Jetpack Compose has taken the Android UI development world by storm. It simplifies UI development by making it declarative and functional. But one of the first things you’ll encounter when using Jetpack Compose is the setContent
function. In this blog, we’ll break down what setContent
function is, how it works, and why it matters.
What is setContent
Function?
In traditional Android UI development (using XML layouts), you would typically call setContentView()
inside your Activity
to set the screen’s layout. With Jetpack Compose, things have changed!
setContent
replaces setContentView
when you want to define your UI using Compose.
From now on, if you use Jetpack Compose, use this:
// This is defined for illustration purposes. You can use any composition or composable functions inside setContent{...}.
setContent {
MyComposableContent()
}
Instead of using the older setContentView
method with XML layouts.
Basic Syntax Example of setContent
function
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.material3.Text
import android.os.Bundle
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
Text("Hello, Jetpack Compose!")
}
}
}
How Does setContent
Work?
The setContent
function is part of the ComponentActivity
class. It allows you to set a Composable block as your UI content.
What Happens When You Call setContent
?
- Initializes the Compose UI framework.
- Sets the content view with a root
ComposeView
that renders your composables. - Observes recompositions to keep the UI up-to-date with state changes.
Essentially, setContent
is the entry point for Jetpack Compose to build and display your UI.
Function Signature
Here’s the function definition again for reference:
fun ComponentActivity.setContent(
parent: CompositionContext? = null,
content: @Composable () -> Unit
)
Here,
Extension Function:setContent()
is an extension function for ComponentActivity
, which means you can call it on any ComponentActivity
or its subclasses like AppCompatActivity
.
parent: CompositionContext? = null
:
- This optional parameter represents the parent composition context. In Jetpack Compose, a
CompositionContext
coordinates updates and re-compositions of composable functions. - Passing a parent helps coordinate the lifecycle of this composition with another, which is useful for nested composable hierarchies.
content: @Composable () -> Unit
:
- This parameter is a composable lambda function that defines the UI contents.
- For Example,
setContent {
Text("Hello Compose!")
}
Preview
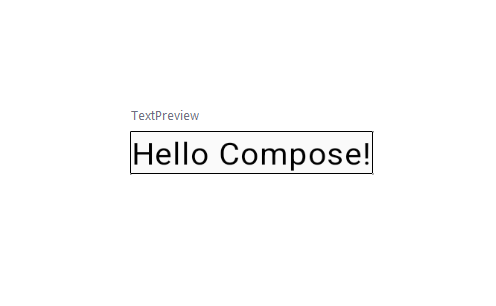
I am going a little out of context, but it’s okay. I feel it’s important for our next discussion. Let’s think: what do you think might be inside the setContent()
function body ({..})?
fun ComponentActivity.setContent(){...}
Does Android still use the old View system at its core, or has it introduced something new? If Android has introduced a new system, how can we still support our old or legacy code written in XML?
The answer is simple: ComposeView. It acts as a bridge between Jetpack Compose and the traditional View system. This allows us to integrate Jetpack Compose with existing XML-based layouts and continue using our legacy code when needed.
Although Jetpack Compose offers a completely new way to build UIs, it does not use the old View system internally for rendering. Instead, Compose has its own rendering mechanism. However, thanks to ComposeView
and interoperability APIs like AndroidView
, Compose and the View system can seamlessly work together. This is why setContent()
can host composables within an activity or fragment, enabling us to mix both approaches in a single app.
It’s just an additional insight. Now, let’s get back on track: inside setContent()
, we’ll find a ComposeView
. Let’s explore what’s inside it to better understand how it works.
public fun ComponentActivity.setContent(
parent: CompositionContext? = null,
content: @Composable () -> Unit
) {
val existingComposeView = window.decorView
.findViewById<ViewGroup>(android.R.id.content)
.getChildAt(0) as? ComposeView
if (existingComposeView != null) with(existingComposeView) {
setParentCompositionContext(parent)
setContent(content)
} else ComposeView(this).apply {
// Set content and parent **before** setContentView
// to have ComposeView create the composition on attach
setParentCompositionContext(parent)
setContent(content)
// Set the view tree owners before setting the content view so that the inflation process
// and attach listeners will see them already present
setOwners()
setContentView(this, DefaultActivityContentLayoutParams)
}
}
Let’s break down the body of the function:
val existingComposeView = window.decorView
.findViewById<ViewGroup>(android.R.id.content)
.getChildAt(0) as? ComposeView
Here,
window.decorView
:
- The root view of the window where your activity’s content resides.
.findViewById<ViewGroup>(android.R.id.content)
:
android.R.id.content
is the standard ID for the content view of an activity.- This line finds the view group that holds the content of the activity.
.getChildAt(0) as? ComposeView
:
- This retrieves the first child of the content view (assuming it’s a
ComposeView
). - The
as? ComposeView
safely casts the child to aComposeView
if it’s already present.
Next, there’s a check for whether the ComposeView
already exists:
if (existingComposeView != null) with(existingComposeView) {
setParentCompositionContext(parent)
setContent(content)
}
if (existingComposeView != null)
:
- Checks if a
ComposeView
is already present as the first child of the content view.
with(existingComposeView)
:
- If the
ComposeView
exists, this block configures it: setParentCompositionContext(parent)
: Sets the parent composition context for coordinating composition updates.setContent(content)
: Sets the new composable content to be displayed in theComposeView
.
This approach reuses the existing ComposeView
if available, avoiding the need to create a new one.
If no existing ComposeView
is found, a new one is created:
else ComposeView(this).apply {
// Set content and parent **before** setContentView
// to have ComposeView create the composition on attach
setParentCompositionContext(parent)
setContent(content)
// Set the view tree owners before setting the content view so that the inflation process
// and attach listeners will see them already present
setOwners()
setContentView(this, DefaultActivityContentLayoutParams) // Note : here this is composeview as it is inside apply
}
ComposeView(this)
:
- Creates a new
ComposeView
, passing the currentComponentActivity
as the context.
setParentCompositionContext(parent)
:
- Sets the parent composition context for coordinating updates.
setContent(content)
:
- Sets the composable lambda as the content of the
ComposeView
.
setOwners()
:
- Ensures the
ViewTreeLifecycleOwner
andViewTreeViewModelStoreOwner
are set. These owners are necessary for handling the lifecycle and ViewModel integration properly.
setContentView(this, DefaultActivityContentLayoutParams)
:
- Sets the newly created
ComposeView
as the root view of the activity, using default layout parameters.
In short,
Reusing Existing
ComposeView
:If there’s already a
ComposeView
, the function updates its content directly, improving efficiency by avoiding creating a new view.Creating New
ComposeView
:If no
ComposeView
exists, a new one is created and set as the activity’s content view.Composition Context:
The
parent
parameter helps maintain the composable hierarchy and ensures updates are properly synchronized.Lifecycle Awareness:
setOwners()
ensures that theComposeView
has the necessary lifecycle owners before it gets attached to the activity.
By the way, what exactly is ComposeView
?
I already gave a little hint, but there’s still more to explore. So, let’s dive into the details.
ComposeView
is a special View
provided by Jetpack Compose that serves as a bridge between the traditional Android View system and the Compose framework. Essentially, it allows you to embed composable UI within a standard Android View hierarchy.
Let’s break down what ComposeView
is, how it works, and where it’s useful.
Overview of ComposeView
The ComposeView
class extends AbstractComposeView
, making it a View
that can host Jetpack Compose UI components.
- Purpose: Allows seamless integration of Jetpack Compose content into existing Android View-based UI. It acts as a container for composable content in environments that primarily use Views (e.g., activities or fragments that aren’t fully migrated to Compose).
- Key Functionality: Provides a method
setContent
to define the Compose UI content.
Type
ComposeView
does not directly extend android.view.View
. Instead:
android.view.View
└── android.view.ViewGroup
└── androidx.compose.ui.platform.AbstractComposeView
└── androidx.compose.ui.platform.ComposeView
ComposeView
extends androidx.compose.ui.platform.AbstractComposeView
, which in turn extends android.view.ViewGroup
, and ultimately, ViewGroup
extends android.view.View
.
Here’s an actual code snippet:
class ComposeView @JvmOverloads constructor(
context: Context,
attrs: AttributeSet? = null,
defStyleAttr: Int = 0
) : AbstractComposeView(context, attrs, defStyleAttr) {
private val content = mutableStateOf<(@Composable () -> Unit)?>(null)
@Suppress("RedundantVisibilityModifier")
protected override var shouldCreateCompositionOnAttachedToWindow: Boolean = false
private set
@Composable
override fun Content() {
content.value?.invoke()
}
override fun getAccessibilityClassName(): CharSequence {
return javaClass.name
}
/**
* Set the Jetpack Compose UI content for this view.
* Initial composition will occur when the view becomes attached to a window or when
* [createComposition] is called, whichever comes first.
*/
fun setContent(content: @Composable () -> Unit) {
shouldCreateCompositionOnAttachedToWindow = true
this.content.value = content
if (isAttachedToWindow) {
createComposition()
}
}
}
Here,
Constructor:
- Uses
@JvmOverloads
to allow flexibility in calling the constructor with fewer parameters. - Inherits from
AbstractComposeView
, which handles Jetpack Compose rendering in a View-based system.
State to Hold Content:
content
is amutableStateOf
variable that holds a nullable Composable lambda (@Composable () -> Unit
).
Flag for Composition on Attachment:
shouldCreateCompositionOnAttachedToWindow
ensures that the composition is created when the view is attached to the window.- By default, it’s
false
. It becomestrue
whensetContent
is called.
Composable Content Rendering:
- The
Content()
function is overridden fromAbstractComposeView
. - If
content
is not null, it invokes the stored Composable lambda.
Accessibility:
getAccessibilityClassName()
returns the class name for accessibility purposes.
setContent Function:
- Accepts a Composable function and sets it to the
content
property. - Sets
shouldCreateCompositionOnAttachedToWindow
totrue
. - If the view is already attached to the window (
isAttachedToWindow
), it immediately callscreateComposition()
to render the content.
Adding ComposeView in XML
To add ComposeView
in an XML layout, follow these steps:
Add Compose dependencies in your build.gradle
:
// use latest versions
implementation "androidx.activity:activity-compose:1.7.2"
implementation "androidx.compose.ui:ui:1.6.0"
implementation "androidx.compose.material:material:1.6.0"
Add ComposeView
in your XML layout:
<androidx.compose.ui.platform.ComposeView
android:id="@+id/composeView"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
Adding Composable to ComposeView in Activity/Fragment
In your Activity or Fragment, set the content for ComposeView
.
val composeView = findViewById<ComposeView>(R.id.composeView)
composeView.setContent {
MaterialTheme {
Greeting("Compose in XML")
}
}
@Composable
fun Greeting(name: String) {
Text(text = "Hello, $name!", style = MaterialTheme.typography.h6)
}
Integrating ComposeView in a Fragment
If you are using ComposeView
in a Fragment:
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
return ComposeView(requireContext()).apply {
setContent {
MaterialTheme {
Greeting("Compose in Fragment")
}
}
}
}
Why Use ComposeView?
- Incremental Migration: Migrate your app gradually without rewriting everything.
- Reuse Composables: Use powerful Composable functions in legacy projects.
- Flexibility: Combine both systems seamlessly.
- Modern UI Components: Bring Compose’s declarative UI and reactive state management to older architectures.
Key Differences: setContent
vs setContentView
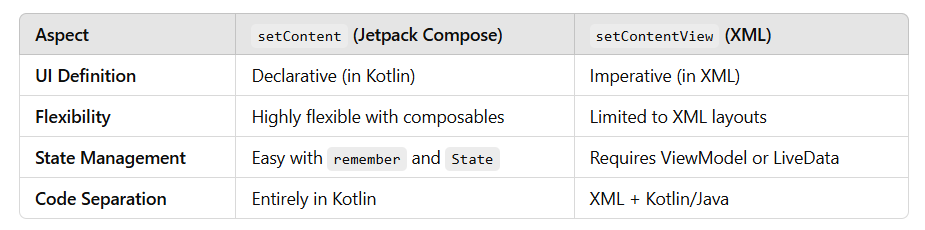
Best Practices for Using setContent
Keep setContent
Clean and Simple:
- The lambda inside
setContent
should primarily call composable functions. Avoid complex logic inside the lambda to keep your code clean and readable.
Use Themes and Styling:
- Wrap your content in a theme (e.g.,
MaterialTheme
) to ensure consistent styling across your app.
Separate Concerns:
- Structure your composables into separate functions and files based on their functionality. This improves readability and maintainability.
State Management:
- Use
remember
andmutableStateOf
for local state management within composables. For shared state, consider usingViewModel
andLiveData
orStateFlow
.
Common Pitfalls to Avoid
Blocking the UI Thread:
- Avoid long-running tasks or complex calculations inside
setContent
. Perform such tasks in a background thread usingCoroutineScope
.
Deeply Nested Composables:
- Keep composable functions small and focused to avoid deeply nested structures, which can affect performance and readability.
Ignoring State Changes:
- Ensure state changes trigger recomposition by using
mutableStateOf
or other state management solutions.
Conclusion
The setContent
function is your entry point to building UI with Jetpack Compose. It replaces setContentView
and opens the door to declarative, composable-based UI development. By understanding how setContent
works and following best practices, you can create clean, maintainable, and dynamic user interfaces in your Android applications.
Jetpack Compose simplifies UI development, making it a pleasure to design responsive and interactive apps. Embrace setContent
and the power of composables to elevate your Android development experience!