Object creation and constructors are fundamental concepts in Java programming. Understanding these concepts is crucial for writing efficient and maintainable code. Additionally, the singleton design pattern, which restricts the instantiation of a class to a single object, plays a vital role in various scenarios. This blog delves into these three key areas, providing a comprehensive guide for Java developers.
Object Creation in Java
In Java, objects are instances of classes, which act as blueprints defining the structure and behavior of the objects they create. The process of creating an object involves allocating memory and initializing its attributes. Let’s explore how object creation is done in Java:
// Sample class definition
public class MyClass {
// Class variables or attributes
private int myAttribute;
// Constructor
public MyClass(int initialValue) {
this.myAttribute = initialValue;
}
// Methods
public void doSomething() {
System.out.println("Doing something with myAttribute: " + myAttribute);
}
}
// Object creation
MyClass myObject = new MyClass(42);
myObject.doSomething();
In the example above, we define a class MyClass
with a private attribute myAttribute
, a constructor that initializes this attribute, and a method doSomething
that prints the attribute value. The object myObject
is then created using the new
keyword, invoking the constructor with an initial value of 42.
Total 5 ways we create new objects in java
Moving beyond the basics of how object created, let’s explore the five distinct methods to create new objects in Java.
- Using the ‘new’ Keyword: The most common and straightforward method involves the use of the ‘new’ keyword. This keyword, followed by the constructor, allocates memory for a new object.
- Utilizing ‘newInstance()’ Method: Another approach is the use of the ‘newInstance()’ method. This method is particularly useful when dealing with classes dynamically, as it allows the creation of objects without explicitly invoking the constructor.
- Leveraging Factory Methods: Factory methods offer a design pattern where object creation is delegated to factory classes. This approach enhances flexibility and encapsulation, providing a cleaner way to create objects.
- Employing Clone Methods: Java supports the cloning mechanism through the ‘clone()’ method. This method creates a new object with the same attributes as the original, offering an alternative way to generate objects.
- Object Creation via Deserialization: Deserialization involves reconstructing an object from its serialized form. By employing deserialization, objects can be created based on the data stored during serialization.
Constructors in Java
Constructors are special methods within a class responsible for initializing the object’s state when it is created. They have the same name as the class and are invoked using the new
keyword during object creation. It’s important to note that both the instance block and the constructor serve distinct functions. The instance block is utilized for activities beyond initialization, such as counting the number of created objects.
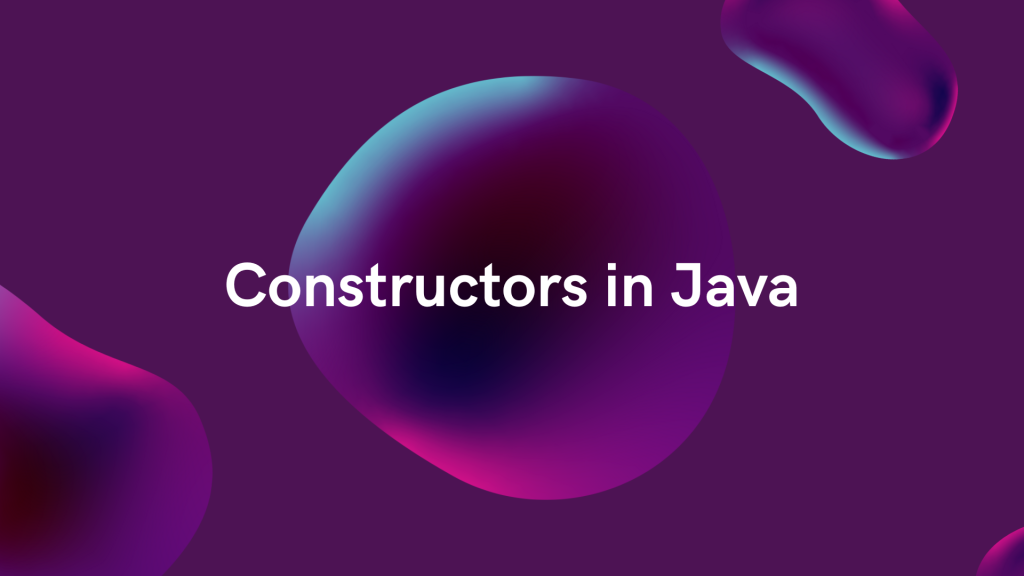
Rules for writing constructors:
- The name of the class and the name of the constructor must be the same.
- The concept of return type is not applicable to constructors, including void. If, by mistake, void is used with the class name as a constructor, it won’t generate a compiler error because the compiler treats it as a method.
- The only applicable modifiers for constructors are public, private, protected, and default. Other types are not allowed.
- Only the compiler will generate a default constructor, not the JVM. If you do not write any constructor, it will be created automatically.
Default Constructor Prototype:
- It is always a no-argument constructor.
- The access modifier is exactly the same as the class; only consider ‘public’ as applicable, and others are not allowed.
- It contains only one line, i.e., ‘super()’. This is a no-argument call to the super constructor, but this rule is applicable only to ‘public’ and ‘default’.
- The first line is always ‘this()’ or ‘super()’. If you don’t write anything, the compiler places ‘super()’ in the default constructor.
- Within the constructor, we can use ‘super()’ or ‘this()’, but not simultaneously, and they cannot be used outside the constructor.
- We can call a constructor directly from another constructor only.
Understanding Programmers’ Code and Compiler-Generated Code for Constructors
Programmers write constructors to define how objects of a class should be instantiated and initialized. However, compilers also have a role in generating default constructors when programmers don’t explicitly provide them. Let’s explore it in detail.
Programmers’ Code for Constructors
Purpose of Constructors: Constructors are special methods within a class that are called when an object is created. They initialize the object’s state and set it up for use. Programmers design constructors to meet specific requirements of their classes.
Syntax and Naming Conventions: Programmers follow certain syntax rules and naming conventions when writing constructors. The constructor’s name must match the class name, and it can take parameters to facilitate customizable initialization.
public class MyClass {
// Programmers' code for constructor
public MyClass(int parameter) {
// Initialization logic here
}
}
Custom Initialization Logic: Programmers have the flexibility to include custom initialization logic within constructors. This logic can involve setting default values, validating input parameters, or performing any necessary setup for the object.
Overloading Constructors: Programmers can overload constructors by providing multiple versions with different parameter lists. This allows for versatility when creating objects with various configurations.
public class MyClass {
// Parameterized constructor
public MyClass(int parameter) {
// Initialization logic
}
// Default constructor (no parameters)
public MyClass() {
// Default initialization logic
}
}
Compiler-Generated Code for Constructors:
Default Constructors: If a programmer doesn’t explicitly provide a constructor, the compiler steps in and generates a default constructor. This default constructor is a no-argument constructor that initializes the object with default values.
public class MyClass {
// Compiler-generated default constructor
public MyClass() {
// Default initialization logic by the compiler
}
}
Super Constructor Call: In the absence of explicit constructor calls by the programmer, the compiler inserts a call to the superclass constructor (via super()
) as the first line of the constructor. This ensures proper initialization of the inherited components.
No-Argument Initialization: Compiler-generated default constructors are often no-argument constructors that perform basic initialization. However, this initialization might not suit the specific needs of the class, which is why programmers often provide their own constructors.
Compiler Warnings: While the compiler-generated default constructor is helpful, it may generate warnings if the class contains fields that are not explicitly initialized. Programmers can suppress these warnings by providing their own constructors with proper initialization.
Understanding super() and this() in Constructors
In the realm of object-oriented programming, the keywords super()
and this()
play a crucial role when it comes to invoking constructors. These expressions are used to call the constructor of the superclass (super()
) or the current class (this()
). Let’s explore the nuances of using super()
and this()
in constructors.
Purpose of super() and this():
- super(): This keyword is used to invoke the constructor of the superclass. It allows the subclass to utilize the constructor of its superclass, ensuring proper initialization of inherited members.
- this(): This keyword is employed to call the constructor of the current class. It is useful for scenarios where a class has multiple constructors, and one constructor wants to invoke another to avoid redundant code.
Usage Constraints:
Only in Constructor at First Line: Both super()
and this()
can be used only within the constructor, and they must appear as the first line of code within that constructor. This restriction ensures that necessary initialization steps are taken before any other logic in the constructor is executed.
public class ExampleClass extends SuperClass {
// Constructor using super()
public ExampleClass() {
super(); // Constructor call to superclass
// Other initialization logic for the current class
}
// Constructor using this()
public ExampleClass(int parameter) {
this(); // Constructor call to another constructor in the same class
// Additional logic based on the parameter
}
}
Limited to Once in Constructor: Both super()
and this()
can be used only once in a constructor. This limitation ensures that constructor calls are clear and do not lead to ambiguity or circular dependencies.
public class ExampleClass extends SuperClass {
// Correct usage
public ExampleClass() {
super(); // Can be used once
this(); // Can be used once
}
// Incorrect usage - leads to compilation error
public ExampleClass(int parameter) {
super();
this(); // Compilation error: Constructor call can only be used once
}
}
Understanding ‘super
‘ and ‘this'
Keywords in Java
Same like super() and this(), the keywords super
and this
are essential for referencing instance members of the superclass and the current class, respectively. Let’s explore the characteristics and usage of super
and this
:
Purpose of super
and this
:
super
: This keyword is used to refer to the instance members (fields or methods) of the superclass. It is particularly useful in scenarios where the subclass has overridden a method, and you want to call the superclass version.this
: This keyword is employed to refer to the instance members of the current class. It is beneficial when there is a need to disambiguate between instance variables of the class and parameters passed to a method or a constructor.
Usage Constraints:
Anywhere Except Static Context: Both super
and this
can be used anywhere within non-static methods, constructors, or instance blocks. However, they cannot be used directly in a static context, such as in a static method or a static block. Attempting to use super
or this
in a static context will result in a compilation error.
public class ExampleClass {
int instanceVariable;
// Non-static method
public void exampleMethod() {
int localVar = this.instanceVariable; // Using 'this' to reference instance variable
// Additional logic
}
// Static method - Compilation error
public static void staticMethod() {
int localVar = this.instanceVariable; // CE: Cannot use 'this' in a static context
// Additional logic
}
}
Multiple Usages: Both super
and this
can be used any number of times within methods, constructors, or instance blocks. This flexibility allows developers to reference the appropriate instance members as needed.
public class ExampleClass extends SuperClass {
int subclassVariable;
// Method using 'super' and 'this'
public void exampleMethod() {
int localVar1 = super.methodInSuperclass(); // Using 'super' to call a method from the superclass
int localVar2 = this.subclassVariable; // Using 'this' to reference a subclass instance variable
// Additional logic
}
}
Understanding Overloaded Constructors in Java
In Java programming, an overloaded constructor refers to the practice of defining multiple constructors within a class, each with a different set of arguments. This mirrors the concept of method overloading, where automatic promotion occurs. Let’s delve into the characteristics of overloaded constructors and some important considerations:
Overloaded Constructor Concept:
- Definition: Overloaded constructors are multiple constructors within a class, distinguished by differences in their argument lists. This enables flexibility when creating objects, accommodating various initialization scenarios.
- Automatic Promotion: Similar to method overloading, automatic promotion of arguments happens in overloaded constructors. Java automatically converts smaller data types to larger ones to match the constructor signature.
Inheritance and Overriding Constraints:
Not Applicable to Constructors: Inheritance and method overriding concepts do not apply to constructors. Each class, including abstract classes, can have constructors. However, interfaces, which consist of static variables, do not contain constructors.
Recursive Constructor Invocation:
Stack Overflow Exception: Unlike method recursion where a stack overflow exception occurs after execution, recursive constructor invocation leads to a compile-time error. It’s crucial to handle recursive constructor calls carefully to prevent code execution issues.
No-Argument Constructor Recommendation:
Avoiding Issues: When writing an argument constructor in a parent class, it is highly recommended to include a no-argument constructor. This is because the child class constructor automatically adds a super()
call, which can create problems if a no-argument constructor is not present in the parent class.
public class ParentClass {
// Argument constructor
public ParentClass(int parameter) {
// Constructor logic
}
// Recommended no-argument constructor
public ParentClass() {
// No-argument constructor logic
}
}
Exception Handling in Constructors:
Checked Exception Propagation: If a parent class constructor throws a checked exception, the child class constructor must compulsorily throw the same checked exception or its parent exception. This ensures proper exception handling across the class hierarchy.
public class ParentClass {
// Constructor with checked exception
public ParentClass() throws SomeCheckedException {
// Constructor logic
}
}
public class ChildClass extends ParentClass {
// Child class constructor must propagate the same or a parent checked exception
public ChildClass() throws SomeCheckedException {
super(); // Call to the parent constructor
// Additional constructor logic
}
}
Understanding the principles of overloaded constructors in Java is essential for creating flexible and robust class structures. Adhering to best practices, such as including a no-argument constructor and handling exceptions consistently, ensures smooth execution and maintainability of code within the context of constructors.
Understanding Singleton Design Pattern in Java
In Java, the Singleton pattern is a design pattern that ensures a class has only one instance and provides a global point to this instance. It is often employed in scenarios where having a single instance of a class is beneficial, such as in the case of Runtime
, BusinessDelegate
, or ServiceLocator
. Let’s explore the characteristics and advantages of Singleton classes in Java:
Singleton Class Concept:
Single Private Constructor: The key feature of a Singleton class is the presence of a single private constructor. This constructor restricts the instantiation of the class from external sources, ensuring that only one instance can be created.
public class SingletonClass {
private static final SingletonClass instance = new SingletonClass();
// Private constructor
private SingletonClass() {
// Constructor logic
}
// Access method to get the single instance
public static SingletonClass getInstance() {
return instance;
}
}
Singleton Instances:
Usage Scenario:
// Utilizing the Singleton instance across the application
Runtime r1 = Runtime.getRuntime();
Runtime r2 = Runtime.getRuntime();
// ...
Runtime r100000 = Runtime.getRuntime(); // Up to 100,000 or more requests use the same object
Advantages of Singleton Class:
- Performance Improvement: Singleton classes offer performance benefits by providing a single instance shared among multiple clients. This avoids the overhead of creating and managing multiple instances.
- Global Access: The Singleton pattern provides a global point of access to the single instance. This ensures that any part of the application can easily access and utilize the shared object.
Singleton Design Pattern: Two Approaches
In Java, the Singleton design pattern ensures that a class has only one instance and provides a global point of access to that instance. Two common approaches involve using one private constructor, one private static variable, and one public factory method. The Runtime
class is a notable example implementing this pattern. Let’s explore both approaches:
Approach 1: Eager Initialization
In this approach, the singleton instance is created eagerly during class loading. The private constructor ensures that the class cannot be instantiated from external sources, and the public factory method provides access to the single instance.
public class Test {
Approach 2: Lazy Initialization with Double-Checked Locking
This approach initializes the singleton instance lazily, creating it only when needed. The getTest method checks if the instance is null before creating it. Double-checked locking ensures thread safety in a multithreaded environment. // Eagerly initialized static variable
private static Test t = new Test();
// Private constructor
private Test() {
// Constructor logic
}
// Public factory method
public static Test getTest() {
return t;
}
}
Approach 2: Lazy Initialization with Double-Checked Locking
This approach initializes the singleton instance lazily, creating it only when needed. The getTest
method checks if the instance is null
before creating it. Double-checked locking ensures thread safety in a multithreaded environment.
public class Test {
// Lazily initialized static variable
private static Test t = null;
// Private constructor
private Test() {
// Constructor logic
}
// Public factory method with double-checked locking
public static Test getTest() {
if (t == null) {
synchronized (Test.class) {
if (t == null) {
t = new Test();
}
}
}
return t;
}
}
Usage of Singleton Instances:
Instances of the Test
class are obtained through the getTest
method, ensuring that there is only one instance throughout the application.
// Using Singleton instances
Test instance1 = Test.getTest();
Test instance2 = Test.getTest();
// Both instances refer to the same object
System.out.println(instance1 == instance2); // Output: true
Restricting Child Class Creation in Java
In Java, final classes inherently prevent inheritance, making it impossible to create child classes. However, if a class is not declared as final, but there is a desire to prevent the creation of child classes, one effective method is to use a private constructor and declare all constructors in the class as private. This approach restricts the instantiation of both the superclass and any potential subclasses. Let’s explore this concept:
public class Parent {
// Private constructor to prevent external instantiation
private Parent() {
// Constructor logic
}
}
In this scenario, attempting to create a child class that extends Parent
would be problematic due to the private constructor:
public class Child extends Parent {
// Compiler error: Implicit super constructor Parent() is not visible for default constructor.
public Child() {
super(); // Attempting to access the private constructor of the superclass
}
}
Explanation:
- Private Constructor in Parent Class: The
Parent
class has a private constructor, making it inaccessible from outside the class. This means that even if a child class attempts to callsuper()
, it cannot access the private constructor of the parent class. - Child Class Compilation Error: In the
Child
class, attempting to create a constructor that callssuper()
results in a compilation error. This is because the private constructor in theParent
class is not visible to theChild
class.
Usage of Private Constructor:
The private constructor ensures that instances of the Parent
class cannot be created externally. Therefore, it prevents not only the creation of child classes but also the instantiation of the parent class from outside the class itself.
By utilizing a private constructor in a class and declaring all constructors as private, it is possible to restrict the creation of both child classes and instances of the class from external sources. This approach adds an additional layer of control over class instantiation and inheritance in Java.
Conclusion
Understanding object creation, constructors, and the singleton design pattern is essential for writing robust and efficient Java code. These concepts enable you to create objects, initialize them properly, and control their lifecycle. By effectively utilizing these tools, you can enhance the maintainability and performance of your Java applications.