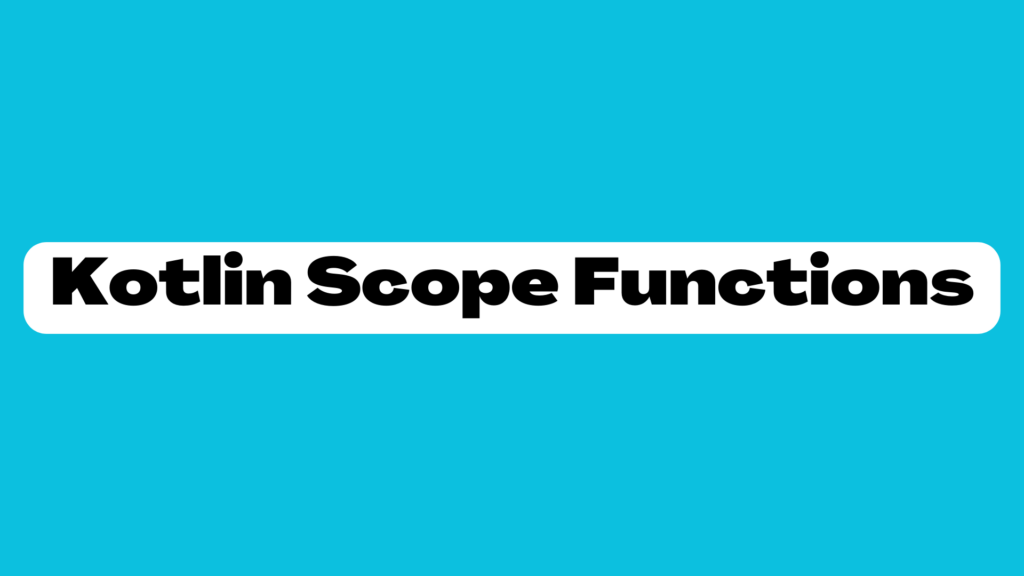
Kotlin is a popular programming language that offers a wide range of features for developers to write concise and expressive code. One of the most powerful features of Kotlin is its scope functions, which are a set of functions that allow you to execute a block of code within the context of an object. In this blog post, we’ll explore Kotlins scope functions and how you can use them to write more efficient and readable code.
Overview of Kotlin Scope Functions
Kotlin offers five scope functions: let, run, with, apply, and also. Each of these functions has its unique use cases and can be used to execute a block of code in a specific context. Let’s take a closer look at each of these functions:
1. Let
The let function allows you to execute a block of code on a nullable object. If the object is null, the block of code is not executed. Otherwise, the block of code is executed, and the result of the block is returned. This function is particularly useful when you need to perform some operations on an object that may or may not be null.
2. Run
The run function is similar to the let function, but it is used to execute a block of code on a non-null object. The result of the block is returned, and the object on which the code is executed is referred to as this within the block.
3. With
The with function is used to execute a block of code on an object without the need for an explicit receiver. This function is particularly useful when you need to perform multiple operations on the same object.
4. Apply
The apply function is similar to the with function, but it is used to modify the object on which the code is executed. The object is returned after the block of code is executed, making this function particularly useful for initializing objects.
5. Also
The also function is used to perform some side effects on an object. The object is returned after the block of code is executed, and this function is particularly useful when you need to log or debug some values.
Benefits of Kotlin Scope Functions
Kotlin scope functions offer a number of benefits for developers, including:
- Concise and readable code: Kotlin scope functions allow us to write more concise and readable code by reducing the need for intermediate variables.
- Reduced boilerplate: Scope functions eliminate the need for redundant code and make it easier to perform operations on objects.
- Improved debugging: Kotlin scope functions provide more visibility into the state of objects by allowing you to perform side effects and log values.
Examples
Let’s take a look at some examples of how we can use Kotlin scope functions in our code.
1. Using let to perform operations on a nullable object:
val name: String? = "softAai"
name?.let { println(it) }
In this example, we use the let function to print the value of the name variable only if it is not null.
2. Using run to initialize an object:
val person = Person().run {
firstName = "amol"
lastName = "pawar"
this
}
In this example, we use the run function to initialize a Person object and set its properties. The object is returned after the block of code is executed.
3. Using apply to modify an object:
val person = Person().apply {
firstName = "Amol"
lastName = "Pawar"
}
In this example, we use the apply function to modify a Person object by setting its properties. The object is returned after the block of code is executed.
4. Using with to perform multiple operations on the same object:
val person = Person()
with(person) {
firstName = "Amol"
lastName = "Pawar"
age = 20
occupation = "Software Developer"
}
In this example, we use the with
function to perform multiple operations on the person
object. The with
function allows us to omit the explicit receiver when accessing the properties and methods of the person
object.
5. Using also to perform side effects on an object:
val person = Person("amol", "pawar", 20)
person.also {
logger.info("Person created: $it")
}
In this example, we use the also
function to log the creation of a person
object using a logger. The also
function allows us to perform a side effect on the person
object and return it afterwards.
Disadvantage of Kotlin Scope Functions
While Kotlins scope functions offer a number of benefits, there are also some potential disadvantages to consider:
- Overuse: It’s possible to overuse scope functions, which can make code less readable and harder to maintain. It’s important to use these functions judiciously and only where they add value.
- Learning curve: While the concept of scope functions is relatively simple, it can take some time to become comfortable using them effectively. New developers may find them confusing at first.
- Performance: While the performance impact of Kotlin scope functions is typically minimal, using them extensively can potentially slow down our code. However, this is rarely a concern in practice.
Conclusion
Overall, the benefits of Kotlin scope functions in Kotlin generally outweigh the potential drawbacks. By using these functions judiciously and with care, we can write more efficient and expressive code.