In the world of software development, design patterns emerge as essential tools, offering time-tested solutions to common challenges. These patterns are not just arbitrary guidelines but are structured, proven approaches derived from the collective experience of seasoned developers. By understanding and applying design patterns, developers can craft efficient, maintainable, and scalable software systems.
Introduction to Design Patterns
Design patterns can be thought of as reusable solutions to recurring problems in software design. Imagine you’re building a house; instead of starting from scratch each time, you use blueprints that have been refined over years of experience. Similarly, design patterns serve as blueprints for solving specific problems in software development. They provide a structured approach that helps in tackling common issues such as object creation, object interaction, and code organization.
From Architecture to Software
The concept of design patterns originated outside the realm of software, rooted in architecture. In the late 1970s, architect Christopher Alexander introduced the idea of design patterns in his book “A Pattern Language.” Alexander and his colleagues identified recurring problems in architectural design and proposed solutions that could be applied across various contexts. These solutions were documented as patterns, forming a language that architects could use to create more functional and aesthetically pleasing spaces.
This idea of capturing and reusing solutions resonated with the software community, which faced similar challenges in designing complex systems. By the 1980s and early 1990s, software developers began to recognize the potential of applying design patterns to code, adapting Alexander’s concepts to address common problems in software architecture.
The Gang of Four
The formalization of design patterns in software development took a significant leap forward with the publication of “Design Patterns: Elements of Reusable Object-Oriented Software” in 1994. This book, authored by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides—collectively known as the “Gang of Four” (GoF)—became a seminal work in the field.
Creational Patterns: Focused on object creation mechanisms, ensuring that a system can be efficiently extended without knowing the exact classes that will be instantiated. Examples include the Singleton, Factory, and Builder patterns.
Structural Patterns: Concerned with the composition of classes or objects, simplifying complex relationships and providing flexible solutions for building larger structures. Examples include the Adapter, Composite, and Decorator patterns.
Behavioral Patterns: Focused on communication between objects, defining how objects interact and distribute responsibilities. Examples include the Observer, Strategy, and Command patterns.
Categories of Design Patterns
The three main categories of design patterns are:
- Creational Patterns: Deal with object creation mechanisms.
- Structural Patterns: Focus on the composition of classes or objects.
- Behavioral Patterns: Concern the interaction and responsibility of objects.
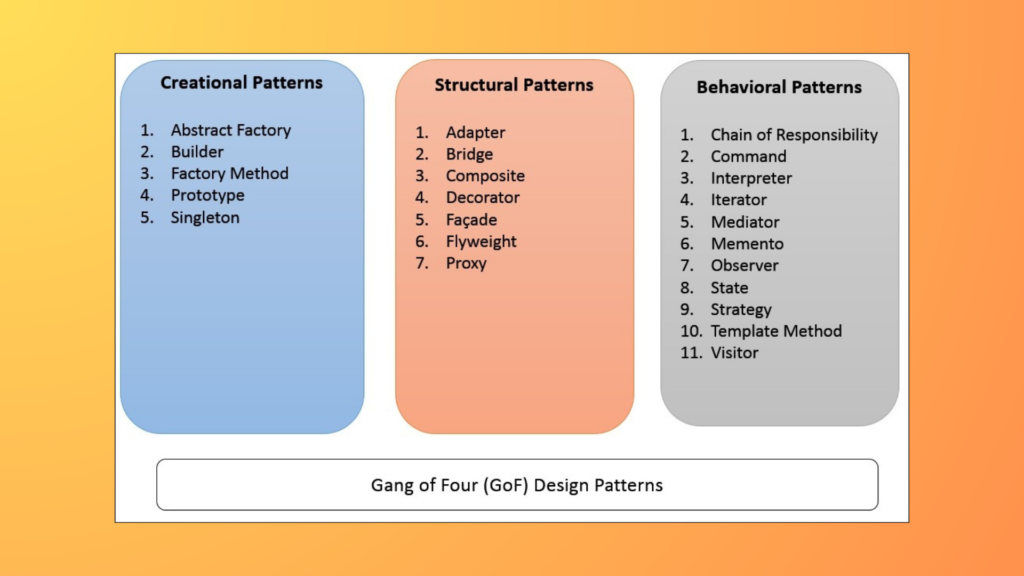
Creational Patterns
These patterns deal with object creation mechanisms, trying to create objects in a manner suitable for the situation.
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
- Factory Method: Defines an interface for creating an object, but lets subclasses alter the type of objects that will be created.
- Abstract Factory: Provides an interface for creating families of related or dependent objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation.
- Prototype: Creates new objects by copying an existing object, known as the prototype.
Structural Patterns
These patterns focus on composing classes or objects into larger structures, like classes or object composition.
- Adapter: Allows incompatible interfaces to work together by wrapping an existing class with a new interface.
- Bridge: Separates an object’s abstraction from its implementation so that the two can vary independently.
- Composite: Composes objects into tree structures to represent part-whole hierarchies.
- Decorator: Adds responsibilities to objects dynamically.
- Facade: Provides a simplified interface to a complex subsystem.
- Flyweight: Reduces the cost of creating and manipulating a large number of similar objects.
- Proxy: Provides a surrogate or placeholder for another object to control access to it.
Behavioral Patterns
These patterns are concerned with algorithms and the assignment of responsibilities between objects.
- Chain of Responsibility: Passes a request along a chain of handlers, where each handler can process the request or pass it on.
- Command: Encapsulates a request as an object, thereby allowing for parameterization and queuing of requests.
- Interpreter: Defines a representation of a grammar for a language and an interpreter to interpret sentences in the language.
- Iterator: Provides a way to access elements of a collection sequentially without exposing its underlying representation.
- Mediator: Reduces chaotic dependencies between objects by having them communicate through a mediator object.
- Memento: Captures and externalizes an object’s internal state without violating encapsulation, so it can be restored later.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
- State: Allows an object to alter its behavior when its internal state changes.
- Strategy: Defines a family of algorithms, encapsulates each one, and makes them interchangeable.
- Template Method: Defines the skeleton of an algorithm in a method, deferring some steps to subclasses.
- Visitor: Represents an operation to be performed on elements of an object structure, allowing new operations to be defined without changing the classes of the elements on which it operates.”
Why Do We Use Design Patterns?
Design patterns aren’t just buzzwords—they’re powerful tools that make software development smoother and more efficient. Here’s why they’re so valuable:
- Reusability: Design patterns provide tried-and-true solutions to common problems. Instead of reinventing the wheel, developers can reuse these patterns, saving time and effort while promoting modularity in software systems.
- Improved Communication: Design patterns create a shared language among developers. When everyone understands the same patterns, it’s easier to discuss and make design decisions as a team.
- Best Practices: Design patterns encapsulate the wisdom of experienced developers. For those new to the field, they offer a way to learn from the best, ensuring that your code follows industry standards.
- Maintainability: Using design patterns often leads to cleaner, more organized code. This makes it easier to update, debug, and extend the codebase as the project evolves.
- Easier Problem-Solving: Design patterns provide a structured approach to tackling complex problems. They help break down big issues into manageable parts, making the development process more efficient.
Design patterns are essential tools that enhance code quality, collaboration, and problem-solving, making them a key asset in any developer’s toolkit.
How Do We Choose the Right Design Pattern
Design patterns are like cool tools in your developer toolbox, but it’s important to use them wisely. Here’s what you need to keep in mind:
- Think About the Situation: Design patterns shine in the right context. But using them just for the sake of it might not always be the best move. Make sure the pattern fits the problem you’re solving.
- Keep It Simple: Sometimes, the simplest solution is the best one. Don’t overcomplicate things by forcing a pattern where a straightforward approach would do the job.
- Watch Out for Speed Bumps: Design patterns can sometimes slow down your program. Weigh the pros and cons to see if the benefits outweigh the potential performance hit.
- Be Ready to Change: As your project grows, what worked before might not be the best choice anymore. Stay flexible and be prepared to adapt as needed.
Using design patterns is like having a set of handy tools at your disposal. Just remember that not every tool is right for every job. Choose the ones that best fit the situation, and your code will be stronger and more reliable!
Conclusion
The journey of design patterns from architecture to software highlights the power of abstraction and the value of shared knowledge. From their origins in the work of Christopher Alexander to the seminal contributions of the Gang of Four, design patterns have become a cornerstone of software design, enabling developers to build robust, maintainable systems with greater efficiency.
As software development continues to evolve, so too will design patterns, adapting to new challenges and opportunities. By understanding the history and evolution of design patterns, developers can better appreciate their importance and apply them effectively in their work, ensuring that their solutions stand the test of time.