Ever notice how every app we use—from Amazon to our banking apps—makes everything seem so effortless? What we see on the surface is just the tip of the iceberg. Beneath that sleek interface lies a mountain of complex code working tirelessly to ensure everything runs smoothly. This is where the Facade Design Pattern shines, providing a way to hide all those intricate details and offering us a straightforward way to interact with complex systems.
So, what exactly is a facade? Think of it as a smooth layer that conceals the complicated stuff, allowing us to focus on what truly matters. In coding, this pattern lets us wrap multiple components or classes into one easy-to-use interface, making our interactions clean and simple. And if you’re using Kotlin, implementing this pattern is a breeze—Kotlin’s modern syntax and interfaces make creating facades feel effortless.
You might be wondering, “Isn’t this just like data hiding in OOP?” Not quite! Facades are more about simplifying access to complex systems rather than merely keeping details private. So, let’s dive in, explore what makes the Facade Pattern so powerful, look at real-life examples, and see the ups and downs of using it in Kotlin. Let’s get started!
Facade Design Pattern
The Facade pattern is part of the structural design patterns in the well-known Gang of Four (GoF) design patterns. This pattern provides a simplified interface to a complex subsystem, which may involve multiple classes and interactions. The primary goal of the Facade pattern is to reduce the complexity by creating a single entry point that manages complex logic behind the scenes, allowing the client (user of the code) to interact with a simplified interface.
In simple words, instead of directly interacting with multiple classes, methods, or modules within a complex subsystem, a client can work with a single Facade class that handles the complexities.
Imagine you’re trying to use a complex appliance with lots of buttons and settings. Instead of figuring out how to navigate all those features, you just have a single, easy-to-use control panel that manages everything behind the scenes. That’s exactly what the Facade pattern does.
It creates a straightforward interface that acts as a single entry point to a complex subsystem. This way, you don’t have to deal with multiple classes or methods directly; you can just interact with the Facade class, which takes care of all the complexity for you. It’s all about making things easier and less overwhelming!
I always believe that to truly use or understand any design pattern, it’s essential to grasp its structure first. Once we have a solid understanding of how it works, we can apply it to our everyday coding. So, let’s take a look at the structure of facade pattern first, and then we can dive into the coding part together.
Structure of the Facade Design Pattern
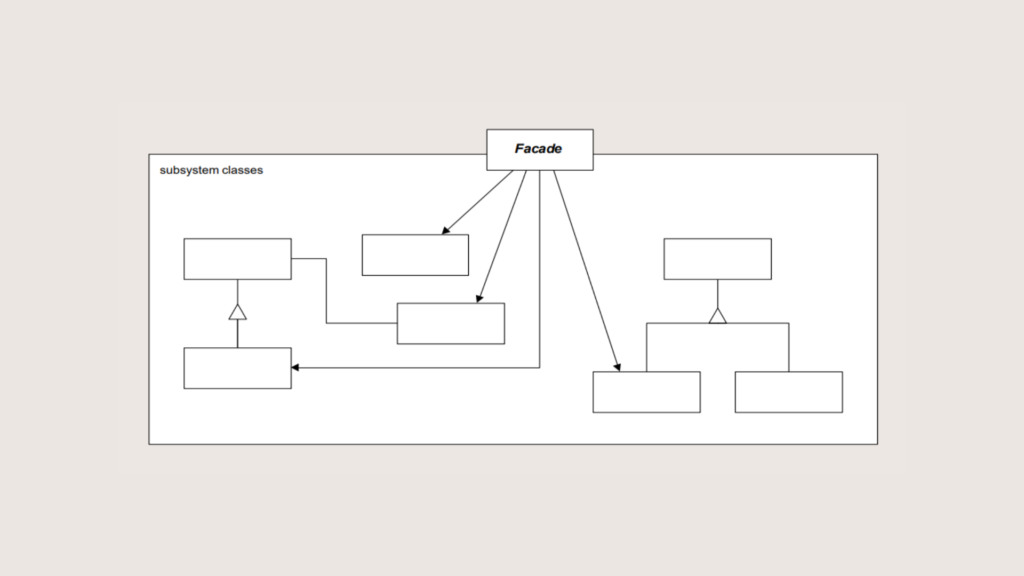
In the Facade Pattern, we have:
- Subsystem classes that handle specific, granular tasks.
- A Facade class that provides a simplified interface to these subsystems, delegating requests to the appropriate classes.
Let’s see how this looks in Kotlin.
Simple Scenario
Think about our office coffee maker for a second. When we want to brew our favorite blend, we often have to click multiple buttons on the control panel. Let’s see how we can make coffee with a single click using the Facade pattern in our code.
We’ll create a CoffeeMaker
class that includes complex subsystems: a Grinder
, a Boiler
, and a CoffeeMachine
. The CoffeeMakerFacade
will provide a simple interface for the user to make coffee without dealing with the underlying complexity.
// Subsystem 1: Grinder
class Grinder {
fun grindBeans() {
println("Grinding coffee beans...")
}
}
// Subsystem 2: Boiler
class Boiler {
fun heatWater() {
println("Heating water...")
}
}
// Subsystem 3: CoffeeMachine
class CoffeeMachine {
fun brewCoffee() {
println("Brewing coffee...")
}
}
// Facade: CoffeeMakerFacade
class CoffeeMakerFacade(
private val grinder: Grinder,
private val boiler: Boiler,
private val coffeeMachine: CoffeeMachine
) {
fun makeCoffee() {
println("Starting the coffee-making process...")
grinder.grindBeans()
boiler.heatWater()
coffeeMachine.brewCoffee()
println("Coffee is ready!")
}
}
// Client code
fun main() {
// Creating subsystem objects
val grinder = Grinder()
val boiler = Boiler()
val coffeeMachine = CoffeeMachine()
// Creating the Facade
val coffeeMaker = CoffeeMakerFacade(grinder, boiler, coffeeMachine)
// Using the Facade to make coffee
coffeeMaker.makeCoffee()
}
// Output
Starting the coffee-making process...
Grinding coffee beans...
Heating water...
Brewing coffee...
Coffee is ready!
Here,
Subsystems
Grinder
: Handles the coffee bean grinding.Boiler
: Manages the heating of water.CoffeeMachine
: Responsible for brewing the coffee.
Facade
CoffeeMakerFacade
: Simplifies the coffee-making process by providing a single methodmakeCoffee()
, which internally calls the necessary methods from the subsystems in the correct order.
Client Code
- The
main()
function creates instances of the subsystems and the facade. It then callsmakeCoffee()
, demonstrating how the facade abstracts the complexity of the underlying systems.
This is just a simple example to help us understand how the Facade pattern works. Next, we’ll explore another real-world scenario that’s more complex, but we’ll keep it simple.
Facade Pattern in Travel Booking System
Let’s say we want to provide a simple way for users to book their entire travel package in one go without worrying about booking each service (flight, hotel, taxi) individually.
Here’s how the Facade pattern can help!
We’ll create a TravelFacade
to handle flight, hotel, and taxi bookings, making the experience seamless. Each booking service—flight, hotel, and taxi—will have its own class with separate logic, while TravelFacade
provides a unified interface to book the entire package.
Before we write the facade interface, let’s start by defining each booking service.
//Note: It's better to define each service in a separate file.
// FlightBooking.kt
class FlightBooking {
fun bookFlight(from: String, to: String): String {
// Simulate flight booking logic
return "Flight booked from $from to $to"
}
}
// HotelBooking.kt
class HotelBooking {
fun bookHotel(location: String, nights: Int): String {
// Simulate hotel booking logic
return "$nights-night stay booked in $location"
}
}
// TaxiBooking.kt
class TaxiBooking {
fun bookTaxi(pickupLocation: String, destination: String): String {
// Simulate taxi booking logic
return "Taxi booked from $pickupLocation to $destination"
}
}
Now, the TravelFacade
class will act as a single interface that the client interacts with to book their entire travel package.
// TravelFacade.kt
class TravelFacade {
private val flightBooking = FlightBooking()
private val hotelBooking = HotelBooking()
private val taxiBooking = TaxiBooking()
fun bookFullPackage(from: String, to: String, hotelLocation: String, nights: Int): List<String> {
val flightConfirmation = flightBooking.bookFlight(from, to)
val hotelConfirmation = hotelBooking.bookHotel(hotelLocation, nights)
val taxiConfirmation = taxiBooking.bookTaxi("Airport", hotelLocation)
return listOf(flightConfirmation, hotelConfirmation, taxiConfirmation)
}
}
And now, the client can simply use the TravelFacade
without worrying about managing individual bookings for flights, hotels, and taxis
// Main.kt
fun main() {
val travelFacade = TravelFacade()
val travelPackage = travelFacade.bookFullPackage(
from = "New York",
to = "Paris",
hotelLocation = "Paris City Center",
nights = 5
)
// Display the booking confirmations
travelPackage.forEach { println(it) }
}
Output
Flight booked from Pune to Andaman and Nicobar Islands
5-night stay booked in Welcomhotel By ITC Hotels, Marine Hill, Port Blair
Taxi booked from Airport to Welcomhotel By ITC Hotels, Marine Hill, Port Blair
Here,
- Individual services (FlightBooking, HotelBooking, TaxiBooking) have their own booking logic.
TravelFacade
abstracts the booking process, allowing the client to book a complete package with one call tobookFullPackage()
.- The client doesn’t need to understand or interact with each subsystem directly.
Let’s look at another use case in Android. Facade can be applied across different architectures, but I’ll give a more general view so anyone can easily relate and apply it in their code.
Network Communication Facade in Android
Creating a Network Communication Facade in Android with Kotlin helps us streamline and simplify how we interact with different network APIs and methods. This pattern lets us hide the complex details of various network operations, providing the app with a single, easy-to-use interface for making network requests. It’s especially handy when you want to work with multiple networking libraries or APIs in a consistent way.
Here’s a look at how a Network Communication Facade could work in Kotlin
First, let’s start by creating a NetworkFacade
interface.
This interface defines the available methods for network operations (we’ll keep it simple with common methods like GET
and POST
). Any network client can implement this interface to handle requests.
interface NetworkFacade {
suspend fun get(url: String): Result<String>
suspend fun post(url: String, body: Map<String, Any>): Result<String>
// Additional HTTP methods can be added if needed
}
Now, let’s implement this interface with a network client, such as Retrofit or OkHttp. Here, I’ll use OkHttp
as an example.
import okhttp3.OkHttpClient
import okhttp3.Request
import okhttp3.RequestBody.Companion.toRequestBody
import okhttp3.MediaType.Companion.toMediaType
import okhttp3.Response
import org.json.JSONObject
import kotlin.Result
class OkHttpNetworkFacade : NetworkFacade {
private val client = OkHttpClient()
override suspend fun get(url: String): Result<String> {
val request = Request.Builder()
.url(url)
.get()
.build()
return client.newCall(request).execute().use { response ->
if (response.isSuccessful) {
Result.success(response.body?.string() ?: "")
} else {
Result.failure(Exception("GET request failed with code: ${response.code}"))
}
}
}
override suspend fun post(url: String, body: Map<String, Any>): Result<String> {
val json = JSONObject(body).toString()
val requestBody = json.toRequestBody("application/json; charset=utf-8".toMediaType())
val request = Request.Builder()
.url(url)
.post(requestBody)
.build()
return client.newCall(request).execute().use { response ->
if (response.isSuccessful) {
Result.success(response.body?.string() ?: "")
} else {
Result.failure(Exception("POST request failed with code: ${response.code}"))
}
}
}
}
If we need to switch to Retrofit for other services, we can also implement the same interface for Retrofit.
import retrofit2.Retrofit
import retrofit2.converter.gson.GsonConverterFactory
import retrofit2.http.Body
import retrofit2.http.GET
import retrofit2.http.POST
import retrofit2.http.Url
interface RetrofitApiService {
@GET
suspend fun get(@Url url: String): String
@POST
suspend fun post(@Url url: String, @Body body: Map<String, Any>): String
}
class RetrofitNetworkFacade : NetworkFacade {
private val api: RetrofitApiService = Retrofit.Builder()
.baseUrl("https://use-your-base-url-here.com/")
.addConverterFactory(GsonConverterFactory.create())
.build()
.create(RetrofitApiService::class.java)
override suspend fun get(url: String): Result<String> {
return try {
val response = api.get(url)
Result.success(response)
} catch (e: Exception) {
Result.failure(e)
}
}
override suspend fun post(url: String, body: Map<String, Any>): Result<String> {
return try {
val response = api.post(url, body)
Result.success(response)
} catch (e: Exception) {
Result.failure(e)
}
}
}
Now, we can use the NetworkFacade
in the application without worrying about which implementation is in use. This makes it easy to switch between different networking libraries if needed.
class NetworkRepository(private val networkFacade: NetworkFacade) {
suspend fun fetchData(url: String): Result<String> {
return networkFacade.get(url)
}
suspend fun sendData(url: String, data: Map<String, Any>): Result<String> {
return networkFacade.post(url, data)
}
}
To enable flexible configuration, we can use dependency injection (DI) to inject the desired facade implementation—either OkHttpNetworkFacade
or RetrofitNetworkFacade
—when creating the NetworkRepository
.
// Use OkHttpNetworkFacade
val networkRepository = NetworkRepository(OkHttpNetworkFacade())
// Or use RetrofitNetworkFacade
val networkRepository = NetworkRepository(RetrofitNetworkFacade())
Here,
NetworkFacade: This interface defines our network operations. Each client, whether it’s OkHttp
or Retrofit
, can implement this interface, offering different underlying functionalities while maintaining a consistent API for the application.
Result: We use a Result
type to manage successful and failed network calls, which reduces the need for multiple try-catch blocks.
NetworkRepository: The repository interacts with the network clients through the facade. It doesn’t need to know which client is in use, providing flexibility and simplifying testing.
This structure allows us to add more network clients (like Ktor
) in the future or easily swap out existing ones without changing the application logic that relies on network requests.
Benefits of the Facade Pattern
The Facade pattern offers several advantages, especially when dealing with complex systems. Here are a few key benefits:
- Simplifies Usage: It hides the complexity of subsystems and provides a single point of access, making it easier for clients to interact with the system.
- Improves Readability and Maintainability: With a unified interface, understanding the code flow becomes much simpler, which helps in maintaining the code over time.
- Reduces Dependencies: It decouples clients from subsystems, allowing for changes in the underlying system without impacting the client code.
- Increases Flexibility: Changes can be made within the subsystems without affecting the clients using the Facade, providing greater adaptability to future requirements.
When to Use the Facade Pattern
- To Simplify Interactions: Use the Facade pattern when you need to simplify interactions with complex systems or subsystems.
- To Hide Complexity: It’s ideal for hiding complexity from the client, making the system easier to use.
- To Improve Code Readability: The Facade pattern helps enhance code readability by providing a clean, easy-to-understand interface.
- To Maintain a Single Point of Entry: This pattern allows for a single point of entry to different parts of the codebase, which can help manage dependencies effectively.
Disadvantages of the Facade Pattern
While the Facade pattern offers many advantages, it’s essential to consider its drawbacks:
- Potential Over-Simplification: By hiding the underlying complexity, the facade can limit access to the detailed functionality of the subsystem. If users need to interact with specific features not exposed through the facade, they might find it restrictive. For instance, consider a multimedia library with a facade for playing audio and video. If this facade doesn’t allow for adjustments to audio settings like bass or treble, users requiring those tweaks will have to dig into the subsystem, undermining the facade’s purpose.
- Increased Complexity in the Facade: If the facade attempts to manage too many subsystem methods or functionalities, it can become complex itself. This contradicts the goal of simplicity and may require more maintenance. Imagine a facade for a comprehensive payment processing system that tries to include methods for credit card payments, digital wallets, and subscription management. If the facade becomes too feature-rich, it can turn into a large, unwieldy class, making it hard to understand or modify.
- Encapsulation Leakage: The facade pattern can lead to situations where clients become aware of too many details about the subsystems, breaking encapsulation. This can complicate future changes to the subsystem, as clients might depend on specific implementations. For example, if a facade exposes the internal state of a subsystem (like the current status of a printer), clients might start using that state in their logic. If the internal implementation changes (like adopting a new status management system), it could break clients relying on the old state structure.
- Not Always Necessary: For simpler systems, implementing a facade can add unnecessary layers. If the subsystem is already easy to use or doesn’t consist of many components, the facade may be redundant. For example, if you have a simple logging system with a few straightforward methods (like
logInfo
andlogError
), creating a facade to wrap these methods might be overkill. In such cases, direct access to the logging methods may be clearer and easier for developers.
Conclusion
The Facade Pattern is a great choice when you want to simplify complex interactions between multiple classes or subsystems. By creating a single entry point, you can make your code much easier to use and understand. With Kotlin’s class structure and concise syntax, implementing this pattern feels smooth and straightforward.
When used thoughtfully, the Facade Pattern can greatly improve code readability, maintainability, and overall usability—especially in complex projects like multimedia systems, payment gateways, or extensive frameworks. Just remember to balance its benefits with potential drawbacks to ensure it aligns with your design goals.
Happy coding! Enjoy creating clean and intuitive interfaces with the Facade Pattern!