Have you ever stopped to marvel at how breathtaking mobile games have become? Think about the background graphics in popular games like PUBG or Pokémon GO. (Honest confession: I haven’t played these myself, but back in my college days, my friends and I used to have epic Counter-Strike and I.G.I. 2 sessions—and, funny enough, the same group now plays PUBG—except me!) Anyway, the real question is: have you noticed just how detailed the game worlds are? You’ve got lush grass fields, towering trees, cozy houses, and fluffy clouds—so many objects that make these games feel alive. But here’s the kicker: how do they manage all that without your phone overheating or the game lagging as the action intensifies?
It turns out that one of the sneaky culprits behind game lag is the sheer number of objects being created over and over, all of which take up precious memory. That’s where the Flyweight Design Pattern swoops in like a hero.
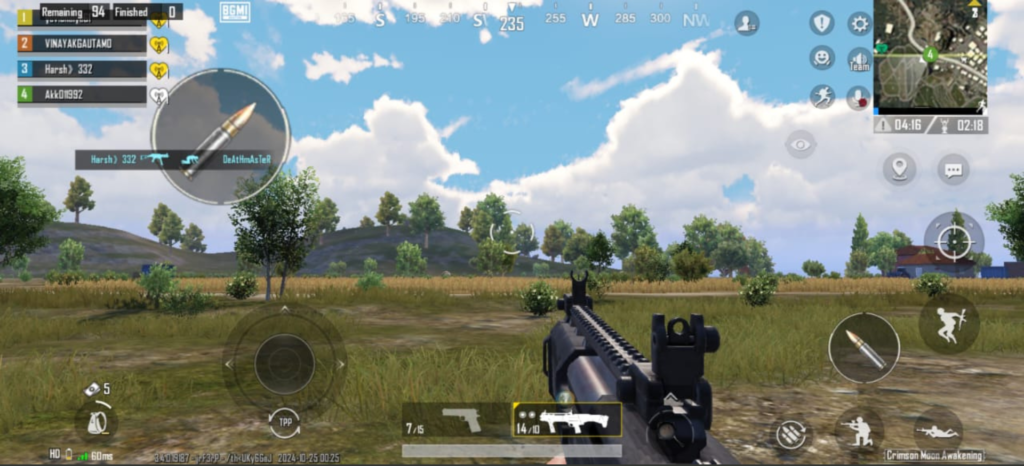
Picture this: you’re playing a game with hundreds of trees, houses, and patches of grass. Now, instead of creating a brand-new tree or patch of grass every time, wouldn’t it make sense to reuse these elements when possible? After all, many of these objects share the same traits—like the green color of grass or the texture of tree leaves. The Flyweight pattern allows the game to do just that: reuse common properties across objects to save memory and keep performance snappy.
In this blog, we’re going to break down exactly how the Flyweight Design Pattern works, why it’s such a game-changer for handling large numbers of similar objects, and how you can use it to optimize your own projects. Let’s dive in and find out how it all works!
What is the Flyweight Pattern?
The Flyweight Pattern is a structural design pattern that reduces memory consumption by sharing as much data as possible with similar objects. Instead of storing the same data repeatedly across multiple instances, the Flyweight pattern stores shared data in a common object and only uses unique data in individual objects. This concept is particularly useful when creating a large number of similar objects.
The core idea behind this pattern is to:
1. Identify and separate intrinsic and extrinsic data.
Intrinsic data is shared across all objects and remains constant.
Extrinsic data is unique to each object instance.
2. Store intrinsic data in a shared flyweight object, and pass extrinsic data at runtime.
Let’s break it down with our game example. Imagine a field of grass where each blade has a common characteristic: color. All the grass blades are green, which remains constant across the entire field. This is the intrinsic data — something that doesn’t change, like the color.
Now, think about the differences between the blades of grass. Some may vary in height or shape, like a blade being wider in the middle or taller than another. Additionally, the exact position of each blade in the field differs. These varying factors, such as height and position, are the extrinsic data.
Without using the Flyweight pattern, you would store both the common and unique data for each blade of grass in separate objects, which quickly leads to redundancy and memory bloat. However, with the Flyweight pattern, we extract the common data (the color) and share it across all grass blades using one object. The varying data (height, shape, and position) is stored separately for each blade, reducing memory usage significantly.
In short, the Flyweight pattern helps optimize your game by sharing common attributes while keeping only the unique properties in separate objects. This is especially useful when working with a large number of similar objects like blades of grass in a game.
Structure of Flyweight Design Pattern
Before we jump into the code, let’s break down the structure of the Flyweight Design Pattern to understand how it works:
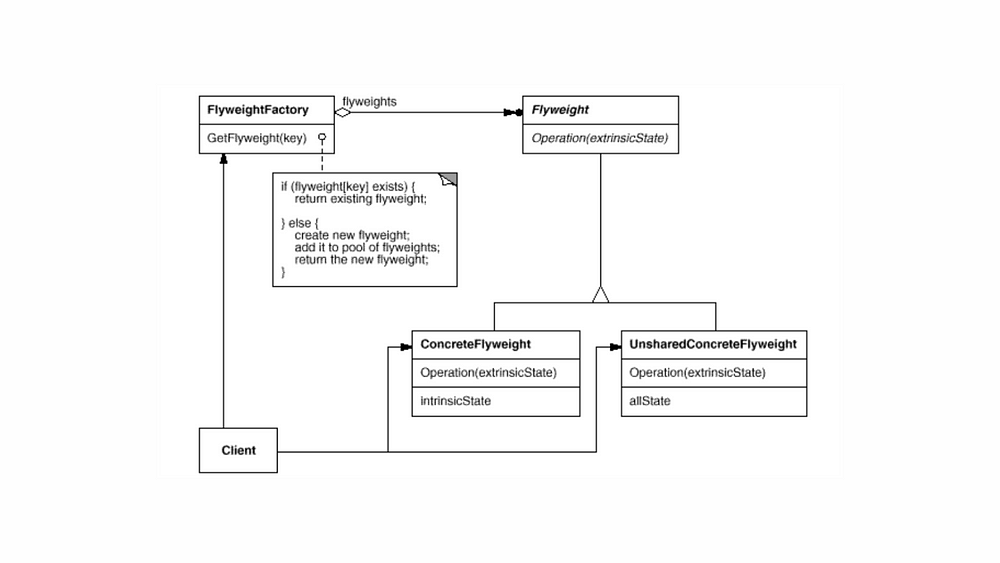
Flyweight
- Defines an interface that allows flyweight objects to receive and act on external (extrinsic) state.
ConcreteFlyweight
- Implements the Flyweight interface and stores intrinsic state (internal, unchanging data).
- Must be shareable across different contexts.
UnsharedConcreteFlyweight
- While the Flyweight pattern focuses on sharing information, there can be cases where instances of concrete flyweight classes are not shared. These objects may hold their own state.
FlyweightFactory
- Creates and manages flyweight objects.
- Ensures that flyweight objects are properly shared to avoid duplication.
Client
- Holds references to flyweight objects.
- Computes or stores the external (extrinsic) state that the flyweights use.
Basically, the Flyweight pattern works by dividing the object state into two categories:
- Intrinsic State: Data that can be shared across multiple objects. It is stored in a shared, immutable object.
- Extrinsic State: Data that is unique to each object instance and is passed to the object when it is used.
Using a Flyweight Factory, objects that share the same intrinsic state are created once and reused multiple times. This approach leads to significant memory savings.
Real World Examples
Grass Field Example
Let’s first implement the Flyweight pattern with a grass field example by creating a Flyweight interface, defining concrete Flyweight classes, building a factory to manage them, and demonstrating their usage with a client.
Define the Flyweight Interface
This interface will declare the method that the flyweight objects will implement.
interface GrassBlade {
fun display(extrinsicState: GrassBladeState)
}
Create ConcreteFlyweight Class
This class represents the shared intrinsic state (color) of the grass blades.
class ConcreteGrassBlade(private val color: String) : GrassBlade {
override fun display(extrinsicState: GrassBladeState) {
println("Grass Blade Color: $color, Height: ${extrinsicState.height}, Position: ${extrinsicState.position}")
}
}
Create UnsharedConcreteFlyweight Class (if necessary)
If you need blades that may have unique characteristics, you can have this class. For simplicity, we won’t implement any specific logic here.
class UnsharedConcreteGrassBlade : GrassBlade {
// Implementation for unshared concrete flyweight if needed
override fun display(extrinsicState: GrassBladeState) {
// Not shared, just a placeholder
}
}
Create the FlyweightFactory
This factory class will manage the creation and sharing of grass blade objects.
class GrassBladeFactory {
private val grassBlades = mutableMapOf<String, GrassBlade>()
fun getGrassBlade(color: String): GrassBlade {
return grassBlades.computeIfAbsent(color) { ConcreteGrassBlade(color) }
}
}
Define the Extrinsic State
This class holds the varying data for each grass blade.
data class GrassBladeState(val height: Int, val position: String)
Implement the Client Code
Here, we will create instances of grass blades and display their properties using the Flyweight pattern.
fun main() {
val grassBladeFactory = GrassBladeFactory()
// Creating some grass blades with shared intrinsic state (color) and varying extrinsic state
val grassBlades = listOf(
Pair(grassBladeFactory.getGrassBlade("Green"), GrassBladeState(5, "A1")),
Pair(grassBladeFactory.getGrassBlade("Green"), GrassBladeState(7, "A2")),
Pair(grassBladeFactory.getGrassBlade("Green"), GrassBladeState(6, "B1")),
Pair(grassBladeFactory.getGrassBlade("Green"), GrassBladeState(4, "B2")),
Pair(grassBladeFactory.getGrassBlade("Green"), GrassBladeState(5, "C1")),
Pair(grassBladeFactory.getGrassBlade("Green"), GrassBladeState(5, "C2"))
)
// Displaying the grass blades
for ((grassBlade, state) in grassBlades) {
grassBlade.display(state)
}
}
Output
Grass Blade Color: Green, Height: 5, Position: A1
Grass Blade Color: Green, Height: 7, Position: A2
Grass Blade Color: Green, Height: 6, Position: B1
Grass Blade Color: Green, Height: 4, Position: B2
Grass Blade Color: Green, Height: 5, Position: C1
Grass Blade Color: Green, Height: 5, Position: C2
Here,
- GrassBlade Interface: This defines a method
display
that takes anextrinsicState
. - ConcreteGrassBlade Class: Implements the Flyweight interface and stores the intrinsic state (color).
- GrassBladeFactory: Manages the creation and sharing of
ConcreteGrassBlade
instances based on color. - GrassBladeState Class: Holds the extrinsic state for each grass blade, such as height and position.
- Main Function: This simulates the game, creates multiple grass blades, and demonstrates how shared data and unique data are handled efficiently.
Forest Example
Let’s build the forest now. Here, we’ll render a forest with grass, trees, and flowers, reusing objects to minimize memory usage and improve performance by applying the Flyweight pattern.
In this example, the ForestObject
will represent a shared object (like grass, trees, and flowers), and the Forest
class will be responsible for managing and rendering these objects with variations in their positions and other properties.
// Step 1: Flyweight Interface
interface ForestObject {
fun render(x: Int, y: Int) // Render object at given coordinates
}
// Step 2: Concrete Flyweight Classes (Grass, Tree, Flower)
class Grass : ForestObject {
private val color = "Green" // Shared property
override fun render(x: Int, y: Int) {
println("Rendering Grass at position ($x, $y) with color $color")
}
}
class Tree : ForestObject {
private val type = "Oak" // Shared property
override fun render(x: Int, y: Int) {
println("Rendering Tree of type $type at position ($x, $y)")
}
}
class Flower : ForestObject {
private val color = "Yellow" // Shared property
override fun render(x: Int, y: Int) {
println("Rendering Flower at position ($x, $y) with color $color")
}
}
// Step 3: Flyweight Factory (Manages the creation and reuse of objects)
class ForestObjectFactory {
private val objects = mutableMapOf<String, ForestObject>()
// Returns an existing object or creates a new one if it doesn't exist
fun getObject(type: String): ForestObject {
return objects.getOrPut(type) {
when (type) {
"Grass" -> Grass()
"Tree" -> Tree()
"Flower" -> Flower()
else -> throw IllegalArgumentException("Unknown forest object type")
}
}
}
}
// Step 4: Forest Class (Client code to manage and render the forest)
class Forest(private val factory: ForestObjectFactory) {
private val objectsInForest = mutableListOf<Pair<ForestObject, Pair<Int, Int>>>()
// Adds a new object with specified type and coordinates
fun plantObject(type: String, x: Int, y: Int) {
val forestObject = factory.getObject(type)
objectsInForest.add(Pair(forestObject, Pair(x, y)))
}
// Renders the entire forest
fun renderForest() {
for ((obj, position) in objectsInForest) {
obj.render(position.first, position.second)
}
}
}
// Step 5: Testing the Flyweight Pattern
fun main() {
val factory = ForestObjectFactory()
val forest = Forest(factory)
// Planting various objects in the forest (reusing the same objects)
forest.plantObject("Grass", 10, 20)
forest.plantObject("Grass", 15, 25)
forest.plantObject("Tree", 30, 40)
forest.plantObject("Tree", 35, 45)
forest.plantObject("Flower", 50, 60)
forest.plantObject("Flower", 55, 65)
// Rendering the forest
forest.renderForest()
}
Here,
Flyweight Interface (ForestObject
): This interface defines the method render
, which will be used to render objects in the forest.
Concrete Flyweights (Grass
, Tree
, Flower
): These classes implement the ForestObject
interface and have shared properties like color and type that are common across multiple instances.
Flyweight Factory (ForestObjectFactory
): This class manages the creation and reuse of objects. It ensures that if an object of the same type already exists, it will return the existing object rather than creating a new one.
Client Class (Forest
): This class is responsible for planting objects in the forest and rendering them. It uses the factory to obtain objects and stores their positions.
Main Function: In the main
function, we plant several objects in the forest, but thanks to the Flyweight Design Pattern, we reuse existing objects to save memory.
Output
Rendering Grass at position (10, 20) with color Green
Rendering Grass at position (15, 25) with color Green
Rendering Tree of type Oak at position (30, 40)
Rendering Tree of type Oak at position (35, 45)
Rendering Flower at position (50, 60) with color Yellow
Rendering Flower at position (55, 65) with color Yellow
Basically, even though we planted multiple grass, tree, and flower objects, the game only created one object per type, thanks to the factory. These objects are reused, with only their positions varying. This approach saves memory and improves performance, especially when there are thousands of similar objects in the game world.
Few more Usecases
- Text Rendering Systems: Letters that share the same font, size, and style can be stored as flyweights, while the position of each character in the document is extrinsic.
- Icons in Operating Systems: Many icons in file browsers share the same image but differ in position or name.
- Web Browsers: Rendering engines often use flyweights to manage CSS style rules, ensuring that the same styles aren’t recalculated multiple times.
Advantages of the Flyweight Pattern
- Memory Efficient: Reduces the number of objects by sharing common data, thus saving memory.
- Improves Performance: With fewer objects to manage, the program can run faster.
- Scalability: Useful in applications with many similar objects (e.g., games, graphical applications).
Drawbacks of the Flyweight Pattern
- Increased Complexity: The pattern introduces more complexity as you need to manage both intrinsic and extrinsic state separately.
- Less Flexibility: Changes to the intrinsic state can affect all instances of the flyweight, which might not be desired in all situations.
- Thread-Safety Issues: Careful management of shared state is required in a multi-threaded environment.
When to Use Flyweight Pattern
- When an application has many similar objects: If creating each object individually would use too much memory, like in games with numerous characters or environments.
- When object creation is expensive: Reusing objects can prevent the overhead of frequently creating new objects.
- When intrinsic and extrinsic states can be separated: The pattern is effective when most object properties are shareable.
Conclusion
The Flyweight design pattern is a powerful tool when you need to optimize memory usage by sharing objects with similar properties. In this post, we explored how the Flyweight pattern works, saw a real-world analogy, and implemented it in Kotlin using grass field and forest examples in a game.
While the Flyweight pattern can be a great way to reduce memory usage, it’s important to carefully analyze whether it’s necessary in your specific application. For simple applications, this pattern might introduce unnecessary complexity. However, when dealing with a large number of objects with similar characteristics, Flyweight is a great choice.
By understanding the intrinsic and extrinsic state separation, you can effectively implement the Flyweight pattern in Kotlin to build more efficient applications.