpreter pattern can actually be quite handy when you’re dealing with languages or grammars, like when building parsers or evaluators. Today, I’ll walk you through the Interpreter pattern in a simple and approachable way, using Kotlin.
So, what exactly is the Interpreter design pattern? Let’s dive in!
What is the Interpreter Design Pattern?
The Interpreter design pattern is used to define a representation for a grammar of a language and provide an interpreter that uses the representation to interpret sentences in the language. In simpler terms, it’s a way to evaluate statements or expressions based on a predefined set of rules or grammar.
It’s particularly useful when you need to evaluate strings that follow a specific format, like mathematical expressions, SQL queries, or even programming languages.
Structure of Iterpreter Design Pattern
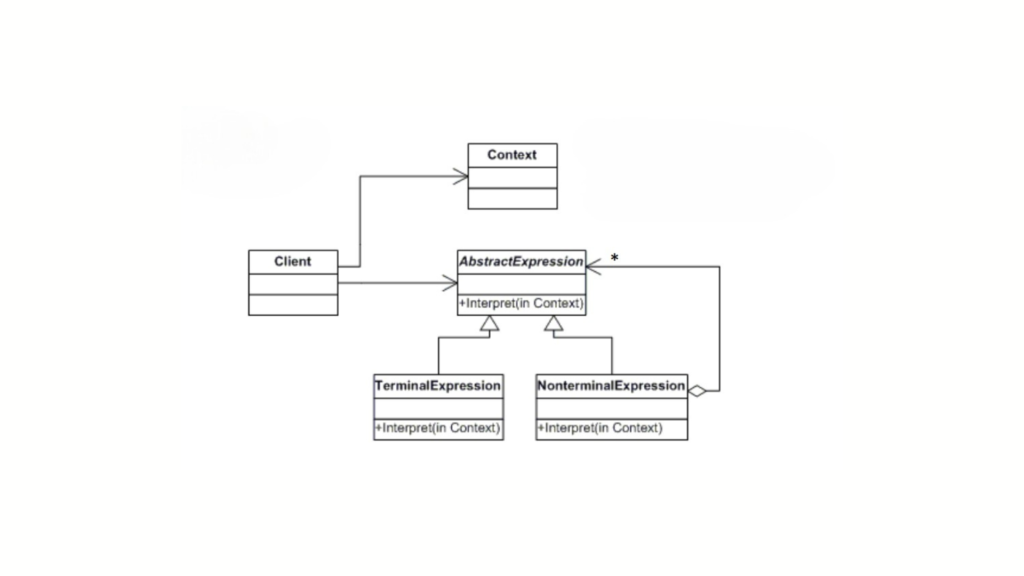
The main components of the Interpreter pattern:
- AbstractExpression: This defines the interface for all expressions. It usually has an
interpret()
method, which is responsible for interpreting the expression. - TerminalExpression: These are the basic expressions in the grammar. They usually don’t have any sub-expressions. For example, in a mathematical expression, a number or a variable would be a terminal expression.
- NonTerminalExpression: These expressions are made up of one or more terminal or non-terminal expressions. For example, an addition or subtraction operator in a mathematical expression.
- Context: This holds the data or the input we want to interpret.
When Should We Use It?
The Interpreter pattern comes in handy when:
- We need to evaluate a series of expressions that follow some grammar or rules.
- We’re dealing with complex expressions that can be broken down into smaller components.
- The language we’re working with is relatively simple but needs a structured approach.
Now that we know what the pattern is and when to use it, let’s look at how we can implement it in Kotlin.
Wait… Have you ever wanted to create a calculator for math expressions like 3 + 5 - 2
? Or a command parser for a small scripting language? That’s the perfect use case!
Simple Math Expression Interpreter
We’re going to interpret a basic math expression like 3 + (5 - 2)
. Here’s how we’ll do it step by step.
Define the Abstract Expression
We’ll start by defining our abstract expression interface, which will be used by both terminal and non-terminal expressions.
// AbstractExpression interface
interface Expression {
fun interpret(context: Map<String, Int>): Int
}
Here, the interpret
method takes a context
(which can be a map of variable values) and returns an integer result.
Create Terminal Expressions
Now, let’s create terminal expressions. These are the base expressions, like numbers in the expression.
// TerminalExpression class for numbers
class NumberExpression(private val number: Int) : Expression {
override fun interpret(context: Map<String, Int>): Int {
return number
}
}
In this class, we simply store a number, and when we interpret it, we return that number.
Create Non-Terminal Expressions
Next, we’ll implement the non-terminal expressions. These are the operators like addition or subtraction. Each non-terminal expression will hold references to two sub-expressions: the left-hand side and the right-hand side.
// NonTerminalExpression class for addition
class AddExpression(private val left: Expression, private val right: Expression) : Expression {
override fun interpret(context: Map<String, Int>): Int {
return left.interpret(context) + right.interpret(context)
}
}
// NonTerminalExpression class for subtraction
class SubtractExpression(private val left: Expression, private val right: Expression) : Expression {
override fun interpret(context: Map<String, Int>): Int {
return left.interpret(context) - right.interpret(context)
}
}
Here, the AddExpression
and SubtractExpression
are the operators, and they each hold two Expression
objects, representing the left and right operands. When we interpret them, we recursively interpret both sides and then apply the operation. Basically each of these expressions takes two sub-expressions (left and right) and performs an operation on their results.
Build the Expression Tree (Bringing All Together)
Now that we’ve created our expressions, we can evaluate them as a tree, where each node represents an operation and the leaves are the numbers. Let’s explore how these components come together in a simple interpreter.
fun main() {
// Build the expression tree
val expression = AddExpression(
NumberExpression(3),
SubtractExpression(NumberExpression(5), NumberExpression(2))
)
// Create a context if needed (in this case, we don't need it, so we use an empty map)
val result = expression.interpret(emptyMap())
// Print the result
println("Result: $result") // Output will be 3 + (5 - 2) = 6
}
Here,
Expression Tree Construction: To begin, we construct an expression tree. At the root, we have an AddExpression
, which consists of two child nodes:
- The left child is a
NumberExpression(3)
. - The right child is a
SubtractExpression
, which further has two children:NumberExpression(5)
andNumberExpression(2)
.
Interpretation: When the interpret()
method is called on the root node (AddExpression
), it processes its children recursively. The AddExpression
calculates the sum of its left and right sub-expressions. The right sub-expression (SubtractExpression
) computes the result of 5 - 2
. Finally, the root evaluates 3 + 3
, resulting in the value 6
.
Context: In this example, no external variables are required, so we use an empty map as the context. But what if we want to handle variables like x + y
, where the values of x
and y
are defined at runtime? In that case, we would use a context like this:
// Context: x = 3, y = 5
val context = mapOf("x" to 3, "y" to 5)
Advantages of Using the Interpreter Pattern
- Extensibility: It’s easy to add more expressions or operators without affecting the existing code. For example, if we wanted to add multiplication or division, we could simply create new
NonTerminalExpression
classes for those operations. - Maintainability: The expression logic is separated into individual components, making the code cleaner and easier to maintain.
- Readability: With the use of well-named classes (like
AddExpression
,NumberExpression
), the code becomes more understandable and easier to extend.
Disadvantages
- Complexity: For simple scenarios, the Interpreter pattern might introduce unnecessary complexity. If the problem doesn’t require a structured approach, a simpler solution might be more appropriate.
- Performance: In cases with large and complex expression trees, the recursive nature of the Interpreter pattern could lead to performance issues. It might not be the best choice for very large grammars.
Conclusion
The Interpreter design pattern is like building a Lego set for a specific problem—it lets you piece together small, reusable blocks (expressions) into a complete solution. While it might not be the go-to pattern for every scenario, it’s a powerful tool when you need to evaluate structured rules or languages.
In Kotlin, this pattern feels natural thanks to its object-oriented features and functional programming capabilities. So next time you’re dealing with something like custom DSLs, math interpreters, or even mini-scripting engines, give the Interpreter pattern a try!
Until next time, happy coding! 😊