As developers, one of our top priorities is ensuring that our Android apps are as secure as possible, especially when they communicate with backend servers over the internet. With cyber threats constantly evolving, it’s essential to take proactive steps in protecting our data and users’ information. One effective technique that I’ve found invaluable is Certificate Pinning.
In this post, I want to walk you through what certificate pinning is, how it works, and why it’s such an important security measure for Android apps. I’ll share my insights and experiences on the topic, and together, we’ll understand why implementing this in our apps can significantly reduce security risks.
What is Certificate Pinning?
Let’s start with the basics: certificate pinning is a security technique where we bind or “pin” the certificate of a trusted server to the app, ensuring that our app communicates only with that server. By doing this, we effectively prevent attackers from using fraudulent or compromised certificates to intercept or tamper with data during the transmission.
To make it clearer, imagine you’re communicating with a server over HTTPS. Typically, your app will trust any certificate that matches the server’s hostname, relying on a trusted Certificate Authority (CA). However, this method leaves an opening for man-in-the-middle (MITM) attacks, where an attacker could insert themselves into the communication by using a forged certificate. Certificate pinning closes this gap by allowing your app to trust only a specific certificate (or public key) for the server’s domain.
Why is Certificate Pinning So Important?
As Android developers, we are constantly dealing with user data, whether it’s login credentials, payment information, or personal preferences. Without proper security measures in place, attackers can exploit vulnerabilities to intercept this data, potentially causing serious harm to our users and our reputation.
By implementing certificate pinning, we are drastically reducing the risk of MITM attacks. These types of attacks are particularly common when users are connected to unsecured or public networks, like public Wi-Fi. Even with encryption in place, attackers could still pose a significant threat by impersonating the server. Pinning ensures that even if an attacker manages to obtain a valid certificate from a compromised CA, it won’t work for our app.
How Does Certificate Pinning Work in Android?
In Android, certificate pinning is implemented by storing a hash of the server’s certificate (or public key) in the app. Whenever the app establishes a connection to the server, it checks whether the certificate presented by the server matches the pinned certificate. If it doesn’t, the connection is immediately terminated.
Here’s a simple breakdown of the process:
- Obtain the server certificate: First, we need to retrieve the server’s public key or certificate, usually in the form of a SHA-256 hash or the certificate itself.
- Pin it in the app: We add this certificate hash or public key pin directly into our app’s code. This ensures that the app only accepts certificates that match.
- Verify during connection: When the app tries to connect to the server, it checks the server’s certificate against the pinned certificate. If there’s a mismatch, the connection is rejected, and the app is prevented from communicating with the server.
The beauty of certificate pinning is its simplicity and the level of security it offers, especially for protecting sensitive user data.
How to Implement Certificate Pinning in Android
Implementing certificate pinning in Android is relatively straightforward. You can use libraries like OkHttp or Retrofit for HTTP requests, which support certificate pinning out of the box. Let’s dive into the implementation part. We’ll break this down into digestible steps, starting with setting up the basic SSL connection and then adding certificate pinning.
Basic SSL/TLS Implementation in Android
First, let’s understand how a regular HTTPS connection is made in Android. Typically, Android uses OkHttp or HttpURLConnection to make network requests.
Basic example using OkHttp to make an HTTPS request
import okhttp3.OkHttpClient
import okhttp3.Request
fun makeRequest() {
val client = OkHttpClient()
val request = Request.Builder()
.url("https://your-website.com/api/endpoint")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
println(response.body!!.string())
}
}
This is a simple HTTPS request using OkHttp, which by default trusts the entire chain of trusted CAs. However, we need more control if we are to ensure that the app only communicates with our server.
This is a simple HTTPS request using OkHttp, which by default trusts the entire chain of trusted CAs. However, we need more control if we are to ensure that the app only communicates with our server.
Implementing Certificate Pinning with OkHttp
To implement certificate pinning, we need to modify the OkHttpClient to trust only a specific certificate (or public key).
First, download the certificate of your server. This can be done through various tools like browsers or OpenSSL.
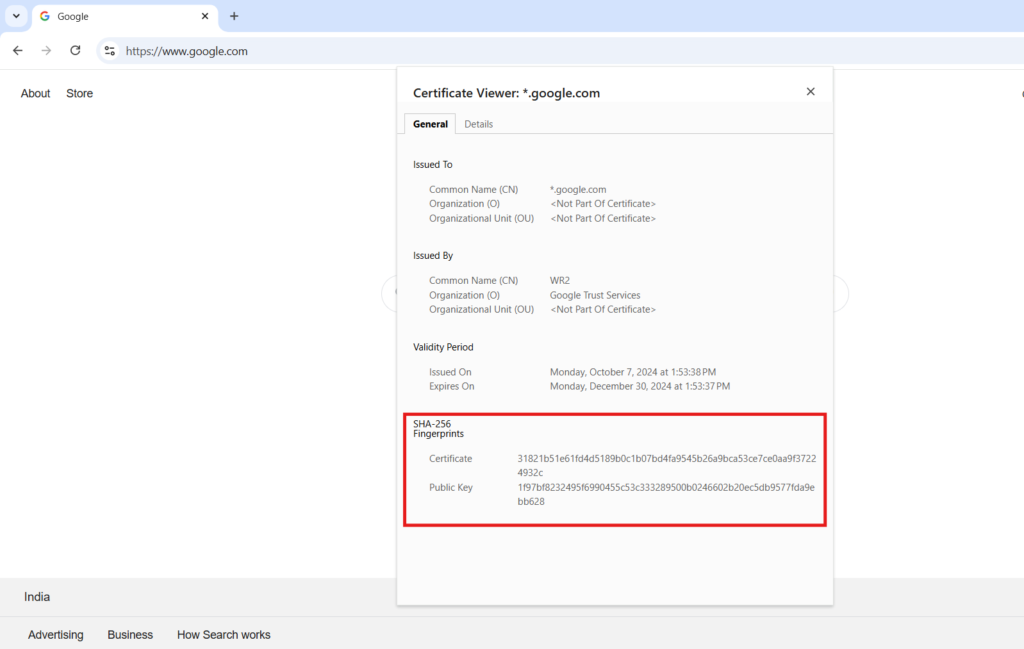
For this example, we will pin the certificate in the form of a SHA256 hash of the public key.
Let’s look at how to implement this.
import okhttp3.CertificatePinner
import okhttp3.OkHttpClient
import okhttp3.Request
fun pinCertificate() {
// SHA256 hash of the server's public key
val certificatePinner = CertificatePinner.Builder()
.add("your-website.com", "sha256/your_certificate_hash_here")
.build()
val client = OkHttpClient.Builder()
.certificatePinner(certificatePinner) // Attach the pin to the OkHttp client
.build()
val request = Request.Builder()
.url("https://your-website.com/api/endpoint")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
println(response.body!!.string())
}
}
Here,
- CertificatePinner.Builder(): This is where you define which certificates are trusted. You can pin certificates by their domain and their corresponding SHA256 hash.
- sha256/your_certificate_hash_here: This is the hash of the public key of the server certificate. Replace it with your server’s actual hash.
- OkHttpClient.Builder(): Here, we attach the certificate pinning to the OkHttp client, ensuring that only certificates matching the pinned hash are trusted.
In this code, if the server’s certificate doesn’t match the pinned certificate, the connection will fail, preventing any communication with unauthorized servers.
Handling Multiple Pinning with Backup Certificates
What happens if your server’s certificate is updated or rotated? This is where backup pinning comes into play. By pinning multiple certificates or public keys, you allow your app to connect even if one certificate changes.
fun pinMultipleCertificates() {
val certificatePinner = CertificatePinner.Builder()
.add("your-website.com", "sha256/AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA=") // Old pin
.add("your-website.com", "sha256/BBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBB=") // New pin
.build()
val client = OkHttpClient.Builder()
.certificatePinner(certificatePinner)
.build()
val request = Request.Builder()
.url("https://your-website.com/api/endpoint")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
println(response.body!!.string())
}
}
This ensures that if your certificate rotates, the app will still trust the new certificate as long as its public key hash is pinned.
Dynamically Pinning Certificates
In some scenarios, it might be necessary to pin certificates dynamically, particularly when working with multiple environments or during development. You can achieve this by fetching the certificate hash at runtime.
fun getPinnedCertificate(environment: String): String {
return when (environment) {
"production" -> "sha256/AAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAAA="
"staging" -> "sha256/BBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBBB="
else -> throw IllegalArgumentException("Unknown environment")
}
}
fun pinCertificateDynamically(environment: String) {
val pin = getPinnedCertificate(environment)
val certificatePinner = CertificatePinner.Builder()
.add("your-website.com", pin)
.build()
val client = OkHttpClient.Builder()
.certificatePinner(certificatePinner)
.build()
val request = Request.Builder()
.url("https://your-website.com/api/endpoint")
.build()
client.newCall(request).execute().use { response ->
if (!response.isSuccessful) throw IOException("Unexpected code $response")
println(response.body!!.string())
}
}
Here, the correct pin is selected based on the environment, giving you flexibility across various stages of development and deployment.
Using HttpsURLConnection for Certificate Pinning (Old Approach)
If you aren’t using OkHttp, you can also pin certificates with HttpsURLConnection
. This approach involves implementing a custom TrustManager
that validates certificates against pinned ones. Old is gold, but it’s not recommended for new development; however, if you’re working with legacy code, it’s worth considering 🙂
import javax.net.ssl.*
import java.security.cert.Certificate
import java.security.cert.X509Certificate
fun pinCertificate(certificates: Array<Certificate>) {
val x509Certificate = certificates[0] as X509Certificate
val pinnedPublicKey = "YOUR_PINNED_PUBLIC_KEY" // Replace with your public key
val certificatePublicKey = x509Certificate.publicKey.encoded.toString(Charsets.UTF_8)
if (pinnedPublicKey != certificatePublicKey) {
throw SSLException("Certificate pinning failure!")
}
}
Here,
X509Certificate
represents the server certificate.pinnedPublicKey
should be replaced with the actual public key you want to pin.
Testing Certificate Pinning
To test your certificate pinning:
- Use Debug Builds: Implement certificate pinning in a debug build to ensure it’s configured correctly.
- Test with Interceptors: Use a network interceptor (such as Charles Proxy) to simulate MITM attacks. If pinning works, the app should reject the connection.
Challenges and Considerations
While certificate pinning is a powerful tool for securing your app, there are a few challenges and considerations to keep in mind:
- Updating pins: If the server’s certificate needs to be changed (for example, when the certificate expires), we’ll need to update the pinned certificate in the app and release a new version. This means we must ensure the certificate is updated regularly and we have a good process in place for deploying new app versions.
- Risk of breakage: If the pinning is too strict, we might face situations where legitimate changes to the server’s certificate (e.g., switching to a different CA) could break the connection. This is why it’s important to monitor certificate changes and have an update strategy.
- Backup mechanism: We can implement a backup mechanism to allow updates to the certificate pin during runtime, giving us flexibility without forcing users to update the app every time a pin change occurs.
Best Practices
Here are a few best practices to ensure we’re using certificate pinning effectively:
1. Pin multiple certificates: It’s a good idea to pin more than one certificate or public key. This gives us flexibility in case of certificate rotation or renewal without breaking the app’s functionality.
2. Handle certificate expiry gracefully: Plan for certificate expiration by regularly rotating certificates and testing your app with updated pins before they expire.
3. Hardcoding Pins: Avoid hardcoding pins in your app for security reasons. If the app is decompiled, attackers can retrieve the pinned certificate hash. Consider dynamically fetching pins or using obfuscation techniques to secure your app.
4. Managing Multiple Environments: As demonstrated earlier, dynamically switching pins based on environments (development, staging, production) is crucial. Be careful not to expose development pins in production environments.
5. Monitor and audit pins: Regularly audit your pinned certificates to ensure they’re up-to-date and match the server’s current certificates. You can also use logging to track failed pin validation attempts.
6. Fallback to normal SSL checks: In cases where pinning fails, allow the app to fall back to the standard SSL/TLS verification to avoid completely blocking the user.
Conclusion
Certificate pinning is a powerful security measure that I highly recommend implementing in our Android apps. It adds an extra layer of protection against MITM attacks and ensures that sensitive data is securely transmitted between the app and the server. While it comes with its challenges, like the need for certificate updates, the security benefits far outweigh the trade-offs. By incorporating pinning into our security strategy, we can give users the peace of mind that their data is safe, even in potentially risky environments.
So, next time you’re working on an Android app, take a few moments to consider certificate pinning. It’s one of those simple yet impactful measures that can make a world of difference in securing our applications.