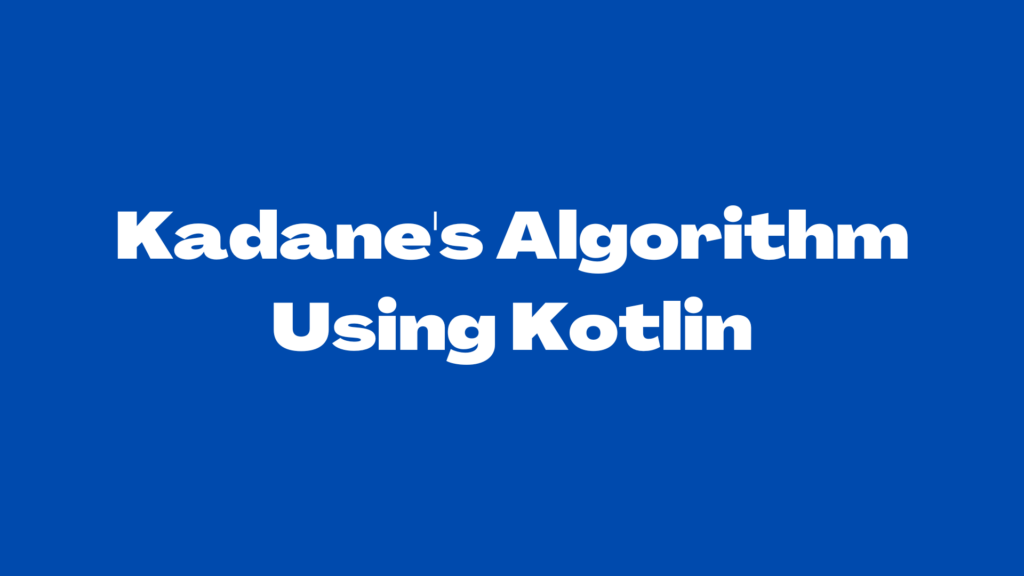
Kotlin Kadane’s algorithm is a well-known algorithm used for finding the maximum subarray sum in a given array. It is an efficient algorithm that works in O(n) time complexity. In this blog, we will discuss Kadane’s algorithm and how to implement it using the Kotlin programming language.
Kotlin Kadane’s Algorithm
Kadane’s algorithm is a dynamic programming algorithm that works by iterating over the array and keeping track of the maximum subarray sum seen so far. The algorithm maintains two variables, max_so_far and max_ending_here, where max_so_far is the maximum subarray sum seen so far, and max_ending_here is the maximum subarray sum that ends at the current index.
The algorithm starts by setting both max_so_far and max_ending_here to the first element of the array. It then iterates over the remaining elements of the array, updating max_ending_here by adding the current element to it. If max_ending_here becomes negative, it is reset to zero, as any subarray with a negative sum cannot be the maximum subarray. If max_ending_here is greater than max_so_far, max_so_far is updated with the value of max_ending_here. At the end of the iteration, max_so_far will contain the maximum subarray sum.
Kotlin Implementation
Now let’s see how we can implement Kadane’s algorithm using Kotlin:
fun maxSubArraySum(arr: IntArray): Int {
var max_so_far = arr[0]
var max_ending_here = arr[0]
for (i in 1 until arr.size) {
max_ending_here = max_ending_here + arr[i]
if (max_ending_here < arr[i])
max_ending_here = arr[i]
if (max_so_far < max_ending_here)
max_so_far = max_ending_here
}
return max_so_far
}
In this implementation, we first initialize max_so_far and max_ending_here to the first element of the array. We then loop over the remaining elements of the array and update max_ending_here by adding the current element to it. If max_ending_here becomes negative, it is reset to zero. If max_ending_here is greater than max_so_far, max_so_far is updated with the value of max_ending_here. Finally, the function returns max_so_far.
Let’s test our implementation with an example:
fun main() {
val arr = intArrayOf(-2, -3, 4, -1, -2, 1, 5, -3)
val maxSum = maxSubArraySum(arr)
println(\"Maximum subarray sum is: $maxSum\")
}
Output:
Maximum subarray sum is: 7
In this example, we have an array of integers, and we want to find the maximum subarray sum. Our implementation correctly returns 7, which is the maximum subarray sum.
Conclusion
Kadane’s algorithm is a simple yet powerful algorithm for finding the maximum subarray sum in an array. In this blog, we have seen how to implement Kadane’s algorithm using Kotlin. This implementation works in O(n) time complexity, making it an efficient algorithm for solving the maximum subarray problem.