Design patterns are the cornerstone of software design, providing standardized solutions to common problems. Among the Gang of Four (GoF) design patterns, creational design patterns are particularly crucial as they focus on object creation mechanisms. In this blog, we will delve into the five GoF creational design patterns in Kotlin: Singleton, Factory Method, Abstract Factory, Builder, and Prototype. We’ll explore each pattern’s purpose, structure, and practical usage in Kotlin, complete with code examples.
Creational Design Patterns: Singleton Pattern
The Singleton pattern restricts the instantiation of a class to one “single” instance. This is useful when exactly one object is needed to coordinate actions across a system. Examples include database connections, logging, configuration settings, or even hardware interface access.
Why and When to Use the Singleton Pattern
The Singleton pattern is often used when:
- You have a resource-heavy object that should be created only once, like a database connection.
- You need global access to an object, like application-wide logging, configuration management, or caching.
- You want to ensure consistency, such as using the same state across multiple activities in Android.
Implementation of Singleton
Implementing the Singleton pattern requires careful consideration to ensure thread safety, lazy or eager initialization, and prevention of multiple instances through serialization or reflection.
Here are different ways to implement the Singleton design pattern:
Singleton in Kotlin: A Built-In Solution
Kotlin simplifies the implementation of the Singleton pattern by providing the object
keyword. This keyword allows you to define a class that automatically has a single instance. Here’s a simple example:
object DatabaseConnection {
init {
println("DatabaseConnection instance created")
}
fun connect() {
println("Connecting to the database...")
}
}
fun main() {
DatabaseConnection.connect()
DatabaseConnection.connect()
}
In this example, DatabaseConnection
is a Singleton. The first time DatabaseConnection.connect()
is called, the instance is created, and the message “DatabaseConnection instance created” is printed. Subsequent calls to connect()
will use the same instance without reinitializing it.
Advantages of Kotlin’s “object
” Singleton
- Simplicity: The
object
keyword makes the implementation of the Singleton pattern concise and clear. - Thread Safety: Kotlin ensures thread safety for objects declared using the
object
keyword. This means that you don’t have to worry about multiple threads creating multiple instances of the Singleton. - Eager Initialization: The Singleton instance is created at the time of the first access, making it easy to manage resource allocation.
Lazy Initialization
In some cases, you might want to delay the creation of the Singleton instance until it’s needed. Kotlin provides the lazy
function, which can be combined with a by
delegation to achieve this:
class ConfigManager private constructor() {
companion object {
val instance: ConfigManager by lazy { ConfigManager() }
}
fun loadConfig() {
println("Loading configuration...")
}
}
fun main() {
val config = Configuration.getInstance1()
config.loadConfig()
}
Here, the ConfigManager
instance is created only when instance.loadConfig()
is called for the first time. This is particularly useful in scenarios where creating the instance is resource-intensive.
Singleton with Parameters
Sometimes, you might need to pass parameters to the Singleton. However, the object
keyword does not allow for constructors with parameters. One approach to achieve this is to use a regular class with a private constructor and a companion object:
class Logger private constructor(val logLevel: String) {
companion object {
@Volatile private var INSTANCE: Logger? = null
fun getInstance(logLevel: String): Logger =
INSTANCE ?: synchronized(this) {
INSTANCE ?: Logger(logLevel).also { INSTANCE = it }
}
}
fun log(message: String) {
println("[$logLevel] $message")
}
}
In this example, the Logger
class is a Singleton that takes a logLevel
parameter. The getInstance
method ensures that only one instance is created, even when accessed from multiple threads. The use of @Volatile
and synchronized
blocks ensures thread safety.
Thread-Safe Singleton (Synchronized Method)
When working in multi-threaded environments (e.g., Android), ensuring that the Singleton instance is thread-safe is crucial. In Kotlin, the object
keyword is inherently thread-safe. However, when using manual Singleton implementations, you need to take additional care.
class ThreadSafeSingleton private constructor() {
companion object {
@Volatile
private var instance: ThreadSafeSingleton? = null
fun getInstance(): ThreadSafeSingleton {
return instance ?: synchronized(this) {
instance ?: ThreadSafeSingleton().also { instance = it }
}
}
}
}
Here, the most important approach used is the double-checked locking pattern. Let’s first see what it is, then look at the above code implementation for a better understanding.
Double-Checked Locking
This method reduces the overhead of synchronization by checking the instance twice before creating it. The @Volatile
annotation ensures visibility of changes to variables across threads.
class Singleton private constructor() {
companion object {
@Volatile
private var instance: Singleton? = null
fun getInstance(): Singleton {
if (instance == null) {
synchronized(this) {
if (instance == null) {
instance = Singleton()
}
}
}
return instance!!
}
}
}
Here’s how both approaches work: This implementation uses double-checked locking. First, the instance is checked outside of the synchronized block. If it’s not null, the instance is returned directly. If it is null, the code enters the synchronized block to ensure that only one thread can initialize the instance. The instance is then checked again inside the block to prevent multiple threads from initializing it simultaneously.
Bill Pugh Singleton (Initialization-on-demand holder idiom)
The Bill Pugh Singleton pattern, or the Initialization-on-Demand Holder Idiom, ensures that the Singleton instance is created only when it is requested for the first time, leveraging the classloader mechanism to ensure thread safety.
Key Points:
- Lazy Initialization: The Singleton instance is not created until the
getInstance()
method is called. - Thread Safety: The class initialization phase is thread-safe, ensuring that only one thread can execute the initialization logic.
- Efficient Performance: No synchronized blocks are used, which avoids the potential performance hit.
class BillPughSingleton private constructor() {
companion object {
// Static inner class - inner classes are not loaded until they are referenced.
private class SingletonHolder {
companion object {
val INSTANCE = BillPughSingleton()
}
}
// Method to get the singleton instance
fun getInstance(): BillPughSingleton {
return SingletonHolder.INSTANCE
}
}
// Any methods or properties for your Singleton can be defined here.
fun showMessage() {
println("Hello, I am Bill Pugh Singleton in Kotlin!")
}
}
fun main() {
// Get the Singleton instance
val singletonInstance = BillPughSingleton.getInstance()
// Call a method on the Singleton instance
singletonInstance.showMessage()
}
====================================================================
O/P - Hello, I am Bill Pugh Singleton in Kotlin!
Explanation of the Implementation
- Private Constructor: The
private constructor()
prevents direct instantiation of the Singleton class. - Companion Object: In Kotlin, the
companion object
is used to hold the Singleton instance. The actual instance is inside theSingletonHolder
companion object, ensuring it is not created until needed. - Lazy Initialization: The
SingletonHolder.INSTANCE
is only initialized whengetInstance()
is called for the first time, ensuring the Singleton is created lazily. - Thread Safety: The Kotlin classloader handles the initialization of the
SingletonHolder
class, ensuring that only one instance of the Singleton is created even if multiple threads try to access it simultaneously. In short, The JVM guarantees that static inner classes are initialized only once, ensuring thread safety without explicit synchronization.
Enum Singleton
In Kotlin, you might wonder why you’d choose an enum
for implementing a Singleton when the object
keyword provides a straightforward and idiomatic way to create singletons. The primary reason to use an enum as a Singleton is its inherent protection against multiple instances and serialization-related issues.
Key Points:
- Thread Safety: Enum singletons are thread-safe by default.
- Serialization: The JVM guarantees that during deserialization, the same instance of the enum is returned, which isn’t the case with other singleton implementations unless you handle serialization explicitly.
- Prevents Reflection Attacks: Reflection cannot be used to instantiate additional instances of an enum, providing an additional layer of safety.
Implementing an Enum Singleton in Kotlin is straightforward. Here’s an example:
enum class Singleton {
INSTANCE;
fun doSomething() {
println("Doing something...")
}
}
fun main() {
Singleton.INSTANCE.doSomething()
}
Explanation:
enum class Singleton
: Defines an enum with a single instance,INSTANCE
.doSomething
: A method within the enum that can perform any operation. This method can be expanded to include more complex logic as needed.- Usage: Accessing the singleton is as simple as calling
Singleton.INSTANCE
.
Benefits of Enum Singleton
Using an enum to implement a Singleton in Kotlin comes with several benefits:
- Simplicity: The code is simple and easy to understand, with no need for explicit thread-safety measures or additional synchronization code.
- Serialization Safety: Enum singletons handle serialization automatically, ensuring that the Singleton property is maintained across different states of the application.
- Reflection Immunity: Unlike traditional Singleton implementations, enums are immune to attacks via reflection, adding a layer of security.
Singleton in Android Development
In Android, Singletons are often used for managing resources like database connections, shared preferences, or network clients. However, care must be taken to avoid memory leaks, especially when dealing with context-dependent objects.
Example with Android Context:
object SharedPreferenceManager {
private const val PREF_NAME = "MyAppPreferences"
private var preferences: SharedPreferences? = null
fun init(context: Context) {
if (preferences == null) {
preferences = context.getSharedPreferences(PREF_NAME, Context.MODE_PRIVATE)
}
}
fun saveData(key: String, value: String) {
preferences?.edit()?.putString(key, value)?.apply()
}
fun getData(key: String): String? {
return preferences?.getString(key, null)
}
}
// Usage in Application class
class MyApp : Application() {
override fun onCreate() {
super.onCreate()
SharedPreferenceManager.init(this)
}
}
Context Initialization: The init
method ensures that the SharedPreferenceManager
is initialized with a valid context, typically from the Application class.
Avoiding Memory Leaks: By initializing with the Application context, we prevent memory leaks that could occur if the Singleton holds onto an Activity or other short-lived context.
Network Client Singleton
object NetworkClient {
val retrofit: Retrofit by lazy {
Retrofit.Builder()
.baseUrl("https://api.softaai.com/")
.addConverterFactory(GsonConverterFactory.create())
.build()
}
}
In this example, NetworkClient
is a Singleton that provides a global Retrofit
instance for making network requests. By using the object
keyword, the instance is lazily initialized the first time it is accessed and shared throughout the application.
Singleton with Dependency Injection
In modern Android development, Dependency Injection (DI) is a common pattern, often implemented using frameworks like Dagger or Hilt. The Singleton pattern can be combined with DI to manage global instances efficiently.
Hilt Example:
@Singleton
class ApiService @Inject constructor() {
fun fetchData() {
println("Fetching data from API")
}
}
// Usage in an Activity or Fragment
@AndroidEntryPoint
class MainActivity : AppCompatActivity() {
@Inject
lateinit var apiService: ApiService
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
apiService.fetchData()
}
}
@Singleton
: The @Singleton
annotation ensures that ApiService
is treated as a Singleton within the DI framework.
@Inject
: This annotation is used to inject the ApiService
instance wherever needed, like in an Activity or Fragment.
When to Use the Singleton Pattern
While the Singleton pattern is useful, it should be used judiciously. Consider using it in the following scenarios:
- Centralized Management: When you need a single point of control for a shared resource, such as a configuration manager, database connection, or thread pool.
- Global State: When you need to maintain a global state across the application, such as user preferences or application settings.
- Stateless Utility Classes: When creating utility classes that don’t need to maintain state, Singleton can provide a clean and efficient implementation.
Caution: Overuse of Singletons can lead to issues like hidden dependencies, difficulties in testing, and reduced flexibility. Always assess whether a Singleton is the best fit for your use case.
Drawbacks and Considerations
Despite its advantages, the Singleton pattern has some drawbacks:
- Global State: Singleton can introduce hidden dependencies across the system, making the code harder to understand and maintain.
- Testing: Singleton classes can be difficult to test in isolation due to their global nature. It might be challenging to mock or replace them in unit tests.
- Concurrency: While Kotlin’s
object
andlazy
initialization handle thread safety well, improper use of Singleton in multithreaded environments can lead to synchronization issues if not handled carefully.

Factory Method Design Patterns
Object creation in software development can feel routine, but what if you could streamline the process? The Factory Method Design Pattern does just that, allowing you to create objects without tightly coupling your code to specific classes. In Kotlin, known for its simplicity and versatility, this pattern is even more effective, helping you build scalable, maintainable applications with ease. Let’s explore why the Factory Method is a must-know tool for Kotlin developers by first looking at a common problem it solves.
Problem
Imagine you’re working on an app designed to simplify transportation bookings. At first, you’re just focusing on Taxis, a straightforward service. But as user feedback rolls in, it becomes clear: people are craving more options. They want to book Bikes, Buses, and even Electric Scooters—all from the same app.
So, your initial setup for Taxis might look something like this:
class Taxi {
fun bookRide() {
println("Taxi ride booked!")
}
}
class Bike {
fun bookRide() {
println("Bike ride booked!")
}
}
class App {
fun bookTransport(type: String) {
when (type) {
"taxi" -> Taxi().bookRide()
"bike" -> Bike().bookRide()
else -> println("Transport not available!")
}
}
}
But, here’s the problem:
Scalability: Each time you want to introduce a new transportation option—like a Bus or an Electric Scooter—you find yourself diving into the App class to make adjustments. This can quickly become overwhelming as the number of transport types grows.
Maintainability: As the App class expands to accommodate new features, it becomes a tangled mess, making it tougher to manage and test. What started as a simple setup turns into a complicated beast.
Coupling: The app is tightly linked with specific transport classes, so making a change in one area often means messing with others. This tight coupling makes it tricky to update or enhance features without unintended consequences.
The Solution – Factory Method Design Pattern
We need a way to decouple the transport creation logic from the App
class. This is where the Factory Method Design Pattern comes in. Instead of hard-coding which transport class to instantiate, we delegate that responsibility to a method in a separate factory. This approach not only simplifies your code but also allows for easier updates and expansions.
Step 1: Define a Common Interface
First, we create a common interface that all transport types (Taxi, Bike, Bus, etc.) will implement. This ensures our app can handle any transport type without knowing the details of each one.
interface Transport {
fun bookRide()
}
Now, we make each transport type implement this interface:
class Taxi : Transport {
override fun bookRide() {
println("Taxi ride booked!")
}
}
class Bike : Transport {
override fun bookRide() {
println("Bike ride booked!")
}
}
class Bus : Transport {
override fun bookRide() {
println("Bus ride booked!")
}
}
Step 2: Create the Factory
Now, we create a Factory class. The factory will decide which transport object to create based on input, but the app itself won’t need to know the details.
abstract class TransportFactory {
abstract fun createTransport(): Transport
}
Step 3: Implement Concrete Factories
For each transport type, we create a corresponding factory class that extends TransportFactory
. Each factory knows how to create its specific type of transport:
class TaxiFactory : TransportFactory() {
override fun createTransport(): Taxi {
return Taxi()
}
}
class BikeFactory : TransportFactory() {
override fun createTransport(): Bike {
return Bike()
}
}
class BusFactory : TransportFactory() {
override fun createTransport(): Bus {
return Bus()
}
}
Step 4: Use the Factory in the App
Now, we update our app to use the factory classes instead of directly creating transport objects. The app no longer needs to know which transport it’s booking — the factory handles that.
class App {
private lateinit var transportFactory: TransportFactory
fun setTransportFactory(factory: TransportFactory) {
this.transportFactory = factory
}
fun bookRide() {
val transport: Transport = transportFactory.createTransport()
transport.bookRide()
}
}
Step 5: Putting It All Together
Now, you can set different factories at runtime, depending on the user’s choice of transport, without modifying the App
class.
fun main() {
val app = App()
// To book a Taxi
app.setTransportFactory(TaxiFactory())
app.bookRide() // Output: Taxi ride booked!
// To book a Bike
app.setTransportFactory(BikeFactory())
app.bookRide() // Output: Bike ride booked!
// To book a Bus
app.setTransportFactory(BusFactory())
app.bookRide() // Output: Bus ride booked!
}
Here’s how the Factory Method Solves the Problem:
- Decoupling: The
App
class no longer needs to know the details of each transport type. It only interacts with theTransportFactory
andTransport
interface. - Scalability: Adding new transport types (like Electric Scooter) becomes easier. You simply create a new class (e.g.,
ScooterFactory
) without changing existing code inApp
. - Maintainability: Each transport creation logic is isolated in its own factory class, making the codebase cleaner and easier to maintain.
What is the Factory Method Pattern?
The Factory Method pattern defines an interface for creating an object, but allows subclasses to alter the type of objects that will be created. Instead of calling a constructor directly to create an object, the pattern suggests calling a special factory method to create the object. This allows for more flexibility and encapsulation.
The Factory Method pattern is also called the “virtual constructor” pattern. It’s used in core Java libraries, like java.util.Calendar.getInstance()
and java.nio.charset.Charset.forName()
.
Why Use the Factory Method?
- Loose Coupling: It helps keep code parts separate, so changes in one area won’t affect others much.
- Flexibility: Subclasses can choose which specific class of objects to create, making it easier to add new features or change existing ones without changing the code that uses these objects.
In short, the Factory Method pattern lets a parent class define the process of creating objects, but leaves the choice of the specific object type to its subclasses.
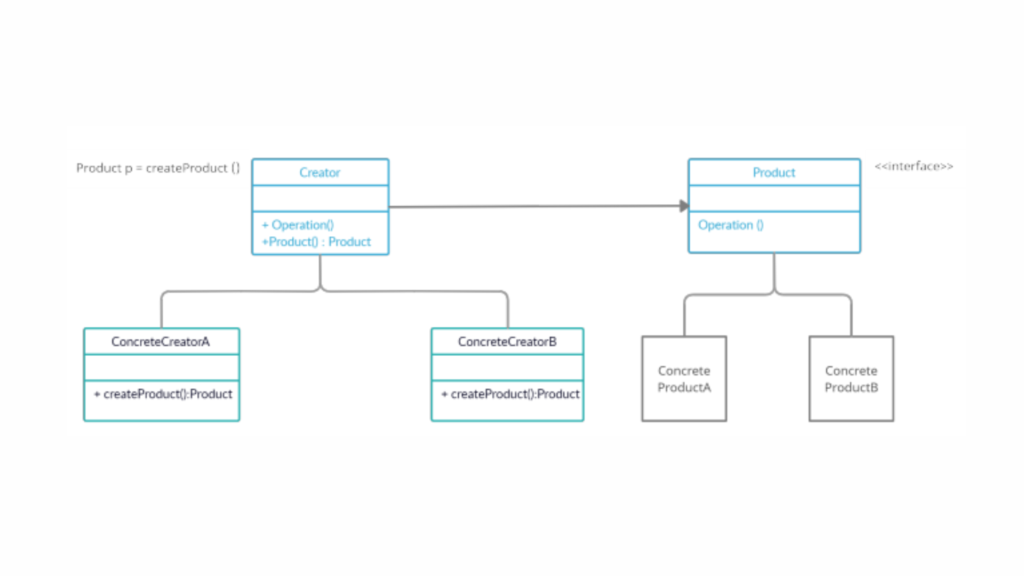
Structure of Factory Method Pattern
The Factory Method pattern can be broken down into the following components:
- Product: An interface or abstract class that defines the common behavior for the objects created by the factory method.
- ConcreteProduct: A class that implements the Product interface.
- Creator: An abstract class or interface that declares the factory method. This class may also provide some default implementation of the factory method that returns a default product.
- ConcreteCreator: A subclass of Creator that overrides the factory method to return an instance of a ConcreteProduct.
Inshort,
- Product: The common interface.
- Concrete Products: Different versions of the Product.
- Creator: Defines the factory method.
- Concrete Creators: Override the factory method to create specific products.
When to Use the Factory Method Pattern
The Factory Method pattern is useful in several situations. Here’s a brief overview; we will discuss detailed implementation soon:
- Unknown Object Dependencies:
- Situation: When you don’t know which specific objects you’ll need until runtime.
- Example: If you’re building an app that handles various types of documents, but you don’t know which document type you’ll need until the user chooses, the Factory Method helps by separating the document creation logic from the rest of your code. You can add new document types by creating new subclasses and updating the factory method.
- Extending Frameworks or Libraries:
- Situation: When you provide a framework or library that others will use and extend.
- Example: Suppose you’re providing a UI framework with square buttons. If someone needs round buttons, they can create a
RoundButton
subclass and configure the framework to use the new button type instead of the default square one.
- Reusing Existing Objects:
- Situation: When you want to reuse objects rather than creating new ones each time.
- Example: If creating a new object is resource-intensive, the Factory Method helps by reusing existing objects, which speeds up the process and saves system resources.
Implementation in Kotlin
Let’s dive into the implementation of the Factory Method pattern in Kotlin with some examples.
Basic Simple Implementation
Consider a scenario where we need to create different types of buttons in a GUI application.
// Step 1: Define the Product interface
interface Button {
fun render()
}
// Step 2: Implement ConcreteProduct classes
class WindowsButton : Button {
override fun render() {
println("Rendering Windows Button")
}
}
class MacButton : Button {
override fun render() {
println("Rendering Mac Button")
}
}
// Step 3: Define the Creator interface
abstract class Dialog {
abstract fun createButton(): Button
fun renderWindow() {
val button = createButton()
button.render()
}
}
// Step 4: Implement ConcreteCreator classes
class WindowsDialog : Dialog() {
override fun createButton(): Button {
return WindowsButton()
}
}
class MacDialog : Dialog() {
override fun createButton(): Button {
return MacButton()
}
}
// Client code
fun main() {
val dialog: Dialog = WindowsDialog()
dialog.renderWindow()
}
In this example:
- The
Button
interface defines the common behavior for all buttons. WindowsButton
andMacButton
are concrete implementations of theButton
interface.- The
Dialog
class defines the factory methodcreateButton()
, which is overridden byWindowsDialog
andMacDialog
to return the appropriate button type.
Advanced Implementation
In more complex scenarios, you might need to include additional logic in the factory method or handle multiple products. Let’s extend the example to include a Linux button and dynamically choose which dialog to create based on the operating system.
// Step 1: Add a new ConcreteProduct class
class LinuxButton : Button {
override fun render() {
println("Rendering Linux Button")
}
}
// Step 2: Add a new ConcreteCreator class
class LinuxDialog : Dialog() {
override fun createButton(): Button {
return LinuxButton()
}
}
// Client code with dynamic selection
fun main() {
val osName = System.getProperty("os.name").toLowerCase()
val dialog: Dialog = when {
osName.contains("win") -> WindowsDialog()
osName.contains("mac") -> MacDialog()
osName.contains("nix") || osName.contains("nux") -> LinuxDialog()
else -> throw UnsupportedOperationException("Unsupported OS")
}
dialog.renderWindow()
}
Here, we added support for Linux and dynamically selected the appropriate dialog based on the operating system. This approach showcases how the Factory Method pattern can be extended to handle more complex scenarios.
Real-World Examples
Factory method pattern for Payment App
Let’s imagine you have several payment methods like Credit Card, PayPal, and Bitcoin. Instead of hardcoding the creation of each payment processor in the app, you can use the Factory Method pattern to dynamically create the correct payment processor based on the user’s selection.
// Step 1: Define the Product interface
interface PaymentProcessor {
fun processPayment(amount: Double)
}
// Step 2: Implement ConcreteProduct classes
class CreditCardProcessor : PaymentProcessor {
override fun processPayment(amount: Double) {
println("Processing credit card payment of $$amount")
}
}
class PayPalProcessor : PaymentProcessor {
override fun processPayment(amount: Double) {
println("Processing PayPal payment of $$amount")
}
}
class BitcoinProcessor : PaymentProcessor {
override fun processPayment(amount: Double) {
println("Processing Bitcoin payment of $$amount")
}
}
// Step 3: Define the Creator abstract class
abstract class PaymentDialog {
abstract fun createProcessor(): PaymentProcessor
fun process(amount: Double) {
val processor = createProcessor()
processor.processPayment(amount)
}
}
// Step 4: Implement ConcreteCreator classes
class CreditCardDialog : PaymentDialog() {
override fun createProcessor(): PaymentProcessor {
return CreditCardProcessor()
}
}
class PayPalDialog : PaymentDialog() {
override fun createProcessor(): PaymentProcessor {
return PayPalProcessor()
}
}
class BitcoinDialog : PaymentDialog() {
override fun createProcessor(): PaymentProcessor {
return BitcoinProcessor()
}
}
// Client code
fun main() {
val paymentType = "PayPal"
val dialog: PaymentDialog = when (paymentType) {
"CreditCard" -> CreditCardDialog()
"PayPal" -> PayPalDialog()
"Bitcoin" -> BitcoinDialog()
else -> throw UnsupportedOperationException("Unsupported payment type")
}
dialog.process(250.0)
}
Here, we defined a PaymentProcessor
interface with three concrete implementations: CreditCardProcessor
, PayPalProcessor
, and BitcoinProcessor
. The client can select the payment type, and the appropriate payment processor is created using the Factory Method.
Factory method pattern for Document App
Imagine you are building an application that processes different types of documents (e.g., PDFs, Word Documents, and Text Files). You want to provide a way to open these documents without hard-coding the types.
// 1. Define the Product interface
interface Document {
fun open(): String
}
// 2. Implement ConcreteProducts
class PdfDocument : Document {
override fun open(): String {
return "Opening PDF Document"
}
}
class WordDocument : Document {
override fun open(): String {
return "Opening Word Document"
}
}
class TextDocument : Document {
override fun open(): String {
return "Opening Text Document"
}
}
// 3. Define the Creator class
abstract class DocumentFactory {
abstract fun createDocument(): Document
fun openDocument(): String {
val document = createDocument()
return document.open()
}
}
// 4. Implement ConcreteCreators
class PdfDocumentFactory : DocumentFactory() {
override fun createDocument(): Document {
return PdfDocument()
}
}
class WordDocumentFactory : DocumentFactory() {
override fun createDocument(): Document {
return WordDocument()
}
}
class TextDocumentFactory : DocumentFactory() {
override fun createDocument(): Document {
return TextDocument()
}
}
// Usage
fun main() {
val pdfFactory: DocumentFactory = PdfDocumentFactory()
println(pdfFactory.openDocument()) // Output: Opening PDF Document
val wordFactory: DocumentFactory = WordDocumentFactory()
println(wordFactory.openDocument()) // Output: Opening Word Document
val textFactory: DocumentFactory = TextDocumentFactory()
println(textFactory.openDocument()) // Output: Opening Text Document
}
Here,
Product Interface (Document): This is the interface that all concrete products (e.g., PdfDocument
, WordDocument
, and TextDocument
) implement. It ensures that all documents have the open()
method.
Concrete Products (PdfDocument, WordDocument, TextDocument): These classes implement the Document
interface. Each class provides its own implementation of the open()
method, specific to the type of document.
Creator (DocumentFactory): This is an abstract class that declares the factory method createDocument()
. The openDocument()
method relies on this factory method to obtain a document and then calls the open()
method on it.
Concrete Creators (PdfDocumentFactory, WordDocumentFactory, TextDocumentFactory): These classes extend the DocumentFactory
class and override the createDocument()
method to return a specific type of document.
Factory Method Pattern in Android Development
In Android development, the Factory Method Pattern is commonly used in many scenarios where object creation is complex or dependent on external factors like user input, configuration, or platform-specific implementations. Here are some examples:
ViewModelProvider in MVVM Architecture
When working with ViewModel
s in Android’s MVVM architecture, you often use the Factory Method Pattern to create instances of ViewModel
.
class ResumeSenderViewModelFactory(private val repository: ResumeSenderRepository) : ViewModelProvider.Factory {
override fun <T : ViewModel?> create(modelClass: Class<T>): T {
if (modelClass.isAssignableFrom(ResumeSenderViewModel::class.java)) {
return ResumeSenderViewModel(repository) as T
}
throw IllegalArgumentException("Unknown ViewModel class")
}
}
This factory method is responsible for creating ViewModel
instances and passing in necessary dependencies like the repository
.
Let’s quickly look at a few more.
Dependency Injection
interface DependencyFactory {
fun createNetworkClient(): NetworkClient
fun createDatabase(): Database
}
class ProductionDependencyFactory : DependencyFactory {
override fun createNetworkClient(): NetworkClient {
return Retrofit.Builder()
.baseUrl("https://api.softaai.com")
.build()
.create(ApiService::class.java)
}
override fun createDatabase(): Database {
return Room.databaseBuilder(
context,
AppDatabase::class.java,
"softaai_database"
).build()
}
}
class TestingDependencyFactory : DependencyFactory {
override fun createNetworkClient(): NetworkClient {
return MockNetworkClient()
}
override fun createDatabase(): Database {
return InMemoryDatabaseBuilder(context, AppDatabase::class.java)
.build()
}
}
Themeing and Styling
interface ThemeFactory {
fun createTheme(): Theme
}
class LightThemeFactory : ThemeFactory {
override fun createTheme(): Theme {
return Theme(R.style.AppThemeLight)
}
}
class DarkThemeFactory : ThemeFactory {
override fun createTheme(): Theme {
return Theme(R.style.AppThemeDark)
}
}
Data Source Management
interface DataSourceFactory {
fun createDataSource(): DataSource
}
class LocalDataSourceFactory : DataSourceFactory {
override fun createDataSource(): DataSource {
return LocalDataSource(database)
}
}
class RemoteDataSourceFactory : DataSourceFactory {
override fun createDataSource(): DataSource {
return RemoteDataSource(networkClient)
}
}
Image Loading
interface ImageLoaderFactory {
fun createImageLoader(): ImageLoader
}
class GlideImageLoaderFactory : ImageLoaderFactory {
override fun createImageLoader(): ImageLoader {
return Glide.with(context).build()
}
}
class PicassoImageLoaderFactory : ImageLoaderFactory {
override fun createImageLoader(): ImageLoader {
return Picasso.with(context).build()
}
}
Benefits of the Factory Method Pattern
Flexibility: The Factory Method pattern provides flexibility in object creation, allowing subclasses to choose the type of object to instantiate.
Decoupling: It decouples the client code from the object creation code, making the system more modular and easier to maintain. Through this, we achieve the Single Responsibility Principle.
Scalability: Adding new products to the system is straightforward and doesn’t require modifying existing code. Through this, we achieve the Open/Closed Principle.
Drawbacks of the Factory Method Pattern
Complexity: The Factory Method pattern can introduce additional complexity to the codebase, especially when dealing with simple object creation scenarios.
Overhead: It might lead to unnecessary subclassing and increased code size if not used appropriately.

Abstract Factory Design Pattern
Design patterns are key to solving recurring software design challenges. The Abstract Factory pattern, a creational design pattern, offers an interface to create related objects without specifying their concrete classes. It’s particularly helpful when your system needs to support various product types with shared traits but different implementations.
Here, in this section, we’ll explore the Abstract Factory pattern, its benefits, and how to implement it in Kotlin.
What is Abstract Factory Pattern?
We will look at the Abstract Factory Pattern in detail, but before that, let’s first understand one core concept: the ‘object family.
Object family
An “object family” refers to a group of related or dependent objects that are designed to work together. In the context of software design, particularly in design patterns like the Abstract Factory, an object family is a set of products that are designed to interact or collaborate with each other. Each product in this family shares a common theme, behavior, or purpose, making sure they can work seamlessly together without compatibility issues.
For example, if you’re designing a UI theme for a mobile app, you might have an object family that includes buttons, text fields, and dropdowns that all conform to a particular style (like “dark mode” or “light mode”). These objects are designed to be used together to prevent mismatching styles or interactions.
In software, preventing mismatches is crucial because inconsistencies between objects can cause bugs, user confusion, or functionality breakdowns. Design patterns like Abstract Factory help ensure that mismatched objects don’t interact, preventing unwanted behavior and making sure that all components belong to the same family.
Abstract Factory Pattern
The Abstract Factory pattern operates at a higher level of abstraction compared to the Factory Method pattern. Let me break this down in simple terms:
- Factory Method pattern: It provides an interface for creating an object but allows subclasses to alter the type of objects that will be created. In other words, it returns one of several possible sub-classes (or concrete products). You have a single factory that produces specific instances of a class, based on some logic or criteria.
- Abstract Factory pattern: It goes one step higher. Instead of just returning one concrete product, it returns a whole factory (a set of related factories). These factories, in turn, are responsible for producing families of related objects. In other words, the Abstract Factory itself creates factories (or “creators”) that will eventually return specific sub-classes or concrete products.
So, the definition is:
The Abstract Factory Pattern defines an interface or abstract class for creating families of related (or dependent) objects without specifying their concrete subclasses. This means that an abstract factory allows a class to return a factory of classes. Consequently, the Abstract Factory Pattern operates at a higher level of abstraction than the Factory Method Pattern. The Abstract Factory Pattern is also known as a “kit.”
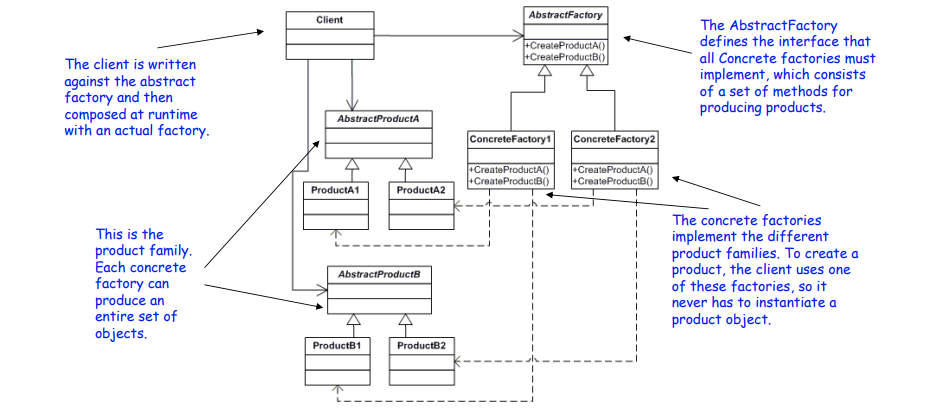
Structure of Abstract Factory Design Pattern
Abstract Factory:
- Defines methods for creating abstract products.
- Acts as an interface that declares methods for creating each type of product.
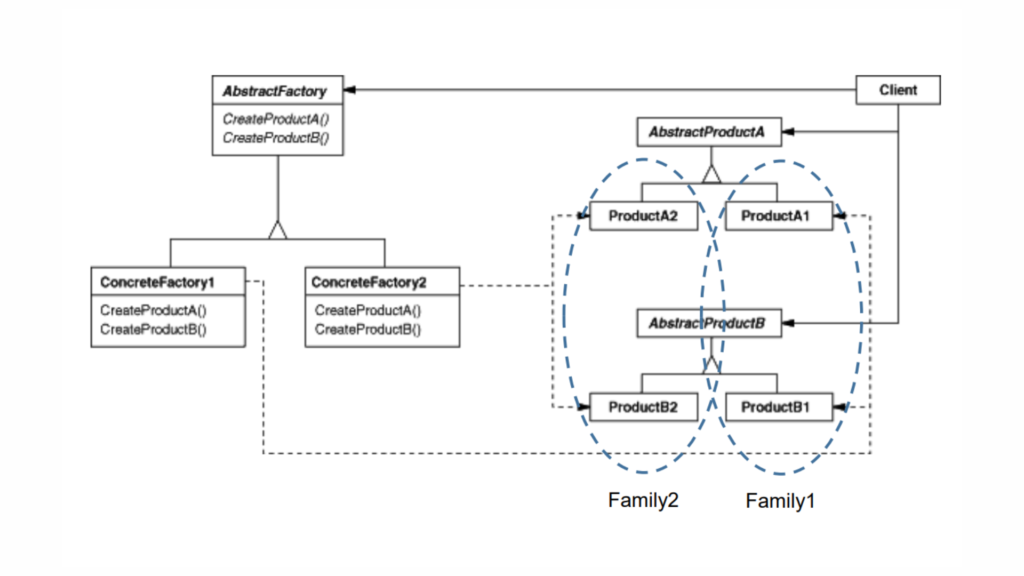
Concrete Factory:
- Implements the Abstract Factory methods to create concrete products.
- Each Concrete Factory is responsible for creating products that belong to a specific family or theme.
Abstract Product:
- Defines an interface or abstract class for a type of product object.
- This could be a generalization of the product that the factory will create.
Concrete Product:
- Implements the Abstract Product interface.
- Represents specific instances of the products that the factory will create.
Client:
- Uses the Abstract Factory and Abstract Product interfaces to work with the products.
- The client interacts with the factories through the abstract interfaces, so it does not need to know about the specific classes of the products it is working with.
Step-by-Step Walkthrough: Implementing the Abstract Factory in Kotlin
Let’s assume we’re working with a UI theme system where we have families of related components, such as buttons and checkboxes. These components can be styled differently based on a Light Theme or a Dark Theme.
Now, let’s implement a GUI theme system with DarkTheme and LightTheme using the Abstract Factory pattern.
Step 1: Define the Abstract Products
First, we’ll define interfaces for products, i.e., buttons and checkboxes, which can have different implementations for each theme.
// Abstract product: Button
interface Button {
fun paint()
}
// Abstract product: Checkbox
interface Checkbox {
fun paint()
}
These are abstract products that define the behaviors common to all buttons and checkboxes, regardless of the theme.
Step 2: Create Concrete Products
Next, we create concrete implementations for the DarkTheme and LightTheme variations of buttons and checkboxes.
// Concrete product for DarkTheme: DarkButton
class DarkButton : Button {
override fun paint() {
println("Rendering Dark Button")
}
}
// Concrete product for DarkTheme: DarkCheckbox
class DarkCheckbox : Checkbox {
override fun paint() {
println("Rendering Dark Checkbox")
}
}
// Concrete product for LightTheme: LightButton
class LightButton : Button {
override fun paint() {
println("Rendering Light Button")
}
}
// Concrete product for LightTheme: LightCheckbox
class LightCheckbox : Checkbox {
override fun paint() {
println("Rendering Light Checkbox")
}
}
Each product conforms to its respective interface while providing theme-specific rendering logic.
Step 3: Define Abstract Factory Interface
Now, we define the abstract factory that will create families of related objects (buttons and checkboxes).
// Abstract factory interface
interface GUIFactory {
fun createButton(): Button
fun createCheckbox(): Checkbox
}
This factory is responsible for creating theme-consistent products without knowing their concrete implementations.
Step 4: Create Concrete Factories
We now define two concrete factories that implement the GUIFactory interface for DarkTheme and LightTheme.
// Concrete factory for DarkTheme
class DarkThemeFactory : GUIFactory {
override fun createButton(): Button {
return DarkButton()
}
override fun createCheckbox(): Checkbox {
return DarkCheckbox()
}
}
// Concrete factory for LightTheme
class LightThemeFactory : GUIFactory {
override fun createButton(): Button {
return LightButton()
}
override fun createCheckbox(): Checkbox {
return LightCheckbox()
}
}
Each concrete factory creates products that belong to a specific theme (dark or light).
Step 5: Client Code
The client is agnostic about the theme being used. It interacts with the abstract factory to create theme-consistent buttons and checkboxes.
// Client code
class Application(private val factory: GUIFactory) {
fun render() {
val button = factory.createButton()
val checkbox = factory.createCheckbox()
button.paint()
checkbox.paint()
}
}
fun main() {
// Client is configured with a concrete factory
val darkFactory: GUIFactory = DarkThemeFactory()
val app1 = Application(darkFactory)
app1.render()
val lightFactory: GUIFactory = LightThemeFactory()
val app2 = Application(lightFactory)
app2.render()
}
//Output
Rendering Dark Button
Rendering Dark Checkbox
Rendering Light Button
Rendering Light Checkbox
Here, in this code:
- The client,
Application
, is initialized with a factory, eitherDarkThemeFactory
orLightThemeFactory
. - Based on the factory, it creates and renders theme-consistent buttons and checkboxes.
Real-World Examples
Suppose we have different types of banks, like a Retail Bank and a Corporate Bank. Each bank offers different types of accounts and loans:
- Retail Bank offers Savings Accounts and Personal Loans.
- Corporate Bank offers Business Accounts and Corporate Loans.
We want to create a system where the client (e.g., a bank application) can interact with these products without needing to know the specific classes that implement them.
Here, we’ll use the Abstract Factory Pattern to create families of related objects: bank accounts and loan products.
Implementation
Abstract Products
// Abstract Product for Accounts
interface Account {
fun getAccountType(): String
}
// Abstract Product for Loans
interface Loan {
fun getLoanType(): String
}
Concrete Products
// Concrete Product for Retail Bank Savings Account
class RetailSavingsAccount : Account {
override fun getAccountType(): String {
return "Retail Savings Account"
}
}
// Concrete Product for Retail Bank Personal Loan
class RetailPersonalLoan : Loan {
override fun getLoanType(): String {
return "Retail Personal Loan"
}
}
// Concrete Product for Corporate Bank Business Account
class CorporateBusinessAccount : Account {
override fun getAccountType(): String {
return "Corporate Business Account"
}
}
// Concrete Product for Corporate Bank Corporate Loan
class CorporateLoan : Loan {
override fun getLoanType(): String {
return "Corporate Loan"
}
}
Abstract Factory
// Abstract Factory for creating Accounts and Loans
interface BankFactory {
fun createAccount(): Account
fun createLoan(): Loan
}
Concrete Factories
// Concrete Factory for Retail Bank
class RetailBankFactory : BankFactory {
override fun createAccount(): Account {
return RetailSavingsAccount()
}
override fun createLoan(): Loan {
return RetailPersonalLoan()
}
}
// Concrete Factory for Corporate Bank
class CorporateBankFactory : BankFactory {
override fun createAccount(): Account {
return CorporateBusinessAccount()
}
override fun createLoan(): Loan {
return CorporateLoan()
}
}
Client
fun main() {
// Client code that uses the abstract factory
val retailFactory: BankFactory = RetailBankFactory()
val corporateFactory: BankFactory = CorporateBankFactory()
val retailAccount: Account = retailFactory.createAccount()
val retailLoan: Loan = retailFactory.createLoan()
val corporateAccount: Account = corporateFactory.createAccount()
val corporateLoan: Loan = corporateFactory.createLoan()
println("Retail Bank Account: ${retailAccount.getAccountType()}")
println("Retail Bank Loan: ${retailLoan.getLoanType()}")
println("Corporate Bank Account: ${corporateAccount.getAccountType()}")
println("Corporate Bank Loan: ${corporateLoan.getLoanType()}")
}
//Output
Retail Bank Account: Retail Savings Account
Retail Bank Loan: Retail Personal Loan
Corporate Bank Account: Corporate Business Account
Corporate Bank Loan: Corporate Loan
Here,
- Abstract Products (
Account
andLoan
): Define the interfaces for the products. - Concrete Products: Implement these interfaces with specific types of accounts and loans for different banks.
- Abstract Factory (
BankFactory
): Provides methods to create abstract products. - Concrete Factories (
RetailBankFactory
,CorporateBankFactory
): Implement the factory methods to create concrete products. - Client: Uses the factory to obtain the products and interact with them, without knowing their specific types.
This setup allows the client to work with different types of banks and their associated products without being tightly coupled to the specific classes that implement them.
Let’s see one more, suppose you are creating a general-purpose gaming environment and want to support different types of games. Player objects interact with Obstacle objects, but the types of players and obstacles vary depending on the game you are playing. You determine the type of game by selecting a particular GameElementFactory, and then the GameEnvironment manages the setup and play of the game.
Implementation
Abstract Products
// Abstract Product for Obstacle
interface Obstacle {
fun action()
}
// Abstract Product for Player
interface Player {
fun interactWith(obstacle: Obstacle)
}
Concrete Products
// Concrete Product for Player: Kitty
class Kitty : Player {
override fun interactWith(obstacle: Obstacle) {
print("Kitty has encountered a ")
obstacle.action()
}
}
// Concrete Product for Player: KungFuGuy
class KungFuGuy : Player {
override fun interactWith(obstacle: Obstacle) {
print("KungFuGuy now battles a ")
obstacle.action()
}
}
// Concrete Product for Obstacle: Puzzle
class Puzzle : Obstacle {
override fun action() {
println("Puzzle")
}
}
// Concrete Product for Obstacle: NastyWeapon
class NastyWeapon : Obstacle {
override fun action() {
println("NastyWeapon")
}
}
Abstract Factory
// Abstract Factory
interface GameElementFactory {
fun makePlayer(): Player
fun makeObstacle(): Obstacle
}
Concrete Factories
// Concrete Factory: KittiesAndPuzzles
class KittiesAndPuzzles : GameElementFactory {
override fun makePlayer(): Player {
return Kitty()
}
override fun makeObstacle(): Obstacle {
return Puzzle()
}
}
// Concrete Factory: KillAndDismember
class KillAndDismember : GameElementFactory {
override fun makePlayer(): Player {
return KungFuGuy()
}
override fun makeObstacle(): Obstacle {
return NastyWeapon()
}
}
Game Environment
// Game Environment
class GameEnvironment(private val factory: GameElementFactory) {
private val player: Player = factory.makePlayer()
private val obstacle: Obstacle = factory.makeObstacle()
fun play() {
player.interactWith(obstacle)
}
}
Main Function
fun main() {
// Creating game environments with different factories
val kittiesAndPuzzlesFactory: GameElementFactory = KittiesAndPuzzles()
val killAndDismemberFactory: GameElementFactory = KillAndDismember()
val game1 = GameEnvironment(kittiesAndPuzzlesFactory)
val game2 = GameEnvironment(killAndDismemberFactory)
println("Game 1:")
game1.play() // Output: Kitty has encountered a Puzzle
println("Game 2:")
game2.play() // Output: KungFuGuy now battles a NastyWeapon
}
Here,
Abstract Products:
Obstacle
andPlayer
are interfaces that define the methods for different game elements.
Concrete Products:
Kitty
andKungFuGuy
are specific types of players.Puzzle
andNastyWeapon
are specific types of obstacles.
Abstract Factory:
GameElementFactory
defines the methods for creatingPlayer
andObstacle
.
Concrete Factories:
KittiesAndPuzzles
creates aKitty
player and aPuzzle
obstacle.KillAndDismember
creates aKungFuGuy
player and aNastyWeapon
obstacle.
Game Environment:
GameEnvironment
uses the factory to create and interact with game elements.
Main Function:
- Demonstrates how different game environments (factories) produce different combinations of players and obstacles.
This design allows for a flexible gaming environment where different types of players and obstacles can be easily swapped in and out based on the chosen factory, demonstrating the power of the Abstract Factory Pattern in managing families of related objects.
Abstract Factory Pattern in Android Development
When using a Dependency Injection framework, you might use the Abstract Factory pattern to provide different implementations of dependencies based on runtime conditions.
// Abstract Product
interface NetworkClient {
fun makeRequest(url: String): String
}
// Concrete Products
class HttpNetworkClient : NetworkClient {
override fun makeRequest(url: String): String = "HTTP Request to $url"
}
class HttpsNetworkClient : NetworkClient {
override fun makeRequest(url: String): String = "HTTPS Request to $url"
}
// Abstract Factory
interface NetworkClientFactory {
fun createClient(): NetworkClient
}
//Concrete Factories
class HttpClientFactory : NetworkClientFactory {
override fun createClient(): NetworkClient = HttpNetworkClient()
}
class HttpsClientFactory : NetworkClientFactory {
override fun createClient(): NetworkClient = HttpsNetworkClient()
}
//Client code
fun main() {
val factory: NetworkClientFactory = HttpsClientFactory() // or HttpClientFactory()
val client: NetworkClient = factory.createClient()
println(client.makeRequest("softaai.com")) // Output: HTTPS Request to softaai.com or HTTP Request to softaai.com
}
When to Use Abstract Factory?
A system must be independent of how its products are created: This means you want to decouple the creation logic from the actual usage of objects. The system will use abstract interfaces, and the concrete classes that create the objects will be hidden from the user, promoting flexibility.
A system should be configured with one of multiple families of products: If your system needs to support different product variants that are grouped into families (like different UI components for MacOS, Windows, or Linux), Abstract Factory allows you to switch between these families seamlessly without changing the underlying code.
A family of related objects must be used together: Often, products in a family are designed to work together, and mixing objects from different families could cause problems. Abstract Factory ensures that related objects (like buttons, windows, or icons in a GUI) come from the same family, preserving compatibility.
You want to reveal only interfaces of a family of products and not their implementations: This approach hides the actual implementation details, exposing only the interface. By doing so, you make the system easier to extend and maintain, as any changes to the product families won’t affect client code directly.
Abstract Factory vs Factory Method
The Factory Method pattern provides a way to create a single product, while the Abstract Factory creates families of related products. If you only need to create one type of object, the Factory Method might be sufficient. However, if you need to handle multiple related objects (like in our theme example), the Abstract Factory is more suitable.
Advantages of Abstract Factory
- Isolation of Concrete Classes: The client interacts with factory interfaces, making it independent of concrete class implementations.
- Consistency Among Products: The factory ensures that products from the same family are used together, preventing inconsistent states.
- Scalability: Adding new families (themes) of products is straightforward. You only need to introduce new factories and product variants without affecting existing code.
Disadvantages of Abstract Factory
- Complexity: As more product families and variations are introduced, the number of classes can grow substantially, leading to more maintenance complexity.
- Rigid Structure: If new types of products are required that don’t fit the existing family structure, refactoring may be needed.

Builder Design Pattern
Creating objects with multiple parameters can get complicated, especially when some are optional or require validation. The Builder Design Pattern simplifies this by offering a flexible, structured way to construct complex objects.
Here, in this section, we’ll break down the Builder Design Pattern in Kotlin, exploring how it works, why it’s useful, and how to apply it effectively. By the end, you’ll be ready to use the Builder pattern in your Kotlin projects.
What is the Builder Design Pattern?
Some objects are complex and need to be built step-by-step (think of objects with multiple fields or components). Instead of having a single constructor that takes in many arguments (which can get confusing), the Builder pattern provides a way to build an object step-by-step. By using this approach, we can have multiple different ways to build (or “represent”) the object, but still follow the same process of construction
In simple terms, the Builder Design Pattern is like ordering a burger at a fancy burger joint. You don’t just ask for “a burger” (unless you enjoy living dangerously); instead, you customize it step by step. First, you pick your bun, then your patty, cheese, sauces, toppings—you get the idea. By the time you’re done, you’ve built your perfect burger 🍔.
Similarly, in software development, when you want to create an object, instead of passing every possible parameter into a constructor (which can be messy and error-prone), you build the object step by step in a clean and readable manner. The Builder Design Pattern helps you construct complex objects without losing your sanity.
Let’s take one more real-world example with a Car
class. First, we’ll see the scenario without the Builder Pattern (also known as the Constructor Overload Nightmare).
class Car(val make: String, val model: String, val color: String, val transmission: String, val hasSunroof: Boolean, val hasBluetooth: Boolean, val hasHeatedSeats: Boolean)
Ugh, look at that. My eyes hurt just reading it. 🥲 Now, let’s fix this using the Builder Pattern (Don’t worry about the structure; we’ll look at it soon):
class Car private constructor(
val make: String?,
val model: String?,
val color: String?,
val transmission: String?,
val hasSunroof: Boolean,
val hasBluetooth: Boolean,
val hasHeatedSeats: Boolean
) {
// Builder Class Nested Inside Car Class
class Builder {
private var make: String? = null
private var model: String? = null
private var color: String? = null
private var transmission: String? = null
private var hasSunroof: Boolean = false
private var hasBluetooth: Boolean = false
private var hasHeatedSeats: Boolean = false
fun make(make: String) = apply { this.make = make }
fun model(model: String) = apply { this.model = model }
fun color(color: String) = apply { this.color = color }
fun transmission(transmission: String) = apply { this.transmission = transmission }
fun hasSunroof(hasSunroof: Boolean) = apply { this.hasSunroof = hasSunroof }
fun hasBluetooth(hasBluetooth: Boolean) = apply { this.hasBluetooth = hasBluetooth }
fun hasHeatedSeats(hasHeatedSeats: Boolean) = apply { this.hasHeatedSeats = hasHeatedSeats }
fun build(): Car {
return Car(make, model, color, transmission, hasSunroof, hasBluetooth, hasHeatedSeats)
}
}
}
Usage:
val myCar = Car.Builder()
.make("Tesla")
.model("Model S")
.color("Midnight Silver")
.hasBluetooth(true)
.hasSunroof(true)
.build()
println("I just built a car: ${myCar.make} ${myCar.model}, in ${myCar.color}, with Bluetooth: ${myCar.hasBluetooth}")
Boom! 💥 You’ve just built a car step by step, specifying only the parameters you need without cramming everything into one big constructor. Isn’t that a lot cleaner?
Technical Definition:
The Builder Pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations.
The Builder Design Pattern allows you to construct objects step by step, without needing to pass a hundred parameters into the constructor. It also lets you create different versions of the same object, using the same construction process. In simple terms, it separates object construction from its representation, making it more flexible and manageable.
For example, think of building a house. You have several steps: laying the foundation, building the walls, adding the roof, etc. If you change how each of these steps is done (e.g., using wood or brick for the walls), you end up with different kinds of houses. Similarly, in programming, different implementations of each step can lead to different final objects, even if the overall process is the same.
Structure of Builder Design Pattern
Here’s a breakdown of the structure of the Builder Design pattern:
- Product: This is the complex object that is being built. It might have several parts or features that need to be assembled. The
Product
class defines these features and provides methods to access or manipulate them. - Builder: This is an abstract interface or class that declares the construction steps necessary to create the
Product
. It often includes methods to set various parts of theProduct
. - ConcreteBuilder: This class implements the
Builder
interface and provides specific implementations of the construction steps. It keeps track of the current state of the product being built and assembles it step by step. Once the construction is complete, it returns the finalProduct
. - Director: The
Director
class is responsible for managing the construction process. It uses aBuilder
instance to construct the product. It controls the order of the construction steps, ensuring that the product is built in a consistent and valid way. - Client: The
Client
is responsible for initiating the construction process. It creates aDirector
and aConcreteBuilder
, and then uses theDirector
to construct theProduct
through theConcreteBuilder
.
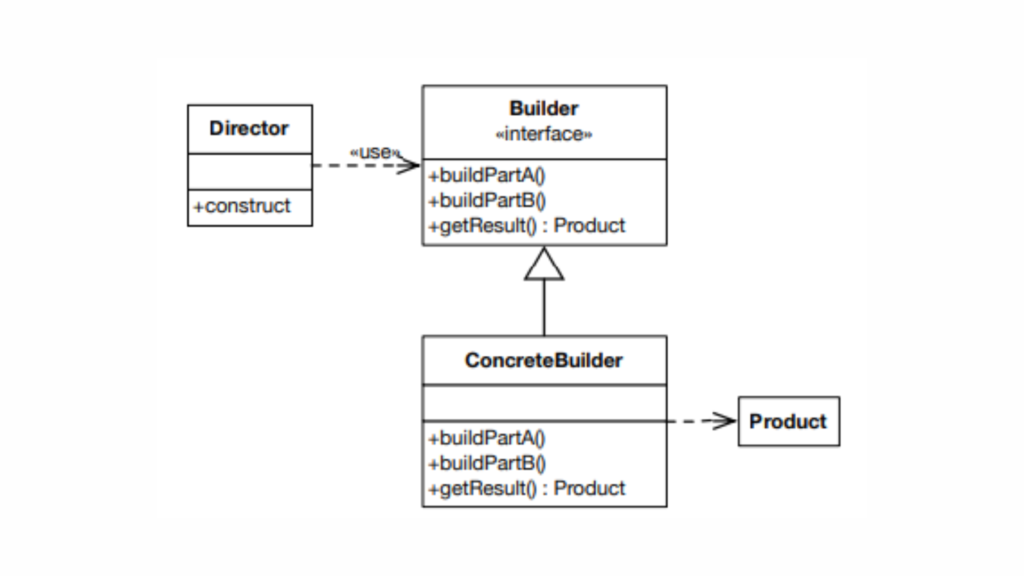
Let’s break down each component:
Builder (Interface)
The Builder
interface (or abstract class) defines the methods for creating different parts of the Product
. It typically includes methods like buildPartA()
, buildPartB()
, etc., and a method to get the final Product
. Here’s a brief overview:
- Methods:
buildPartA()
: Defines how to build part A of theProduct
.buildPartB()
: Defines how to build part B of theProduct
.getResult()
: Returns the finalProduct
after construction.
ConcreteBuilder
The ConcreteBuilder
class implements the Builder
interface. It provides specific implementations for the construction steps and keeps track of the current state of the Product
. Once the construction is complete, it can return the constructed Product
.
- Methods:
buildPartA()
: Implements the logic to build part A of theProduct
.buildPartB()
: Implements the logic to build part B of theProduct
.getResult()
: Returns the constructedProduct
.
Director
The Director
class orchestrates the construction process. It uses a Builder
instance to construct the Product
step by step, controlling the order of the construction steps.
- Methods:
construct()
: Manages the sequence of construction steps using theBuilder
.- It might also call methods like
buildPartA()
andbuildPartB()
in a specific order.
Product
The Product
represents the complex object being built. It is assembled from various parts defined by the Builder
. It usually includes features or properties that were set during the building process.
Real-World Examples
Let’s say we want to build a House object. A house can be simple, luxury, or modern, with different features (like number of windows, rooms, etc.). Each of these houses requires similar steps during construction, but the outcome is different.
Key Components:
- Product: The object that is being built (House in this case).
- Builder Interface: Declares the steps to build different parts of the product.
- Concrete Builders: Implement the steps to build different versions of the product.
- Director: Controls the building process and calls the necessary steps in a sequence.
Kotlin Example: House Construction
// Product: The object that is being built
data class House(
var foundation: String = "",
var structure: String = "",
var roof: String = "",
var interior: String = ""
)
// Builder Interface: Declares the building steps
interface HouseBuilder {
fun buildFoundation()
fun buildStructure()
fun buildRoof()
fun buildInterior()
fun getHouse(): House
}
// Concrete Builder 1: Builds a luxury house
class LuxuryHouseBuilder : HouseBuilder {
private val house = House()
override fun buildFoundation() {
house.foundation = "Luxury Foundation with basement"
}
override fun buildStructure() {
house.structure = "Luxury Structure with high-quality materials"
}
override fun buildRoof() {
house.roof = "Luxury Roof with tiles"
}
override fun buildInterior() {
house.interior = "Luxury Interior with modern design"
}
override fun getHouse(): House {
return house
}
}
// Concrete Builder 2: Builds a simple house
class SimpleHouseBuilder : HouseBuilder {
private val house = House()
override fun buildFoundation() {
house.foundation = "Simple Foundation"
}
override fun buildStructure() {
house.structure = "Simple Structure with basic materials"
}
override fun buildRoof() {
house.roof = "Simple Roof with asphalt shingles"
}
override fun buildInterior() {
house.interior = "Simple Interior with basic design"
}
override fun getHouse(): House {
return house
}
}
// Director: Controls the building process
class Director(private val houseBuilder: HouseBuilder) {
fun constructHouse() {
houseBuilder.buildFoundation()
houseBuilder.buildStructure()
houseBuilder.buildRoof()
houseBuilder.buildInterior()
}
}
// Client: Using the builder pattern
fun main() {
// Construct a luxury house
val luxuryBuilder = LuxuryHouseBuilder()
val director = Director(luxuryBuilder)
director.constructHouse()
val luxuryHouse = luxuryBuilder.getHouse()
println("Luxury House: $luxuryHouse")
// Construct a simple house
val simpleBuilder = SimpleHouseBuilder()
val director2 = Director(simpleBuilder)
director2.constructHouse()
val simpleHouse = simpleBuilder.getHouse()
println("Simple House: $simpleHouse")
}
Here,
- House (Product): Represents the object being built, with attributes like foundation, structure, roof, and interior.
- HouseBuilder (Interface): Declares the steps required to build a house.
- LuxuryHouseBuilder and SimpleHouseBuilder (Concrete Builders): Provide different implementations of how to construct a luxury or simple house by following the same steps.
- Director: Orchestrates the process of building a house. It doesn’t know the details of construction but knows the sequence of steps.
- Client: Chooses which builder to use and then delegates the construction to the director.
Let’s revisit our initial real-world example of a Car
class. Let’s try to build it by following the proper structure of the Builder Design Pattern.
// Product
class Car(
val engine: String,
val wheels: Int,
val color: String
) {
override fun toString(): String {
return "Car(engine='$engine', wheels=$wheels, color='$color')"
}
}
// Builder Interface
interface CarBuilder {
fun buildEngine(engine: String): CarBuilder
fun buildWheels(wheels: Int): CarBuilder
fun buildColor(color: String): CarBuilder
fun getResult(): Car
}
// ConcreteBuilder
class ConcreteCarBuilder : CarBuilder {
private var engine: String = ""
private var wheels: Int = 0
private var color: String = ""
override fun buildEngine(engine: String): CarBuilder {
this.engine = engine
return this
}
override fun buildWheels(wheels: Int): CarBuilder {
this.wheels = wheels
return this
}
override fun buildColor(color: String): CarBuilder {
this.color = color
return this
}
override fun getResult(): Car {
return Car(engine, wheels, color)
}
}
// Director
class CarDirector(private val builder: CarBuilder) {
fun constructSportsCar() {
builder.buildEngine("V8")
.buildWheels(4)
.buildColor("Red")
}
fun constructFamilyCar() {
builder.buildEngine("V6")
.buildWheels(4)
.buildColor("Blue")
}
}
// Client
fun main() {
val builder = ConcreteCarBuilder()
val director = CarDirector(builder)
director.constructSportsCar()
val sportsCar = builder.getResult()
println(sportsCar)
director.constructFamilyCar()
val familyCar = builder.getResult()
println(familyCar)
}
// Output
//Car(engine='V8', wheels=4, color='Red')
//Car(engine='V6', wheels=4, color='Blue')
Here,
- Product:
Car
class represents the complex object with various parts. - Builder:
CarBuilder
interface defines methods to set different parts of theCar
. - ConcreteBuilder:
ConcreteCarBuilder
provides implementations for theCarBuilder
methods and assembles theCar
. - Director:
CarDirector
manages the construction process and defines specific configurations. - Client: The
main
function initiates the building process by creating aConcreteCarBuilder
and aCarDirector
, then constructs different types of cars.
Builder Design Pattern – Collaboration
In the Builder design pattern, the Director and Builder work together to create complex objects step by step. Here’s how their collaboration functions:
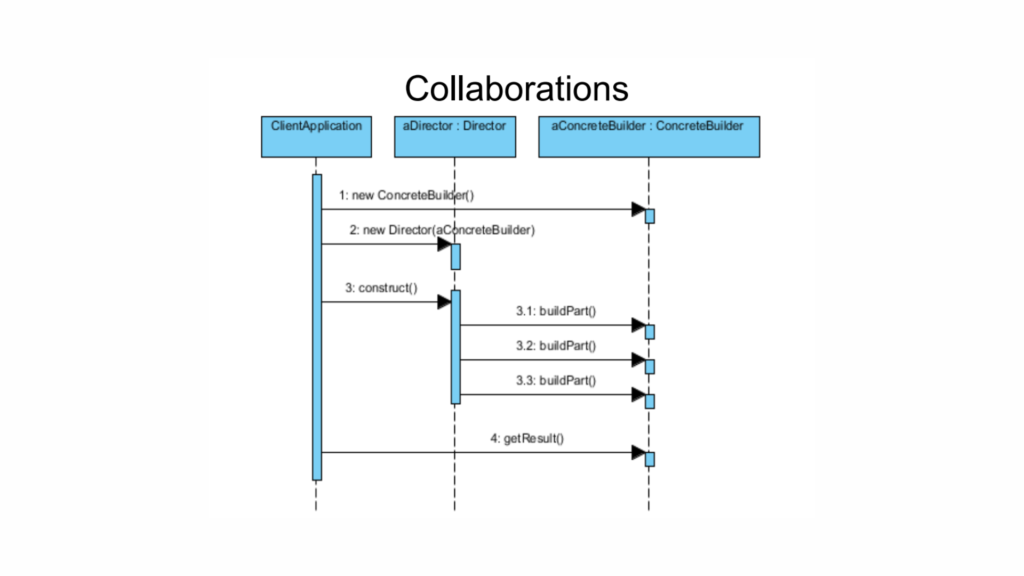
- Client Sets Up the Director and Builder:
- The client (main program) creates a Director and selects a specific Builder to do the construction work.
- Director Gives Instructions:
- The Director tells the Builder what part of the product to build, step by step.
- Builder Constructs the Product:
- The Builder follows the instructions from the Director and adds each part to the product as it’s told to.
- Client Gets the Finished Product:
- Once everything is built, the client gets the final product from the Builder.
Roles
- Director’s Role: Manages the process, knows the order in which the parts need to be created, but not the specifics of how the parts are built.
- Builder’s Role: Handles the construction details, assembling the product part by part as instructed by the Director.
- Client’s Role: Initiates the process, sets up the Director with the appropriate Builder, and retrieves the completed product.
Real-World Examples in Android
In Android, the Builder Design pattern is commonly used to construct objects that require multiple parameters or a specific setup order. A classic real-world example of this is building dialogs, such as AlertDialog
, or creating notifications using NotificationCompat.Builder
.
AlertDialog Builder
An AlertDialog in Android is a great example of the Builder pattern. It’s used to build a dialog step by step, providing a fluent API to add buttons, set the title, message, and other properties.
val alertDialog = AlertDialog.Builder(this)
.setTitle("Delete Confirmation")
.setMessage("Are you sure you want to delete this item?")
.setPositiveButton("Yes") { dialog, which ->
// Handle positive button click
}
.setNegativeButton("No") { dialog, which ->
dialog.dismiss()
}
.create()
alertDialog.show()
Here, the AlertDialog.Builder
is used to construct a complex dialog. Each method (setTitle
, setMessage
, setPositiveButton
) is called in a chained manner, and finally, create()
is called to generate the final AlertDialog
object.
Notification Builder Using NotificationCompat.Builder
Another common use of the Builder pattern in Android is when constructing notifications.
val notificationManager = getSystemService(Context.NOTIFICATION_SERVICE) as NotificationManager
// Create a notification channel for Android O and above
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
val channel = NotificationChannel("channel_id", "Channel Name", NotificationManager.IMPORTANCE_DEFAULT)
notificationManager.createNotificationChannel(channel)
}
val notification = NotificationCompat.Builder(this, "channel_id")
.setSmallIcon(R.drawable.ic_notification)
.setContentTitle("New Message")
.setContentText("You have a new message!")
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.build()
notificationManager.notify(1, notification)
Here, the NotificationCompat.Builder
allows you to create a notification step by step. You can set various attributes like the icon, title, text, and priority, and finally, call build()
to create the notification object.
Builder Design Pattern vs. Abstract Factory Pattern
Abstract Factory Pattern
- Purpose: It focuses on creating multiple related or dependent objects (often of a common family or theme) without specifying their exact classes.
- Object Creation Knowledge: The Abstract Factory knows ahead of time what objects it will create, and the configuration is usually predefined.
- Fixed Configuration: Once deployed, the configuration of the objects produced by the factory tends to remain fixed. The factory doesn’t change its set of products during runtime.
Builder Design Pattern
- Purpose: It focuses on constructing complex objects step by step, allowing more flexibility in the object creation process.
- Object Construction Knowledge: The Director (which orchestrates the Builder) knows how to construct the object but does so by using various Builders to manage different configurations.
- Dynamic Configuration: The Builder allows the configuration of the object to be modified during runtime, offering more flexibility. The specific configuration is chosen dynamically based on the concrete builder used during construction.
Key Differences
- Scope: Abstract Factory deals with families of related objects, while Builder constructs a single, complex object.
- Flexibility: Abstract Factory has a fixed set of products, while Builder allows step-by-step customization during runtime.
- Role of Director: In the Builder pattern, the Director oversees object construction, while the Abstract Factory does not rely on a director to manage creation steps.
In short, use Abstract Factory when you need to create families of objects, and use Builder when constructing a complex object in steps is more important.
Advantages of Builder Design Pattern
- Encapsulates Complex Construction:
The Builder pattern encapsulates the process of constructing complex objects, keeping the construction logic separate from the actual object logic. - Supports Multi-step Object Construction:
It allows objects to be built step-by-step, enabling greater flexibility in how an object is constructed, as opposed to a one-step factory approach. - Abstracts Internal Representation:
The internal details of the product being built are hidden from the client. The client interacts only with the builder, without worrying about the product’s internal structure. - Flexible Product Implementation:
The product implementations can be swapped without impacting the client code as long as they conform to the same abstract interface. This promotes maintainability and scalability.
Disadvantages of Builder Design Pattern
- Increased Code Complexity:
Implementing the Builder pattern can lead to more classes and additional boilerplate code, which may be overkill for simpler objects that don’t require complex construction. - Not Ideal for Simple Objects:
For objects that can be constructed in a straightforward manner, using a Builder pattern might be unnecessarily complex and less efficient compared to simple constructors or factory methods. - Can Lead to Large Number of Builder Methods:
As the complexity of the object grows, the number of builder methods can increase, which might make the Builder class harder to maintain or extend. - Potential for Code Duplication:
If the construction steps are similar across various products, there could be some code duplication, especially when multiple builders are required for related products.

Prototype Design Pattern
Design patterns may sometimes seem like fancy terms reserved for architects, but they solve real problems we face in coding. One such pattern is the Prototype Design Pattern. While the name might sound futuristic, don’t worry—we’re not cloning dinosaurs. Instead, we’re making exact copies of objects, complete with all their properties, without rebuilding them from scratch every time.
Imagine how convenient it would be to duplicate objects effortlessly, just like using a cloning feature in your favorite video game. 🎮 That’s exactly what the Prototype Design Pattern offers—a smart way to streamline object creation.
Here, we’ll explore the Prototype Pattern in Kotlin, break it down with easy-to-follow examples, and show you how to clone objects like a pro. Let’s dive in!
What is the Prototype Design Pattern?
Imagine you’re making an army of robots 🦾 for world domination. You have a base robot design, but each robot should have its unique characteristics (maybe different colors, weapons, or dance moves 💃). Creating every robot from scratch seems exhausting. What if you could just make a copy, tweak the details, and deploy? That’s the Prototype Design Pattern!
The Prototype Pattern allows you to create new objects by copying existing ones (called prototypes). This approach is super useful when object creation is costly, and you want to avoid all the drama of reinitializing or setting up.
TL;DR:
- Purpose: To avoid the cost of creating objects from scratch.
- How: By cloning existing objects.
- When: Use when object creation is expensive or when we want variations of an object with minor differences.
Since we’re diving into the world of object cloning, let’s first take a good look at how it works. Think of it as learning the basics of cloning before you start creating your own army of identical robots—just to keep things interesting!
Clonning & The Clone Wars ⚔️
The core concept in the Prototype Pattern is the Cloneable
interface. In many programming languages, including Java, objects that can be cloned implement this interface. The clone()
method typically provides the mechanism for creating a duplicate of an object.
The Cloneable
interface ensures that the class allows its objects to be cloned and defines the basic behavior for cloning. By default, this usually results in a shallow copy of the object.
Hold on! Before you start cloning like there’s no tomorrow, it’s essential to grasp the difference between shallow copies and deep copies, as they can significantly affect how your clones behave.
Shallow vs. Deep Copying
Shallow Copy: In a shallow clone, only the object itself is copied, but any references to other objects remain shared. For instance, if your object has a list or an array, only the reference to that list is copied, not the actual list elements. When we clone an object, we only copy the top-level fields. If the object contains references to other objects (like arrays or lists), those references are shared, not copied. It’s like making photocopies of a contract but using the same pen to sign all of them. Not cool.
Deep Copy: In contrast, deep cloning involves copying not just the object but also all objects that it references. All objects, including the nested ones, are fully cloned. In this case, each contract gets its own pen. Much cooler.
I’ve already written a detailed article on this topic. Please refer to it if you want to dive deeper and gain full control over the concept.
Structure of the Prototype Design Pattern
The Prototype Design Pattern consists of a few key components that work together to facilitate object cloning. Here’s a breakdown:
- Prototype Interface: This defines the
clone()
method, which is responsible for cloning objects. - Concrete Prototype: This class implements the
Prototype
interface and provides the actual logic for cloning itself. - Client: The client code interacts with the prototype to create clones of existing objects, avoiding the need to instantiate new objects from scratch.
In Kotlin, you can use the Cloneable
interface to implement the prototype pattern.
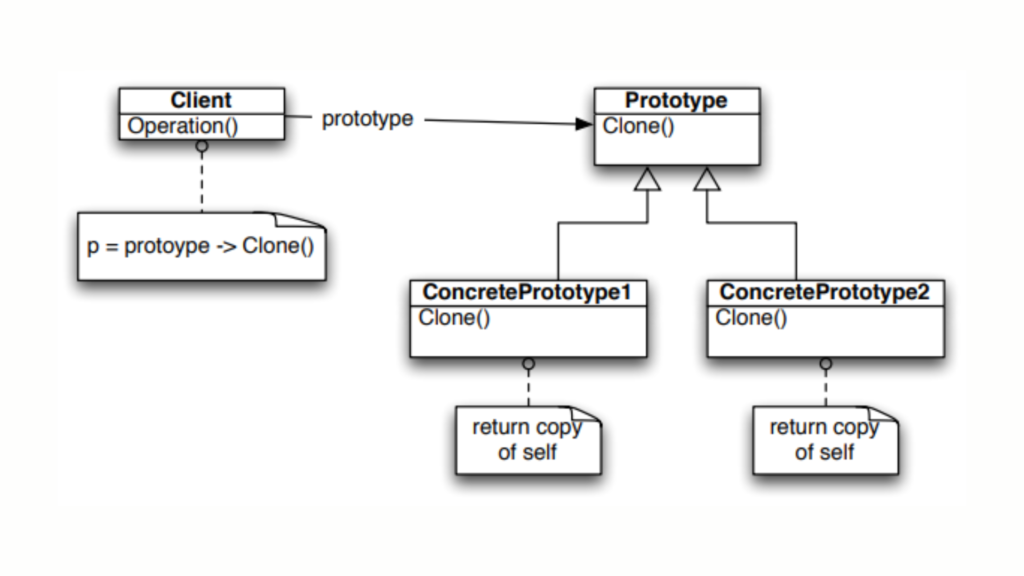
In this typical UML diagram for the Prototype Pattern, you would see the following components:
- Prototype (interface): Defines the contract for cloning.
- Concrete Prototype (class): Implements the clone method to copy itself.
- Client (class): Interacts with the prototype interface to get a cloned object.
How the Prototype Pattern Works
As we now know, the Prototype pattern consists of the following components:
- Prototype: This is an interface or abstract class that defines a method to clone objects.
- Concrete Prototype: These are the actual classes that implement the clone functionality. Each class is responsible for duplicating its instances.
- Client: The client class, which creates new objects by cloning prototypes rather than calling constructors.
In Kotlin, you can use the Cloneable
interface to implement the prototype pattern.
Implementing Prototype Pattern in Kotlin
Let’s go through a practical example of how to implement the Prototype Design Pattern in Kotlin.
Step 1: Define the Prototype Interface
Kotlin has a Cloneable
interface that indicates an object can be cloned, but the clone()
method is not defined in Cloneable
itself. Instead, you need to override the clone()
method from the Java Object
class in a class that implements Cloneable
.
Please note that you won’t see any explicit import statement when using Cloneable
and the clone()
method in Kotlin. This is because both Cloneable
and clone()
are part of the Java standard library, which is automatically available in Kotlin without requiring explicit imports.
interface Prototype : Cloneable {
public override fun clone(): Prototype
}
In the above code, we define the Prototype
interface and inherit the Cloneable
interface, which allows us to override the clone()
method.
Step 2: Create Concrete Prototypes
Now, let’s create concrete implementations of the Prototype. These classes will define the actual objects we want to clone.
data class Circle(var radius: Int, var color: String) : Prototype {
override fun clone(): Circle {
return Circle(this.radius, this.color)
}
fun draw() {
println("Drawing Circle with radius $radius and color $color")
}
}
data class Rectangle(var width: Int, var height: Int, var color: String) : Prototype {
override fun clone(): Rectangle {
return Rectangle(this.width, this.height, this.color)
}
fun draw() {
println("Drawing Rectangle with width $width, height $height, and color $color")
}
}
Here, we have two concrete classes, Circle
and Rectangle
. Both classes implement the Prototype
interface and override the clone()
method to return a copy of themselves.
Circle
has propertiesradius
andcolor
.Rectangle
has propertieswidth
,height
, andcolor
.
Each class has a draw()
method for demonstration purposes to show the state of the object.
Step 3: Using the Prototype Pattern
Now that we have our prototype objects (Circle
and Rectangle
), we can clone them to create new objects.
fun main() {
// Create an initial circle prototype
val circle1 = Circle(5, "Red")
circle1.draw() // Output: Drawing Circle with radius 5 and color Red
// Clone the circle to create a new circle
val circle2 = circle1.clone()
circle2.color = "Blue" // Change the color of the cloned circle
circle2.draw() // Output: Drawing Circle with radius 5 and color Blue
// Create an initial rectangle prototype
val rectangle1 = Rectangle(10, 20, "Green")
rectangle1.draw() // Output: Drawing Rectangle with width 10, height 20, and color Green
// Clone the rectangle and modify its width
val rectangle2 = rectangle1.clone()
rectangle2.width = 15
rectangle2.draw() // Output: Drawing Rectangle with width 15, height 20, and color Green
}
Explanation:
Creating a Prototype (circle1
): We create a Circle
object with a radius of 5 and color "Red"
.
Cloning the Prototype (circle2
): Instead of creating another circle object from scratch, we clone circle1
using the clone()
method. We change the color of the cloned circle to "Blue"
to show that it is a different object from the original one.
Creating a Rectangle Prototype: Similarly, we create a Rectangle
object with a width of 10, height of 20, and color "Green"
.
Cloning the Rectangle (rectangle2
): We then clone the rectangle and modify the width of the cloned object.
Why Use Prototype?
You might be wondering, “Why not just create new objects every time?” Here are a few good reasons:
- Efficiency: Some objects are expensive to create. Think of database records or UI elements with lots of configurations. Cloning is faster than rebuilding.
- Avoid Complexity: If creating an object involves many steps (like baking a cake), cloning helps you avoid repeating those steps.
- Customization: You can create a base object and clone it multiple times, tweaking each clone to suit your needs (like adding more chocolate chips to a clone of a cake).
How the pattern works in Kotlin in a more efficient and readable way
Kotlin makes the implementation of the Prototype Pattern easy and concise with its support for data classes and the copy()
function. The copy function can create new instances of objects with the option to modify fields during copying.
Here’s a basic structure of the Prototype Pattern in Kotlin:
interface Prototype : Cloneable {
fun clone(): Prototype
}
data class GameCharacter(val name: String, val health: Int, val level: Int): Prototype {
override fun clone(): GameCharacter {
return copy() // This Kotlin function creates a clone
}
}
fun main() {
val originalCharacter = GameCharacter(name = "Hero", health = 100, level = 1)
// Cloning the original character
val clonedCharacter = originalCharacter.clone()
// Modifying the cloned character
val modifiedCharacter = clonedCharacter.copy(name = "Hero Clone", level = 2)
println("Original Character: $originalCharacter")
println("Cloned Character: $clonedCharacter")
println("Modified Character: $modifiedCharacter")
}
//Output
Original Character: GameCharacter(name=Hero, health=100, level=1)
Cloned Character: GameCharacter(name=Hero, health=100, level=1)
Modified Character: GameCharacter(name=Hero Clone, health=100, level=2)
Here, we can see how the clone
method creates a new instance of GameCharacter
with the same attributes as the original. The modified character shows that you can change attributes of the cloned instance without affecting the original. This illustrates the Prototype pattern’s ability to create new objects by copying existing ones.
Real-World Use Cases
Creating a Prototype for Game Characters
In a game development scenario, characters often share similar configurations with slight variations. The Prototype Pattern allows the game engine to create these variations without expensive initializations.
For instance, consider a game where you need multiple types of warriors, all with the same base stats but slightly different weapons. Instead of creating new instances from scratch, you can clone a base character and modify the weapon or other attributes.
Now, let’s dive into some Kotlin code and see how we can implement the Prototype Pattern like Kotlin rockstars! 🎸
Step 1: Define the Prototype Interface
We’ll start by creating an interface that all objects (robots, in this case) must implement if they want to be “cloneable.”
interface CloneablePrototype : Cloneable{
fun clone(): CloneablePrototype
}
Simple, right? This CloneablePrototype
interface has one job: provide a method to clone objects.
Step 2: Concrete Prototype (Meet the Robots!)
Let’s create some robots. Here’s a class for our robot soldiers:
data class Robot(
var name: String,
var weapon: String,
var color: String
) : CloneablePrototype {
override fun clone(): Robot {
return Robot(name, weapon, color)
// Note: We could directly use copy() here, but for better understanding, we went with the constructor approach.
}
override fun toString(): String {
return "Robot(name='$name', weapon='$weapon', color='$color')"
}
}
Here’s what’s happening:
- We use Kotlin’s
data class
to make life easier (no need to manually implementequals
,hashCode
, ortoString
). - The
clone()
method returns a newRobot
object with the same attributes as the current one. It’s a perfect copy—like sending a robot through a 3D printer! - The
toString()
method is overridden to give a nice string representation of the robot (for easier debugging and bragging rights).
Step 3: Let’s Build and Clone Our Robots
Let’s simulate an evil villain building an army of robot clones. 🤖
fun main() {
// The original prototype robot
val prototypeRobot = Robot(name = "T-1000", weapon = "Laser Gun", color = "Silver")
// Cloning the robot
val robotClone1 = prototypeRobot.clone().apply {
name = "T-2000"
color = "Black"
}
val robotClone2 = prototypeRobot.clone().apply {
name = "T-3000"
weapon = "Rocket Launcher"
}
println("Original Robot: $prototypeRobot")
println("First Clone: $robotClone1")
println("Second Clone: $robotClone2")
}
Here,
- We start with an original prototype robot (
T-1000
) equipped with a laser gun and shiny silver armor. - Next, we clone it twice. Each time, we modify the clone slightly. One gets a name upgrade and a paint job, while the other gets an epic weapon upgrade. After all, who doesn’t want a rocket launcher?
Output:
Original Robot: Robot(name='T-1000', weapon='Laser Gun', color='Silver')
First Clone: Robot(name='T-2000', weapon='Laser Gun', color='Black')
Second Clone: Robot(name='T-3000', weapon='Rocket Launcher', color='Silver')
Just like that, we’ve created a robot army with minimal effort. They’re all unique, but they share the same essential blueprint. The evil mastermind can sit back, relax, and let the robots take over the world (or maybe start a dance-off).
Cloning a Shape Object in a Drawing Application
In many drawing applications like Adobe Illustrator or Figma, you can create different shapes (e.g., circles, rectangles) and duplicate them. The Prototype pattern can be used to clone these shapes without re-creating them from scratch.
// Prototype interface with a clone method
interface Shape : Cloneable {
fun clone(): Shape
}
// Concrete Circle class implementing Shape
class Circle(var radius: Int) : Shape {
override fun clone(): Shape {
return Circle(this.radius) // Cloning the current object
}
override fun toString(): String {
return "Circle(radius=$radius)"
}
}
// Concrete Rectangle class implementing Shape
class Rectangle(var width: Int, var height: Int) : Shape {
override fun clone(): Shape {
return Rectangle(this.width, this.height) // Cloning the current object
}
override fun toString(): String {
return "Rectangle(width=$width, height=$height)"
}
}
fun main() {
val circle1 = Circle(10)
val circle2 = circle1.clone() as Circle
println("Original Circle: $circle1")
println("Cloned Circle: $circle2")
val rectangle1 = Rectangle(20, 10)
val rectangle2 = rectangle1.clone() as Rectangle
println("Original Rectangle: $rectangle1")
println("Cloned Rectangle: $rectangle2")
}
Here, we define a Shape
interface with a clone()
method. The Circle
and Rectangle
classes implement this interface and provide their own cloning logic.
Duplicating User Preferences in a Mobile App
In mobile applications, user preferences might be complex to initialize. The Prototype pattern can be used to clone user preference objects when creating new user profiles or settings.
// Prototype interface with a clone method
interface UserPreferences : Cloneable {
fun clone(): UserPreferences
}
// Concrete class implementing UserPreferences
class Preferences(var theme: String, var notificationEnabled: Boolean) : UserPreferences {
override fun clone(): UserPreferences {
return Preferences(this.theme, this.notificationEnabled) // Cloning current preferences
}
override fun toString(): String {
return "Preferences(theme='$theme', notificationEnabled=$notificationEnabled)"
}
}
fun main() {
// Original preferences
val defaultPreferences = Preferences("Dark", true)
// Cloning the preferences for a new user
val user1Preferences = defaultPreferences.clone() as Preferences
user1Preferences.theme = "Light" // Customizing for this user
println("Original Preferences: $defaultPreferences")
println("User 1 Preferences: $user1Preferences")
}
Here, the Preferences
object for a user can be cloned when new users are created, allowing the same structure but with different values (like changing the theme).
Cloning Product Prototypes in an E-commerce Platform
An e-commerce platform can use the Prototype pattern to create product variants (e.g., different sizes or colors) by cloning an existing product prototype instead of creating a new product from scratch.
// Prototype interface with a clone method
interface Product : Cloneable {
fun clone(): Product
}
// Concrete class implementing Product
class Item(var name: String, var price: Double, var color: String) : Product {
override fun clone(): Product {
return Item(this.name, this.price, this.color) // Cloning the current product
}
override fun toString(): String {
return "Item(name='$name', price=$price, color='$color')"
}
}
fun main() {
// Original product
val originalProduct = Item("T-shirt", 19.99, "Red")
// Cloning the product for a new variant
val newProduct = originalProduct.clone() as Item
newProduct.color = "Blue" // Changing color for the new variant
println("Original Product: $originalProduct")
println("New Product Variant: $newProduct")
}
In this case, an e-commerce platform can clone the original Item
(product) and modify attributes such as color, without needing to rebuild the entire object.
Advantages and Disadvantages of the Prototype Pattern
Advantages
- Performance optimization: It reduces the overhead of creating complex objects by reusing existing ones.
- Simplified object creation: If the initialization of an object is costly or complex, the prototype pattern makes it easy to create new instances.
- Dynamic customization: You can dynamically modify the cloned objects without affecting the original ones.
Disadvantages
- Shallow vs. Deep Copy: By default, cloning in Kotlin creates shallow copies, meaning that the objects’ properties are copied by reference. You may need to implement deep copying if you want fully independent copies of objects.
- Implementation complexity: Implementing cloneable classes with deep copying logic can become complex, especially if the objects have many nested fields.
Conclusion
In the world of software development, design patterns are more than just abstract concepts; they offer practical solutions to everyday coding challenges. Creational patterns, such as the Factory Method, Abstract Factory, Builder, and Prototype, specifically address the complexities of object creation. These patterns provide structured approaches to streamline object instantiation, whether by simplifying the process of creating related objects or by allowing us to clone existing ones effortlessly.
By incorporating these creational patterns into your Kotlin projects, you can write more maintainable, scalable, and efficient code. Each pattern addresses specific problems, helping developers tackle common design issues with a structured and thoughtful approach. As you continue building applications, these design patterns will become invaluable tools in your development toolkit, empowering you to create cleaner, more adaptable software