Design patterns can sometimes seem like fancy terms that only software architects care about. But the truth is, they solve real problems we encounter while coding. One such pattern is the Prototype Design Pattern. It might sound like something from a sci-fi movie where scientists clone people or dinosaurs—but don’t worry, we’re not cloning dinosaurs here! We’re just cloning objects.
Design patterns can be tricky to grasp at first. But imagine a world where you can create duplicates of objects, complete with all their properties, without the hassle of building them from scratch every time. Sounds cool, right? That’s exactly what the Prototype Design Pattern does—it’s like using the cloning feature for your favorite video game character. 🎮
In this blog, we’ll explore the Prototype Pattern in Kotlin, break down its key components, and have some fun with code examples. By the end, you’ll know how to clone objects like a pro (without needing to master dark magic or science fiction). Let’s jump right in!
What is the Prototype Design Pattern?
Imagine you’re making an army of robots 🦾 for world domination. You have a base robot design, but each robot should have its unique characteristics (maybe different colors, weapons, or dance moves 💃). Creating every robot from scratch seems exhausting. What if you could just make a copy, tweak the details, and deploy? That’s the Prototype Design Pattern!
The Prototype Pattern allows you to create new objects by copying existing ones (called prototypes). This approach is super useful when object creation is costly, and you want to avoid all the drama of reinitializing or setting up.
TL;DR:
- Purpose: To avoid the cost of creating objects from scratch.
- How: By cloning existing objects.
- When: Use when object creation is expensive or when we want variations of an object with minor differences.
Since we’re diving into the world of object cloning, let’s first take a good look at how it works. Think of it as learning the basics of cloning before you start creating your own army of identical robots—just to keep things interesting!
Clonning & The Clone Wars ⚔️
The core concept in the Prototype Pattern is the Cloneable
interface. In many programming languages, including Java, objects that can be cloned implement this interface. The clone()
method typically provides the mechanism for creating a duplicate of an object.
The Cloneable
interface ensures that the class allows its objects to be cloned and defines the basic behavior for cloning. By default, this usually results in a shallow copy of the object.
Hold on! Before you start cloning like there’s no tomorrow, it’s essential to grasp the difference between shallow copies and deep copies, as they can significantly affect how your clones behave.
Shallow vs. Deep Copying
Shallow Copy: In a shallow clone, only the object itself is copied, but any references to other objects remain shared. For instance, if your object has a list or an array, only the reference to that list is copied, not the actual list elements. When we clone an object, we only copy the top-level fields. If the object contains references to other objects (like arrays or lists), those references are shared, not copied. It’s like making photocopies of a contract but using the same pen to sign all of them. Not cool.
Deep Copy: In contrast, deep cloning involves copying not just the object but also all objects that it references. All objects, including the nested ones, are fully cloned. In this case, each contract gets its own pen. Much cooler.
I’ve already written a detailed article on this topic. Please refer to it if you want to dive deeper and gain full control over the concept.
Structure of the Prototype Design Pattern
The Prototype Design Pattern consists of a few key components that work together to facilitate object cloning. Here’s a breakdown:
- Prototype Interface: This defines the
clone()
method, which is responsible for cloning objects. - Concrete Prototype: This class implements the
Prototype
interface and provides the actual logic for cloning itself. - Client: The client code interacts with the prototype to create clones of existing objects, avoiding the need to instantiate new objects from scratch.
In Kotlin, you can use the Cloneable
interface to implement the prototype pattern.
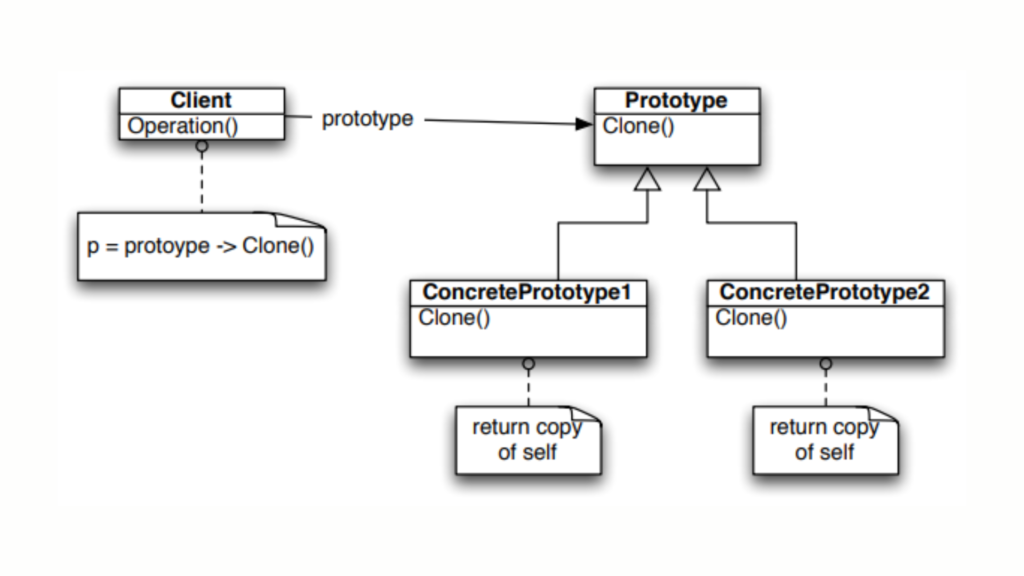
In this typical UML diagram for the Prototype Pattern, you would see the following components:
- Prototype (interface): Defines the contract for cloning.
- Concrete Prototype (class): Implements the clone method to copy itself.
- Client (class): Interacts with the prototype interface to get a cloned object.
How the Prototype Pattern Works
As we now know, the Prototype pattern consists of the following components:
- Prototype: This is an interface or abstract class that defines a method to clone objects.
- Concrete Prototype: These are the actual classes that implement the clone functionality. Each class is responsible for duplicating its instances.
- Client: The client class, which creates new objects by cloning prototypes rather than calling constructors.
In Kotlin, you can use the Cloneable
interface to implement the prototype pattern.
Implementing Prototype Pattern in Kotlin
Let’s go through a practical example of how to implement the Prototype Design Pattern in Kotlin.
Step 1: Define the Prototype Interface
Kotlin has a Cloneable
interface that indicates an object can be cloned, but the clone()
method is not defined in Cloneable
itself. Instead, you need to override the clone()
method from the Java Object
class in a class that implements Cloneable
.
Please note that you won’t see any explicit import statement when using Cloneable
and the clone()
method in Kotlin. This is because both Cloneable
and clone()
are part of the Java standard library, which is automatically available in Kotlin without requiring explicit imports.
interface Prototype : Cloneable {
public override fun clone(): Prototype
}
In the above code, we define the Prototype
interface and inherit the Cloneable
interface, which allows us to override the clone()
method.
Step 2: Create Concrete Prototypes
Now, let’s create concrete implementations of the Prototype. These classes will define the actual objects we want to clone.
data class Circle(var radius: Int, var color: String) : Prototype {
override fun clone(): Circle {
return Circle(this.radius, this.color)
}
fun draw() {
println("Drawing Circle with radius $radius and color $color")
}
}
data class Rectangle(var width: Int, var height: Int, var color: String) : Prototype {
override fun clone(): Rectangle {
return Rectangle(this.width, this.height, this.color)
}
fun draw() {
println("Drawing Rectangle with width $width, height $height, and color $color")
}
}
Here, we have two concrete classes, Circle
and Rectangle
. Both classes implement the Prototype
interface and override the clone()
method to return a copy of themselves.
Circle
has propertiesradius
andcolor
.Rectangle
has propertieswidth
,height
, andcolor
.
Each class has a draw()
method for demonstration purposes to show the state of the object.
Step 3: Using the Prototype Pattern
Now that we have our prototype objects (Circle
and Rectangle
), we can clone them to create new objects.
fun main() {
// Create an initial circle prototype
val circle1 = Circle(5, "Red")
circle1.draw() // Output: Drawing Circle with radius 5 and color Red
// Clone the circle to create a new circle
val circle2 = circle1.clone()
circle2.color = "Blue" // Change the color of the cloned circle
circle2.draw() // Output: Drawing Circle with radius 5 and color Blue
// Create an initial rectangle prototype
val rectangle1 = Rectangle(10, 20, "Green")
rectangle1.draw() // Output: Drawing Rectangle with width 10, height 20, and color Green
// Clone the rectangle and modify its width
val rectangle2 = rectangle1.clone()
rectangle2.width = 15
rectangle2.draw() // Output: Drawing Rectangle with width 15, height 20, and color Green
}
Explanation:
Creating a Prototype (circle1
): We create a Circle
object with a radius of 5 and color "Red"
.
Cloning the Prototype (circle2
): Instead of creating another circle object from scratch, we clone circle1
using the clone()
method. We change the color of the cloned circle to "Blue"
to show that it is a different object from the original one.
Creating a Rectangle Prototype: Similarly, we create a Rectangle
object with a width of 10, height of 20, and color "Green"
.
Cloning the Rectangle (rectangle2
): We then clone the rectangle and modify the width of the cloned object.
Why Use Prototype?
You might be wondering, “Why not just create new objects every time?” Here are a few good reasons:
- Efficiency: Some objects are expensive to create. Think of database records or UI elements with lots of configurations. Cloning is faster than rebuilding.
- Avoid Complexity: If creating an object involves many steps (like baking a cake), cloning helps you avoid repeating those steps.
- Customization: You can create a base object and clone it multiple times, tweaking each clone to suit your needs (like adding more chocolate chips to a clone of a cake).
How the pattern works in Kotlin in a more efficient and readable way
Kotlin makes the implementation of the Prototype Pattern easy and concise with its support for data classes and the copy()
function. The copy function can create new instances of objects with the option to modify fields during copying.
Here’s a basic structure of the Prototype Pattern in Kotlin:
interface Prototype : Cloneable {
fun clone(): Prototype
}
data class GameCharacter(val name: String, val health: Int, val level: Int): Prototype {
override fun clone(): GameCharacter {
return copy() // This Kotlin function creates a clone
}
}
fun main() {
val originalCharacter = GameCharacter(name = "Hero", health = 100, level = 1)
// Cloning the original character
val clonedCharacter = originalCharacter.clone()
// Modifying the cloned character
val modifiedCharacter = clonedCharacter.copy(name = "Hero Clone", level = 2)
println("Original Character: $originalCharacter")
println("Cloned Character: $clonedCharacter")
println("Modified Character: $modifiedCharacter")
}
//Output
Original Character: GameCharacter(name=Hero, health=100, level=1)
Cloned Character: GameCharacter(name=Hero, health=100, level=1)
Modified Character: GameCharacter(name=Hero Clone, health=100, level=2)
Here, we can see how the clone
method creates a new instance of GameCharacter
with the same attributes as the original. The modified character shows that you can change attributes of the cloned instance without affecting the original. This illustrates the Prototype pattern’s ability to create new objects by copying existing ones.
Real-World Use Cases
Creating a Prototype for Game Characters
In a game development scenario, characters often share similar configurations with slight variations. The Prototype Pattern allows the game engine to create these variations without expensive initializations.
For instance, consider a game where you need multiple types of warriors, all with the same base stats but slightly different weapons. Instead of creating new instances from scratch, you can clone a base character and modify the weapon or other attributes.
Now, let’s dive into some Kotlin code and see how we can implement the Prototype Pattern like Kotlin rockstars! 🎸
Step 1: Define the Prototype Interface
We’ll start by creating an interface that all objects (robots, in this case) must implement if they want to be “cloneable.”
interface CloneablePrototype : Cloneable{
fun clone(): CloneablePrototype
}
Simple, right? This CloneablePrototype
interface has one job: provide a method to clone objects.
Step 2: Concrete Prototype (Meet the Robots!)
Let’s create some robots. Here’s a class for our robot soldiers:
data class Robot(
var name: String,
var weapon: String,
var color: String
) : CloneablePrototype {
override fun clone(): Robot {
return Robot(name, weapon, color)
// Note: We could directly use copy() here, but for better understanding, we went with the constructor approach.
}
override fun toString(): String {
return "Robot(name='$name', weapon='$weapon', color='$color')"
}
}
Here’s what’s happening:
- We use Kotlin’s
data class
to make life easier (no need to manually implementequals
,hashCode
, ortoString
). - The
clone()
method returns a newRobot
object with the same attributes as the current one. It’s a perfect copy—like sending a robot through a 3D printer! - The
toString()
method is overridden to give a nice string representation of the robot (for easier debugging and bragging rights).
Step 3: Let’s Build and Clone Our Robots
Let’s simulate an evil villain building an army of robot clones. 🤖
fun main() {
// The original prototype robot
val prototypeRobot = Robot(name = "T-1000", weapon = "Laser Gun", color = "Silver")
// Cloning the robot
val robotClone1 = prototypeRobot.clone().apply {
name = "T-2000"
color = "Black"
}
val robotClone2 = prototypeRobot.clone().apply {
name = "T-3000"
weapon = "Rocket Launcher"
}
println("Original Robot: $prototypeRobot")
println("First Clone: $robotClone1")
println("Second Clone: $robotClone2")
}
Here,
- We start with an original prototype robot (
T-1000
) equipped with a laser gun and shiny silver armor. - Next, we clone it twice. Each time, we modify the clone slightly. One gets a name upgrade and a paint job, while the other gets an epic weapon upgrade. After all, who doesn’t want a rocket launcher?
Output:
Original Robot: Robot(name='T-1000', weapon='Laser Gun', color='Silver')
First Clone: Robot(name='T-2000', weapon='Laser Gun', color='Black')
Second Clone: Robot(name='T-3000', weapon='Rocket Launcher', color='Silver')
Just like that, we’ve created a robot army with minimal effort. They’re all unique, but they share the same essential blueprint. The evil mastermind can sit back, relax, and let the robots take over the world (or maybe start a dance-off).
Cloning a Shape Object in a Drawing Application
In many drawing applications like Adobe Illustrator or Figma, you can create different shapes (e.g., circles, rectangles) and duplicate them. The Prototype pattern can be used to clone these shapes without re-creating them from scratch.
// Prototype interface with a clone method
interface Shape : Cloneable {
fun clone(): Shape
}
// Concrete Circle class implementing Shape
class Circle(var radius: Int) : Shape {
override fun clone(): Shape {
return Circle(this.radius) // Cloning the current object
}
override fun toString(): String {
return "Circle(radius=$radius)"
}
}
// Concrete Rectangle class implementing Shape
class Rectangle(var width: Int, var height: Int) : Shape {
override fun clone(): Shape {
return Rectangle(this.width, this.height) // Cloning the current object
}
override fun toString(): String {
return "Rectangle(width=$width, height=$height)"
}
}
fun main() {
val circle1 = Circle(10)
val circle2 = circle1.clone() as Circle
println("Original Circle: $circle1")
println("Cloned Circle: $circle2")
val rectangle1 = Rectangle(20, 10)
val rectangle2 = rectangle1.clone() as Rectangle
println("Original Rectangle: $rectangle1")
println("Cloned Rectangle: $rectangle2")
}
Here, we define a Shape
interface with a clone()
method. The Circle
and Rectangle
classes implement this interface and provide their own cloning logic.
Duplicating User Preferences in a Mobile App
In mobile applications, user preferences might be complex to initialize. The Prototype pattern can be used to clone user preference objects when creating new user profiles or settings.
// Prototype interface with a clone method
interface UserPreferences : Cloneable {
fun clone(): UserPreferences
}
// Concrete class implementing UserPreferences
class Preferences(var theme: String, var notificationEnabled: Boolean) : UserPreferences {
override fun clone(): UserPreferences {
return Preferences(this.theme, this.notificationEnabled) // Cloning current preferences
}
override fun toString(): String {
return "Preferences(theme='$theme', notificationEnabled=$notificationEnabled)"
}
}
fun main() {
// Original preferences
val defaultPreferences = Preferences("Dark", true)
// Cloning the preferences for a new user
val user1Preferences = defaultPreferences.clone() as Preferences
user1Preferences.theme = "Light" // Customizing for this user
println("Original Preferences: $defaultPreferences")
println("User 1 Preferences: $user1Preferences")
}
Here, the Preferences
object for a user can be cloned when new users are created, allowing the same structure but with different values (like changing the theme).
Cloning Product Prototypes in an E-commerce Platform
An e-commerce platform can use the Prototype pattern to create product variants (e.g., different sizes or colors) by cloning an existing product prototype instead of creating a new product from scratch.
// Prototype interface with a clone method
interface Product : Cloneable {
fun clone(): Product
}
// Concrete class implementing Product
class Item(var name: String, var price: Double, var color: String) : Product {
override fun clone(): Product {
return Item(this.name, this.price, this.color) // Cloning the current product
}
override fun toString(): String {
return "Item(name='$name', price=$price, color='$color')"
}
}
fun main() {
// Original product
val originalProduct = Item("T-shirt", 19.99, "Red")
// Cloning the product for a new variant
val newProduct = originalProduct.clone() as Item
newProduct.color = "Blue" // Changing color for the new variant
println("Original Product: $originalProduct")
println("New Product Variant: $newProduct")
}
In this case, an e-commerce platform can clone the original Item
(product) and modify attributes such as color, without needing to rebuild the entire object.
Advantages and Disadvantages of the Prototype Pattern
Advantages
- Performance optimization: It reduces the overhead of creating complex objects by reusing existing ones.
- Simplified object creation: If the initialization of an object is costly or complex, the prototype pattern makes it easy to create new instances.
- Dynamic customization: You can dynamically modify the cloned objects without affecting the original ones.
Disadvantages
- Shallow vs. Deep Copy: By default, cloning in Kotlin creates shallow copies, meaning that the objects’ properties are copied by reference. You may need to implement deep copying if you want fully independent copies of objects.
- Implementation complexity: Implementing cloneable classes with deep copying logic can become complex, especially if the objects have many nested fields.
Conclusion
The Prototype Design Pattern is a fantastic way to avoid repetitive object creation, especially when those objects are complex or expensive to initialize. It’s perfect for scenarios where you need similar, but slightly different, objects (like our robots!).
So next time you need a robot army, a game character, or even a fleet of space ships, don’t reinvent the wheel—clone it! Just make sure to avoid shallow copies unless you want robots sharing the same laser gun (that could get awkward real fast).
Happy Cloning! ✨
Feel free to share your thoughts, or if your robot clones start acting weird, you can always ask for help. 😅