A few days ago, I shared my thoughts on the Adapter Design Pattern—where I noticed it seamlessly bridges the gap between different systems. Now, as I dive into the Bridge Design Pattern, I see it’s more than just about bridging gaps; it’s about creating flexibility, decoupling abstraction from implementation, and making your code as adaptable as possible.
In this blog, we’ll explore:
- What exactly is the Bridge Design Pattern?
- Its structure and how it works under the hood
- Practical, real-world examples that bring the concept to life
- And perhaps most importantly, how it differs from the Adapter Pattern (because, yes, there’s a key difference!).
So, let’s dive in and discover what makes the Bridge Design Pattern a game-changer in clean, scalable software architecture.
What is the Bridge Design Pattern?
Let’s start with the basics: the Bridge Design Pattern is a structural pattern designed to decouple an abstraction from its implementation. By separating these two components, you can vary them independently, which enhances the system’s flexibility and scalability. This means you can extend either the abstraction or the implementation without disrupting the existing system.
In simpler terms, the Bridge Pattern allows you to modify what your code does without affecting how it does it. If that sounds confusing, don’t worry; it will become clearer as we go on.
Essentially, the Bridge Pattern promotes object composition over inheritance, making it particularly useful when dealing with complex class hierarchies that can lead to a proliferation of subclasses as variations increase.
Why is this important? As your project grows, you might find that every new feature requires adjustments to existing code, which can quickly lead to chaos. The Bridge Pattern helps you avoid this mess by keeping your code flexible and easier to manage.
Why Use the Bridge Design Pattern?
Here’s an example you might relate to: imagine you’re building a simple drawing app. You have different shapes—let’s say Circle
and Rectangle
. You also want to paint them in different colors, like Red
and Green
. Sounds easy, right? But if you approach this by creating classes like RedCircle
, GreenRectangle
, GreenCircle
, etc., you’ll quickly end up with a ton of redundant classes.
Shape
|
----------------|----------------
| |
Rectangle Circle
| |
------------- -------------
| | | |
RedRectangle GreenRectangle RedCircle GreenCircle
With Bridge Pattern
Shape
|
---------------|-----------------
| |
Rectangle (Color) Circle (Color)
-------- Color ----------
| |
Red Green
Enter the Bridge Pattern. It allows you to keep the Shape
and Color
separate so that you can easily mix and match them without creating dozens of new classes. It’s like having a separate “shape drawer” and “color palette” that you can combine however you like.
Components of the Bridge Design Pattern
The Bridge Design Pattern is a structural pattern that decouples an abstraction from its implementation, allowing them to vary independently. This pattern is particularly useful when you want to avoid a proliferation of classes that arise from combining multiple variations of abstractions and implementations.
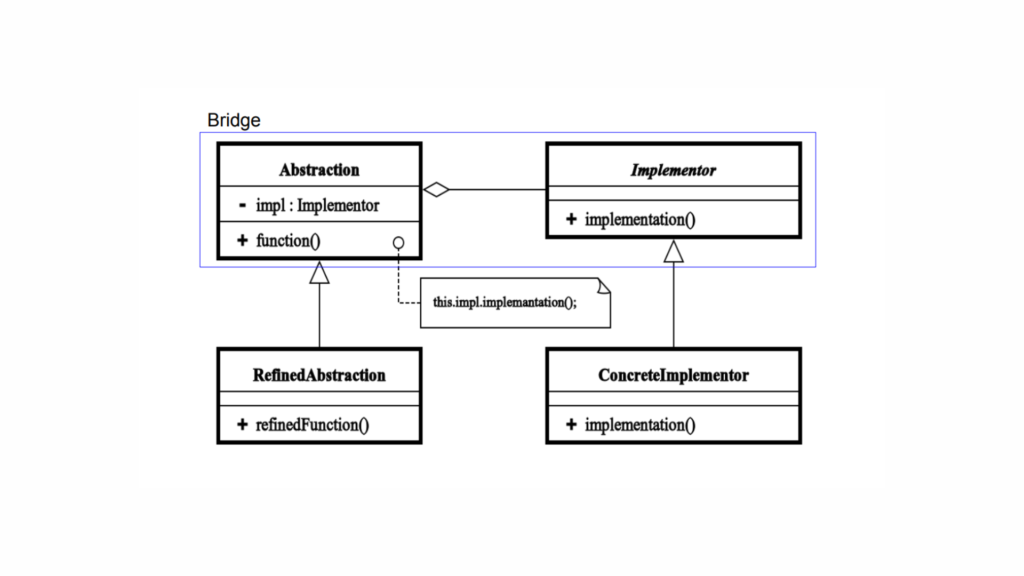
Here,
- Abstraction: This defines the abstract interface and contains a reference to the implementer. The abstraction typically provides a higher-level interface that clients use.
- Refined Abstraction: This extends the abstraction and may provide additional functionality or specificity. It can also override behaviors defined in the abstraction.
- Implementer: This defines the interface for the implementation classes. It does not have to match the abstraction interface; in fact, it can be quite different. The implementer interface can have multiple implementations.
- Concrete Implementers: These are specific implementations of the implementer interface. Each concrete implementer provides a different implementation of the methods defined in the implementer interface.
How the Bridge Pattern Works
- Decoupling: The Bridge Pattern decouples the abstraction from the implementation. This means that you can change or extend either side independently.
- Client Interaction: The client interacts with the abstraction interface, and the abstraction can delegate calls to the implementation without the client needing to know about it.
- Flexibility: You can add new abstractions and implementations without modifying existing code, promoting adherence to the Open/Closed Principle.
Let’s take a more detailed example to illustrate how the Bridge Pattern works.
Example: Shapes and Colors
Scenario: You are building a drawing application that allows users to create shapes with different colors.
Abstraction: Shape
- Methods:
draw()
Refined Abstraction: Circle
and Rectangle
- Each shape has a
draw()
method that uses the color implementation.
Implementer: Color
- Method:
fill()
Concrete Implementers: Red
and Green
- Each color has a specific implementation of the
fill()
method.
// Implementer interface
interface Color {
fun fill()
}
// Concrete Implementers
class Red : Color {
override fun fill() {
println("Filling with Red color.")
}
}
class Green : Color {
override fun fill() {
println("Filling with Green color.")
}
}
// Abstraction
abstract class Shape(protected val color: Color) {
abstract fun draw()
}
// Refined Abstraction
class Circle(color: Color) : Shape(color) {
override fun draw() {
print("Drawing Circle. ")
color.fill()
}
}
class Rectangle(color: Color) : Shape(color) {
override fun draw() {
print("Drawing Rectangle. ")
color.fill()
}
}
// Client Code
fun main() {
val redCircle: Shape = Circle(Red())
redCircle.draw() // Output: Drawing Circle. Filling with Red color.
val greenRectangle: Shape = Rectangle(Green())
greenRectangle.draw() // Output: Drawing Rectangle. Filling with Green color.
}
- Separation of Concerns: The Bridge Pattern promotes separation of concerns by dividing the abstraction from its implementation.
- Flexibility and Extensibility: It provides flexibility and extensibility, allowing new abstractions and implementations to be added without modifying existing code.
- Avoiding Class Explosion: It helps in avoiding class explosion that occurs when you have multiple variations of abstractions and implementations combined together.
The Bridge Design Pattern is particularly useful in scenarios where you want to manage multiple dimensions of variability, providing a clean and maintainable code structure.
Real World Example
Let’s consider a scenario where we are building a notification system. We have different types of notifications (e.g., Email, SMS, Push Notification), and each notification can be sent for different platforms (e.g., Android, iOS).
If we don’t use the Bridge pattern, we might end up with a class hierarchy like this:
AndroidEmailNotification
IOSEmailNotification
AndroidSMSNotification
IOSMSNotification
- …
This quickly becomes cumbersome and difficult to maintain as the number of combinations increases. The Bridge Design Pattern helps us to handle such cases more efficiently by separating the notification type (abstraction) from the platform (implementation).
Before implementing, here’s a quick recap of what the components of the Bridge Pattern are.
- Abstraction: Defines the high-level interface.
- Refined Abstraction: Extends the abstraction and adds additional operations.
- Implementor: Defines the interface for implementation classes.
- Concrete Implementor: Provides concrete implementations of the implementor interface.
Let’s implement the Bridge Design Pattern in Kotlin for our notification system.
Step 1: Define the Implementor (Platform Interface)
interface NotificationSender {
fun sendNotification(message: String)
}
The NotificationSender
interface acts as the Implementor. It defines the method sendNotification()
that will be implemented by concrete platform-specific classes.
Step 2: Concrete Implementors (Platform Implementations)
Now, we’ll create concrete classes that implement NotificationSender
for different platforms.
class AndroidNotificationSender : NotificationSender {
override fun sendNotification(message: String) {
println("Sending notification to Android device: $message")
}
}
class IOSNotificationSender : NotificationSender {
override fun sendNotification(message: String) {
println("Sending notification to iOS device: $message")
}
}
Here, AndroidNotificationSender
and IOSNotificationSender
are Concrete Implementors. They provide platform-specific ways of sending notifications.
Step 3: Define the Abstraction (Notification)
Next, we define the Notification
class, which represents the Abstraction. It contains a reference to the NotificationSender
interface.
abstract class Notification(protected val sender: NotificationSender) {
abstract fun send(message: String)
}
The Notification
class holds a sender
object that allows it to delegate the task of sending notifications to the appropriate platform implementation.
Step 4: Refined Abstractions (Different Types of Notifications)
Now, we’ll create refined abstractions for different types of notifications.
class EmailNotification(sender: NotificationSender) : Notification(sender) {
override fun send(message: String) {
println("Email Notification:")
sender.sendNotification(message)
}
}
class SMSNotification(sender: NotificationSender) : Notification(sender) {
override fun send(message: String) {
println("SMS Notification:")
sender.sendNotification(message)
}
}
Here, EmailNotification
and SMSNotification
extend the Notification
class and specify the type of notification. They use the sender
to send the actual message via the appropriate platform.
Step 5: Putting It All Together
Let’s see how we can use the Bridge Design Pattern in action:
fun main() {
val androidSender = AndroidNotificationSender()
val iosSender = IOSNotificationSender()
val emailNotification = EmailNotification(androidSender)
emailNotification.send("You've got mail!")
val smsNotification = SMSNotification(iosSender)
smsNotification.send("You've got a message!")
}
Output
Email Notification:
Sending notification to Android device: You've got mail!
SMS Notification:
Sending notification to iOS device: You've got a message!
What’s happening here?
- We created an
AndroidNotificationSender
andIOSNotificationSender
for the platforms. - Then, we created
EmailNotification
andSMSNotification
to handle the type of message. - Finally, we sent notifications to both Android and iOS devices using the same abstraction, but different platforms.
Advantages of Bridge Design Pattern
The Bridge Design Pattern provides several advantages:
- Decoupling Abstraction and Implementation: You can develop abstractions and implementations independently. Changes to one won’t affect the other.
- Improved Flexibility: The pattern allows you to extend either the abstraction or the implementation without affecting the rest of the codebase.
- Reduced Class Explosion: It prevents an explosion of subclasses that would otherwise occur with direct inheritance.
- Better Maintainability: Since abstraction and implementation are separated, code becomes cleaner and easier to maintain.
Adapter & Bridge: Difference in Intent
When it comes to design patterns, understanding the difference between the Adapter and Bridge patterns is crucial for effective software development. The Adapter pattern focuses on resolving incompatibilities between two existing interfaces, allowing them to work together seamlessly. In this scenario, the two interfaces operate independently, enabling them to evolve separately over time. However, the coupling between them can be unforeseen, which may lead to complications down the road. On the other hand, the Bridge pattern takes a different approach by connecting an abstraction with its various implementations. This pattern ensures that the evolution of the implementations aligns with the base abstraction, creating a more cohesive structure. In this case, the coupling between the abstraction and its implementations is well-defined and intentional, promoting better maintainability and flexibility. By understanding these distinctions, developers can choose the right pattern based on their specific needs, leading to more robust and adaptable code.
When to Use the Bridge Pattern
Consider using the Bridge Pattern in the following scenarios:
- When your system has multiple dimensions of variations (like different shapes and colors), and you want to minimize subclassing.
- When you need to decouple abstraction from implementation, allowing both to evolve independently.
- When you want to reduce the complexity of a class hierarchy that would otherwise grow out of control with multiple subclasses.
Conclusion
The Bridge Design Pattern is a lifesaver when you have multiple dimensions that need to change independently. By separating the abstraction (what you want to do) from the implementation (how you do it), this pattern ensures your code remains flexible, clean, and easy to extend.
In our notification system example, we applied the pattern, but it can also be used in countless other scenarios, such as database drivers, payment gateways, or even UI frameworks.
Hopefully, this guide has given you a solid understanding of the Bridge Pattern in Kotlin. I encourage you to implement it in your projects and feel free to adapt it as needed!