Kotlin is a modern, statically typed programming language that runs on the Java Virtual Machine (JVM). One of the language’s powerful features is reflection, which allows you to examine and manipulate the structure of your code at runtime. Kotlin reflection provides a set of APIs that enable introspection, dynamic loading, and modification of classes, objects, properties, and functions. In this blog post, we will delve into the world of Kotlin reflection, exploring its various aspects and providing examples to help you understand its capabilities.
Basics of Reflection in Kotlin
Reflection in Kotlin allows you to access properties and methods of objects dynamically at runtime, without knowing them in advance. Normally, when you access a method or property, your program’s source code references a specific declaration, and the compiler ensures that the declaration exists. However, there are situations where you need to work with objects of any type or where the names of methods and properties are only known at runtime. This is where reflection comes in handy.
In Kotlin, there are two reflection APIs you can work with. The first one is the standard Java reflection API, which is defined in java.lang.reflect
package. Since Kotlin classes are compiled to regular Java bytecode, the Java reflection API works perfectly with Kotlin. This means that Java libraries that use reflection are fully compatible with Kotlin code.
The second reflection API is the Kotlin reflection API, defined in the kotlin.reflect
package. This API provides access to concepts that don’t exist in the Java world, such as properties and nullable types. However, it doesn’t fully replace the Java reflection API, and there may be cases where you need to use Java reflection instead. It’s important to note that the Kotlin reflection API is not restricted to Kotlin classes alone; you can use it to access classes written in any JVM language.
Let’s take an example to understand how reflection can be useful. Suppose you have a JSON serialization library that needs to serialize any object to JSON. Since the library can’t reference specific classes and properties, it relies on reflection to dynamically access and serialize the object’s properties at runtime. This allows the library to handle objects of different types without having prior knowledge about their structure.
Here’s a simplified example of using reflection in Kotlin to access the properties of an object dynamically:
data class Person(val name: String, val age: Int)
fun main() {
val person = Person("Alice", 25)
val properties = person.javaClass.declaredFields
for (property in properties) {
property.isAccessible = true
val value = property.get(person)
println("${property.name}: $value")
}
}
In this example, we have a Person
class with two properties: name
and age
. Using reflection, we retrieve the declared fields (name
and age
) of the person
object dynamically. By setting the isAccessible
property to true
, we ensure that we can access private fields as well. Finally, we get the values of the properties using the get()
method and print them.
JVM dependency
To add the Kotlin reflection library as a dependency in your JVM project, you’ll need to include the kotlin-reflect
artifact. Here’s how you can do it in Gradle and Maven:
In Gradle:
dependencies {
implementation "org.jetbrains.kotlin:kotlin-reflect:1.9.0"
}
In Maven:
<dependencies>
<dependency>
<groupId>org.jetbrains.kotlin</groupId>
<artifactId>kotlin-reflect</artifactId>
<version>1.9.0</version>
</dependency>
</dependencies>
Make sure to replace the version (1.9.0
in this example) with the version you desire to use or the latest updated one.
If you’re not using Gradle or Maven, ensure that the kotlin-reflect.jar
is present in the classpath of your project. For IntelliJ IDEA projects that use the command-line compiler or Ant, the reflection library is automatically added by default. However, in the command-line compiler and Ant, you can use the -no-reflect
compiler option to exclude kotlin-reflect.jar
from the classpath if you don’t need it.
Note that, to reduce the runtime library size on platforms where it matters, such as Android, the Kotlin reflection API is packaged into a separate .jar file, kotlin-reflect.jar, which isn’t added to the dependencies of new projects by default. If you’re using the Kotlin reflection API, you need to make sure the library is added as a dependency. IntelliJ IDEA can detect the missing dependency and assist you with adding it.
Kotlin reflection API
The Kotlin reflection API provides a set of classes and interfaces that allow you to inspect and manipulate the structure and behavior of Kotlin classes at runtime. Here are the main components of the Kotlin reflection API that you mentioned:
KClass
In Kotlin, the main entry point for the reflection API is the KClass
interface, which represents a class. It serves as a counterpart to Java’s java.lang.Class
and allows you to perform various reflection operations.
To obtain an instance of KClass
, you can use the ::class
syntax on a class name. For example, if you have a class named MyClass
, you can get its KClass
instance like this:
val myClassKClass: KClass<MyClass> = MyClass::class
Once you have a KClass
instance, you can use it to enumerate and access the declarations contained within the class, its superclasses, interfaces, and so on. The KClass
interface provides functions and properties to perform these operations.
To get the KClass
of an object at runtime, you can use the javaClass
property, which returns the corresponding Java class. Then, you can access the .kotlin
extension property on the Java class to obtain the Kotlin reflection counterpart (KClass
). Here’s an example:
val obj = MyClass()
val objKClass: KClass<out MyClass> = obj.javaClass.kotlin
In the above example, obj.javaClass
returns the Java class of the obj
instance, and .kotlin
is used to obtain the corresponding KClass
instance.
Once you have a KClass
instance, you can use it to perform reflection operations such as accessing properties, functions, constructors, annotations, and more.
class Person(val name: String, val age: Int)
val person = Person("Alice", 29)
val kClass = person.javaClass.kotlin
println(kClass.simpleName)
kClass.memberProperties.forEach { println(it.name) }
Output:
Person
age
name
Explanation:
- The
Person
class is defined with two properties:name
of typeString
andage
of typeInt
. - An instance of
Person
namedperson
is created with the values “Alice” and 29 for thename
andage
properties, respectively. person.javaClass
returns the corresponding Java class object for theperson
instance.kotlin
is used as an extension property on the Java class object to obtain theKClass
instance representing thePerson
class, which is assigned to thekClass
variable.kClass.simpleName
prints the simple name of the class, which in this case is “Person”.kClass.memberProperties
returns a collection ofKProperty
objects representing the non-extension properties of the class, including properties defined in its superclasses.- The
forEach
function is used to iterate over eachKProperty
object in the collection and print its name. In this example, it prints “age” and “name”, which are the names of the properties defined in thePerson
class.
By using kClass.memberProperties
, you can retrieve all the non-extension properties defined in a class, including those inherited from its superclasses. This is a useful feature of Kotlin reflection for dynamically inspecting and working with class properties at runtime.
If you browse the declaration of KClass, you’ll see that, KClass
interface in Kotlin reflection provides several useful methods and properties for accessing the contents of a class. Here are some additional features of KClass
:
interface KClass<T : Any> {
val simpleName: String?
val qualifiedName: String?
val members: Collection<KCallable<*>>
val constructors: Collection<KFunction<T>>
val nestedClasses: Collection<KClass<*>>
// ...
}
simpleName
: Returns the simple name of the class as aString
. This property is useful for getting the name of the class without any package information.qualifiedName
: Returns the fully qualified name of the class as aString
, including the package name. This property is useful when you need the complete name of the class, including the package.members
: Returns a collection ofKCallable
objects representing all members (properties, functions, etc.) of the class. This includes both declared members and inherited members from superclasses.constructors
: Returns a collection ofKFunction
objects representing all constructors of the class. This allows you to retrieve information about the constructors and their parameters.nestedClasses
: Returns a collection ofKClass
objects representing all nested classes declared within the class. This is useful if you want to access and work with nested classes.
These are just a few examples of the additional features provided by the KClass
interface. You can find more details and explore other methods and properties available in the Kotlin standard library reference (http://mng.bz/em4i).
KCallable
In Kotlin, when you want to access and call functions or properties dynamically through reflection, you can use the KCallable
interface. KCallable
is a super interface for functions and properties, and it declares the call
method, which allows you to invoke the corresponding function or property getter.
Here’s an example of using the call
method to call a function through reflection:
fun foo(x: Int) = println(x)
val kFunction = ::foo
kFunction.call(42) // Output: 42
In this example, the ::foo
syntax refers to the function foo
and returns an instance of the KFunction
class from the reflection API. By using the call
method on kFunction
, you can invoke the referenced function. In this case, we provide a single argument, 42
.
If you try to call the function with an incorrect number of arguments, such as function.call()
, it will throw a runtime exception with the message “IllegalArgumentException: Callable expects 1 arguments, but 0 were provided.”
To provide better type safety and enforce the correct number of arguments, you can use a more specific method called invoke
. The type of the ::foo
expression, in this case, is KFunction1<Int, Unit>
, which contains information about parameter and return types. The 1 denotes that this function takes one parameter of type Int
. You can then use the invoke
method to call the function with a fixed number of arguments:
import kotlin.reflect.KFunction2
fun sum(x: Int, y: Int) = x + y
val kFunction: KFunction2<Int, Int, Int> = ::sum
println(kFunction.invoke(1, 2) + kFunction(3, 4)) // Output: 10
In the above example, kFunction.invoke(1, 2)
calls the sum
function with arguments 1
and 2
, and kFunction(3, 4)
is a shorthand notation for invoking the function. Since kFunction
is of type KFunction2<Int, Int, Int>
, it only accepts two arguments of type Int
. If you try to call it with an incorrect number of arguments, the code won’t compile.
So, if you have a
KFunction
of a specific type with known parameters and return type, it’s recommended to use itsinvoke
method for type safety. Thecall
method is a more generic approach that can work with any type of function but doesn’t provide the same level of type safety.
BTW, How, and where are KFunctionN interfaces defined?
The KFunctionN
interfaces, such as KFunction1
, KFunction2
, and so on, represent functions with different numbers of parameters. Each of these types extends the KFunction
interface and adds an invoke
member with the corresponding number of parameters.
For example, the KFunction2
interface declares the invoke
method as follows:
operator fun invoke(p1: P1, p2: P2): R
Here, P1
and P2
represent the parameter types of the function, and R
represents the return type.
It’s important to note that these function types, represented by
KFunctionN
, are synthetic compiler-generated types. You won’t find their explicit declarations in thekotlin.reflect
package or any other standard Kotlin library.
The synthetic types approach allows for flexibility in the number of parameters a function type can have. Generating these types dynamically reduces the size of the kotlin-runtime.jar
and avoids imposing artificial restrictions on the maximum number of function-type parameters.
So, the KFunctionN
interfaces are synthetic types created by the compiler to represent functions with different numbers of parameters. They are not explicitly defined in the kotlin.reflect
package, and their generation is based on the function’s parameter and return types.
KProperty
In Kotlin, you can also use reflection to access and retrieve property values dynamically. The KProperty
interface provides methods to achieve this, with different interfaces for top-level properties and member properties.
For top-level properties, you can use instances of the KProperty0
interface, which has a no-argument get
method. Here’s an example:
var counter = 0
val kProperty = ::counter
kProperty.setter.call(21)
println(kProperty.get()) // Output: 21
In this example, kProperty
refers to the top-level property counter
. By calling kProperty.get()
, you retrieve the current value of the property.
For member properties, you need to use the appropriate interface based on the number of arguments the get
method requires. For example, KProperty1
is used for member properties with a single argument. You also need to provide the object instance on which the property is accessed. Here’s an example:
class Person(val name: String, val age: Int)
val person = Person("Alice", 29)
val memberProperty = Person::age
println(memberProperty.get(person)) // Output: 29
In this case, memberProperty
refers to the age
property of the Person
class. By calling memberProperty.get(person)
, you retrieve the value of the age
property for the specific person
instance.
It’s important to note that KProperty1
is a generic class. The type of memberProperty
in the above example is KProperty<Person, Int>
, where the first type parameter represents the type of the receiver (in this case, Person
) and the second type parameter represents the property type (Int
). This ensures that you can only call the get
method with a receiver of the correct type. Attempting to call memberProperty.get("Alice")
would result in a compilation error.
Please keep in mind that reflection can only be used to access properties defined at the top level or within a class, and not local variables within a function. If you try to obtain a reference to a local variable using
::x
, you will encounter a compilation error stating that “References to variables aren’t supported yet”.
Hierarchy of interfaces in Kotlin Reflection API
In Kotlin, there is a hierarchy of interfaces that allows you to access source code elements at runtime. These interfaces are designed to represent declarations and provide access to various information about them.
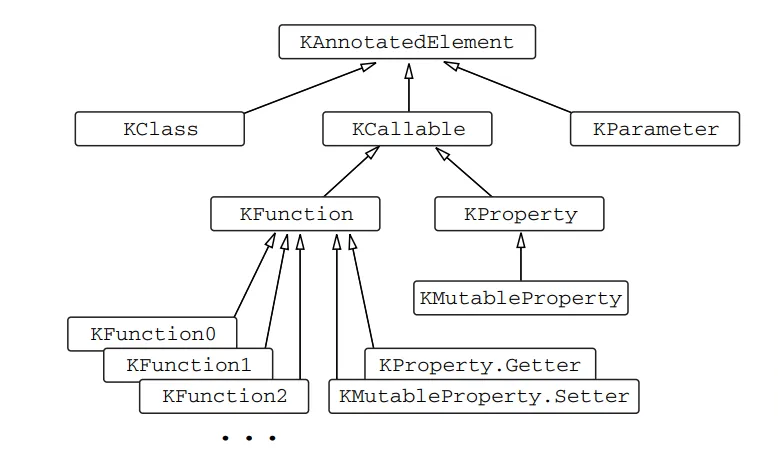
Here’s a breakdown of the key interfaces mentioned:
KAnnotatedElement
: This interface serves as the base for all other interfaces that represent declarations at runtime, such asKClass
,KFunction
, andKParameter
. Since all declarations can be annotated, they inherit this interface to provide access to annotations.KClass
: This interface is used to represent both classes and objects at runtime. It provides access to information about the class or object, such as its name, superclass, interfaces, properties, and functions.KProperty
: This interface represents any property at runtime, regardless of whether it’s mutable (var
) or read-only (val
). It allows you to access information about the property, such as its name, type, annotations, and the getter function.KMutableProperty
: This is a subclass ofKProperty
and specifically represents a mutable property, which is declared usingvar
. It provides additional functionality to modify the property’s value, in addition to the information available throughKProperty
.Getter
andSetter
: These are special interfaces defined inProperty
andKMutableProperty
to work with property accessors as functions. They extend theKFunction
interface, allowing you to access information about the getter and setter functions associated with the property. For example, you can retrieve annotations applied to the getter or setter.
Note that the figure mentions the omission of specific interfaces like
KProperty0
for simplicity.KProperty0
represents a property without any parameters (zero-argument property). Similarly, there are other specific interfaces in the hierarchy that cater to different scenarios and numbers of parameters.
Overall, these interfaces in the hierarchy provide a flexible and comprehensive way to access and manipulate source code elements at runtime, allowing you to retrieve information, modify values, and work with annotations.
Best Practices and Use Cases for Kotlin Reflection:
Serialization and Deserialization
Reflection is commonly used in serialization and deserialization libraries. It allows these libraries to introspect object structures dynamically, enabling automatic conversion between objects and their serialized forms. Reflection facilitates the inspection of object properties and their values, making it easier to transform objects into a serialized format and vice versa.
data class Person(
val name: String,
val age: Int
)
fun main() {
val person = Person("John Doe", 30)
// Serialization using reflection
val serializedData = person::class.memberProperties
.associateBy({ it.name }, { it.get(person) })
println(serializedData) // Output: {name=John Doe, age=30}
// Deserialization using reflection
val deserializedPerson = person::class.constructors.first()
.call(serializedData["name"], serializedData["age"])
println(deserializedPerson) // Output: Person(name=John Doe, age=30)
}
In this example, reflection is used to serialize a Person
object by obtaining its properties using memberProperties
and creating a map of property names and values. The same reflection-based approach is used to deserialize the serialized data back into a Person
object.
Dependency Injection
Frameworks like Spring heavily rely on reflection for implementing dependency injection. Reflection enables these frameworks to scan classes, examine annotations, and wire dependencies at runtime. By leveraging reflection, frameworks can automatically discover and instantiate the required dependencies, reducing the need for manual configuration. Reflection helps in achieving loose coupling and makes the dependency injection process more flexible and adaptable.
class ServiceA {
fun doSomething() {
println("Service A is doing something.")
}
}
class ServiceB {
fun doSomethingElse() {
println("Service B is doing something else.")
}
}
class Client(private val serviceA: ServiceA, private val serviceB: ServiceB) {
fun performActions() {
serviceA.doSomething()
serviceB.doSomethingElse()
}
}
fun main() {
val clientClass = Client::class
val constructors = clientClass.constructors
if (constructors.isNotEmpty()) {
val constructor = constructors.first()
val parameters = constructor.parameters
// Dependency injection using reflection
val serviceA = ServiceA()
val serviceB = ServiceB()
val client = constructor.callBy(mapOf(parameters[0] to serviceA, parameters[1] to serviceB))
client.performActions()
}
}
In this example, reflection is used for dependency injection. The Client
class has dependencies on ServiceA
and ServiceB
. Using reflection, the constructor of Client
is obtained, and the dependencies are instantiated (ServiceA
and ServiceB
). The dependencies are then injected into the Client
object using callBy
and a map of parameter-to-argument pairs.
Testing and Mocking
Reflection is valuable in testing and mocking scenarios. It allows developers to examine and modify internal state, invoke private methods, and create mock objects dynamically. Reflection provides the ability to access and modify otherwise inaccessible members, which is particularly useful for unit testing private methods or creating mock objects with dynamically generated behavior. It simplifies the process of writing test cases and enables comprehensive testing of code components.
class MathUtils {
private fun multiply(a: Int, b: Int): Int {
return a * b
}
fun square(n: Int): Int {
return multiply(n, n)
}
}
fun main() {
val mathUtils = MathUtils()
val multiplyMethod = mathUtils::class.declaredFunctions
.firstOrNull { it.name == "multiply" }
multiplyMethod?.let {
it.isAccessible = true
val result = it.call(mathUtils, 4, 5)
println(result) // Output: 20
}
}
In this example, reflection is used for testing and mocking. The private multiply
method of the MathUtils
class is accessed using reflection by setting its accessibility to true
. The call
method is then used to invoke the method with arguments. This allows us to test the private method’s functionality or mock its behavior in a controlled testing environment.
By following these best practices and leveraging Kotlin reflection in appropriate use cases such as serialization, dependency injection, and testing, developers can harness the full potential of reflection to enhance the flexibility, extensibility, and maintainability of their Kotlin applications.
Limitations of Kotlin Reflection
Reflection has some limitations, such as being unable to access private members by default, requiring additional permissions in security-restricted environments, and being less type-safe than static typing.
The limitations of Kotlin reflection include:
- Performance Overhead: Reflection operations incur performance overhead compared to statically typed code, as they involve runtime introspection and dynamic dispatch.
- Limited Access to Private Members: By default, Kotlin reflection does not provide direct access to private members of classes. Access to private members requires setting accessibility, which may have security implications.
- Security and Permissions: In security-restricted environments, using reflection may require additional permissions or explicit configuration to prevent unauthorized access.
- Type Safety: Reflection is inherently less type-safe compared to static typing. Type errors that would normally be caught by the compiler can only be detected at runtime when using reflection.
- Limited Compile-Time Checks: Reflection bypasses many compile-time checks provided by the Kotlin compiler. Renaming elements accessed via reflection may lead to runtime errors that are not caught during compilation.
- Platform Dependencies: Kotlin reflection relies on platform-specific features and APIs, which may introduce variations in behavior and capabilities across different platforms.
Conclusion
Kotlin reflection provides a powerful set of APIs that enable you to examine and manipulate your code dynamically at runtime. From accessing class information to modifying properties and invoking functions dynamically, reflection opens up a whole new range of possibilities in your Kotlin projects. However, it’s essential to use reflection judiciously and be aware of its performance implications. By understanding the concepts and best practices outlined in this blog post, you’ll be well-equipped to leverage the full potential of Kotlin reflection in your applications.