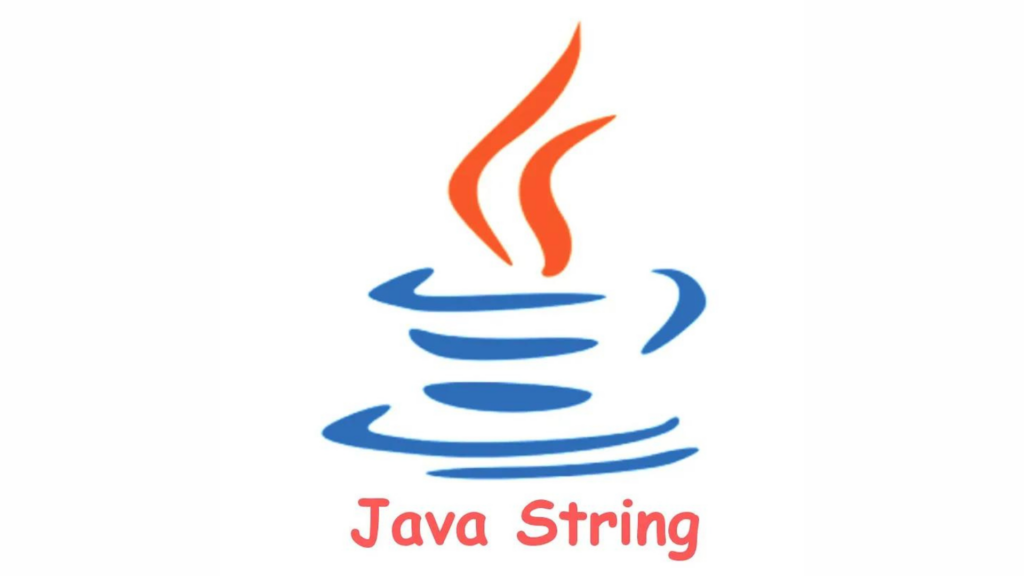
Java, one of the most widely used programming languages, offers a robust and versatile class for handling textual data – the String
class. Understanding the intricacies of Java Strings is crucial for any Java developer. In this comprehensive guide, we’ll explore the important concepts related to Java Strings, covering everything from basic operations to advanced topics.
String is one of the most commonly used data types in Java programming. Understanding the concept of Strings is crucial for any Java developer. In this blog post, we will discuss some of the important concepts related to Strings in Java.
Immutable vs Mutable Java Strings
In Java, Strings are immutable objects, which means once we create a string object, we cannot perform any changes in the existing object. If we are trying to perform any change, a new object will be created with those changes. This non-changeable behavior is known as the immutability of Strings.
For example,
String s = new String("softAai");
s.concat("Apps");
System.out.println(s); //softAai
In the above code, the concat()
method is called on the string object s
, but the original string remains unchanged. This is because a new string object is created with the concatenated value, but it doesn\’t hold any reference in this case, and hence it is eligible for garbage collection.
On the other hand, if we use StringBuffer
instead of String
, we can perform any change in the existing object. This changeable behavior is known as the mutability of the StringBuffer object.
For example,
StringBuffer sb = new StringBuffer("softAai");
sb.append("Apps");
System.out.println(sb); // softAaiApps
In the above code, the append()
method is called on the StringBuffer
object sb
, and the original object is modified with the appended value.
“==” vs .equals()
In Java, the ==
operator compares the references of two objects, while the .equals()
method compares the content of two objects. In the case of Strings, the .equals()
method is overridden for content comparison.
For example,
String s1 = new String("softAai");
String s2 = new String("softAai");
System.out.println(s1 == s2); // false
System.out.println(s1.equals(s2)); // true
In the above code, two different string objects are created, but their contents are the same. The ==
operator compares the references of these objects, which are different, and hence it returns false. But the .equals()
method compares the content of these objects, which are the same, and hence it returns true.
On the other hand, the equals()
method is not overridden for content comparison in StringBuffer
. Hence, the equals()
method of the Object
class is executed, which compares the references of the objects.
For example,
StringBuffer sb1 = new StringBuffer("softAai");
StringBuffer sb2 = new StringBuffer("softAai");
System.out.println(sb1 == sb2); // false
System.out.println(sb1.equals(sb2)); // false
In the above code, two different StringBuffer
objects are created, and their contents are the same. Both the ==
operator and the .equals()
method return false because they compare the references of these objects, which are different.
Conclusion
The concepts of immutability and mutability in strings and the differences between ==
and .equals()
operators are important to understand. By understanding these concepts, you can better optimize memory usage and avoid common pitfalls when working with strings in Java