Android development has experienced tremendous evolution over the years. Among the most significant changes has been the shift in how developers build user interfaces (UIs). Traditionally, Android developers relied on XML-based layouts for UI creation. While this method served its purpose, it came with several limitations and inefficiencies. Enter Jetpack Compose, a revolutionary UI toolkit that eliminates many of the challenges developers faced with traditional Android UI frameworks.
In this blog, we will explore the drawbacks of traditional Android UI toolkits and how Jetpack Compose addresses these issues with its powerful, modern core features. By the end of this post, you’ll understand why Jetpack Compose is a game-changer for Android UI development and how it can streamline your development process.
Traditional Android UI Toolkit: Drawbacks and Limitations
Before we dive into the core features of Jetpack Compose, let’s first take a look at the challenges that Android developers have faced with traditional UI toolkits.
1. Complex XML Layouts
In traditional Android development, UI elements are defined in XML files, which are then “inflated” into the activity or fragment. This approach introduces several complexities:
- Verbose Code: XML layouts tend to be verbose, requiring extensive boilerplate code to define simple UI elements.
- Separation of UI and Logic: With XML layouts, UI components are separated from the logic that controls them. This makes it harder to manage and maintain the app’s UI, especially as the app grows.
- Difficulty in Dynamic Changes: Updating UI components dynamically (e.g., changing a button’s text or visibility) requires cumbersome logic and manual updates to the views, leading to more maintenance overhead.
2. View Binding and FindViewById
Before the introduction of View Binding and Kotlin Extensions, Android developers used the findViewById() method to reference views from XML layouts in their activities. This approach has several drawbacks:
- Null Safety: Using findViewById() can result in null pointer exceptions if a view doesn’t exist in the layout, leading to potential crashes.
- Repetitive Code: Developers have to call findViewById() for each UI element they want to reference, resulting in repetitive and error-prone code.
- Complexity in Managing State: Managing UI state and dynamically updating views based on that state required a lot of boilerplate code and manual intervention.
3. Hard to Maintain Complex UIs
As apps grow in complexity, managing and maintaining the UI becomes more difficult. Developers often need to manage multiple layout files and ensure that changes to one layout do not break others. This becomes especially challenging when dealing with screen sizes, orientations, and platform variations.
4. Limited Flexibility in Layouts
XML layouts are not particularly flexible when it comes to defining complex layouts with intricate customizations. This often requires developers to write custom views or use third-party libraries, adding extra complexity to the project.
The Rise of Jetpack Compose: Modern UI Toolkit
Jetpack Compose is a declarative UI toolkit that allows Android developers to create UIs using Kotlin programming language. Unlike traditional XML-based layouts, Jetpack Compose defines the UI within Kotlin code, making it easier to work with and more dynamic. By leveraging Kotlin’s power, Jetpack Compose provides a more modern, flexible, and efficient way to develop Android UIs.
Now, let’s take a deep dive into how Jetpack Compose overcomes the drawbacks of traditional Android UI toolkits and why it’s quickly becoming the go-to choice for Android development.
1. Declarative UI: Simplicity and Flexibility
One of the key principles behind Jetpack Compose is the declarative approach to UI development. In traditional Android development, you would have to describe the layout of UI elements and the logic separately (in XML and Java/Kotlin code). In Jetpack Compose, everything is done inside the Kotlin code, making it much simpler and more cohesive.
With Jetpack Compose, you describe the UI’s appearance by defining composable functions, which are functions that define how UI elements should look based on the app’s current state. Here’s a simple example of a button in Jetpack Compose:
@Composable
fun GreetingButton(onClick: () -> Unit) {
Button(onClick = onClick) {
Text("Click Me!")
}
}
The declarative nature allows for UI elements to be modified easily by changing the state, reducing the complexity of managing UI components manually.
2. No More findViewById or View Binding
One of the pain points of traditional Android UI development was the need to reference views using findViewById()
or even use View Binding. These approaches added complexity and could result in null pointer exceptions or repetitive code.
With Jetpack Compose, there is no need for findViewById()
because all UI elements are created directly in Kotlin code. Instead of manually referencing views, you define UI components using composables. Additionally, since Jetpack Compose uses state management, the UI automatically updates when the state changes, so there’s no need for manual intervention.
3. Less Boilerplate Code
Jetpack Compose significantly reduces the need for boilerplate code. In traditional XML-based development, a UI element like a button might require multiple lines of code across different files. In contrast, Jetpack Compose reduces it to just a few lines of Kotlin code, which leads to cleaner and more maintainable code.
For instance, creating a TextField in Jetpack Compose is extremely simple:
@Composable
fun SimpleTextField() {
var text by remember { mutableStateOf("") }
TextField(value = text, onValueChange = { text = it })
}
As you can see, there’s no need for complex listeners or setters—everything is managed directly within the composable function.
4. Powerful State Management
State management is an essential aspect of building dynamic UIs. In traditional Android UI toolkits, managing state across different views could be cumbersome. Developers had to rely on LiveData
, ViewModels
, or other complex state management tools to handle UI updates.
Jetpack Compose, however, handles state seamlessly. It allows developers to use state in a much more intuitive way, with mutableStateOf and remember helping to store and manage state directly within composables. When the state changes, the UI automatically recomposes to reflect the new state, saving developers from having to manually refresh views.
@Composable
fun Counter() {
var count by remember { mutableStateOf(0) }
Button(onClick = { count++ }) {
Text("Count: $count")
}
}
This simple, dynamic approach to state management is one of the core reasons why Jetpack Compose is considered a powerful modern toolkit.
5. Customizable and Reusable Components
Jetpack Compose encourages the creation of reusable UI components. Composables can be easily customized and combined to create complex UIs without sacrificing maintainability. In traditional Android development, developers often need to write custom views or use third-party libraries to achieve flexibility in their layouts.
In Jetpack Compose, developers can create custom UI components effortlessly by combining smaller composables and applying modifiers to adjust their behavior and appearance. For example:
@Composable
fun CustomCard(content: @Composable () -> Unit) {
Card(modifier = Modifier.padding(16.dp), elevation = 8.dp) {
content()
}
}
This flexibility allows for more scalable and maintainable UI code, which is particularly beneficial as the app grows.
Jetpack Compose vs. Traditional UI: The Key Differences
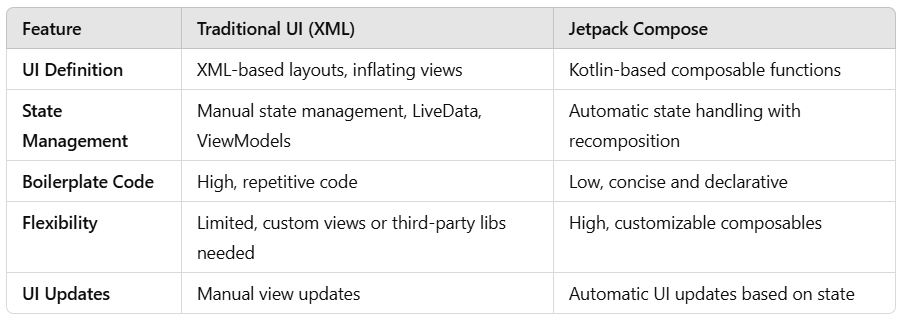
Core Features of Jetpack Compose
- Declarative UI: Build UIs by defining composables, leading to a cleaner and more intuitive way of designing apps.
- State Management: Automatic UI recomposition based on state changes, reducing manual updates.
- Reusable Components: Easy to create modular, reusable, and customizable UI elements.
- Kotlin Integration: Leverages Kotlin’s features for more concise, readable, and maintainable code.
- No XML: Eliminates the need for XML layouts, improving development speed and reducing errors.
Conclusion
Jetpack Compose is not just another Android UI toolkit; it is a game-changing approach to UI development. It addresses the drawbacks of traditional Android UI toolkits by providing a declarative, flexible, and efficient way to build user interfaces. By eliminating the need for XML layouts, simplifying state management, and promoting reusability, Jetpack Compose empowers developers to create modern Android UIs faster and with less complexity.
As Android development continues to evolve, Jetpack Compose is poised to be the future of UI design, and adopting it now can help streamline your development process and lead to better, more maintainable apps.