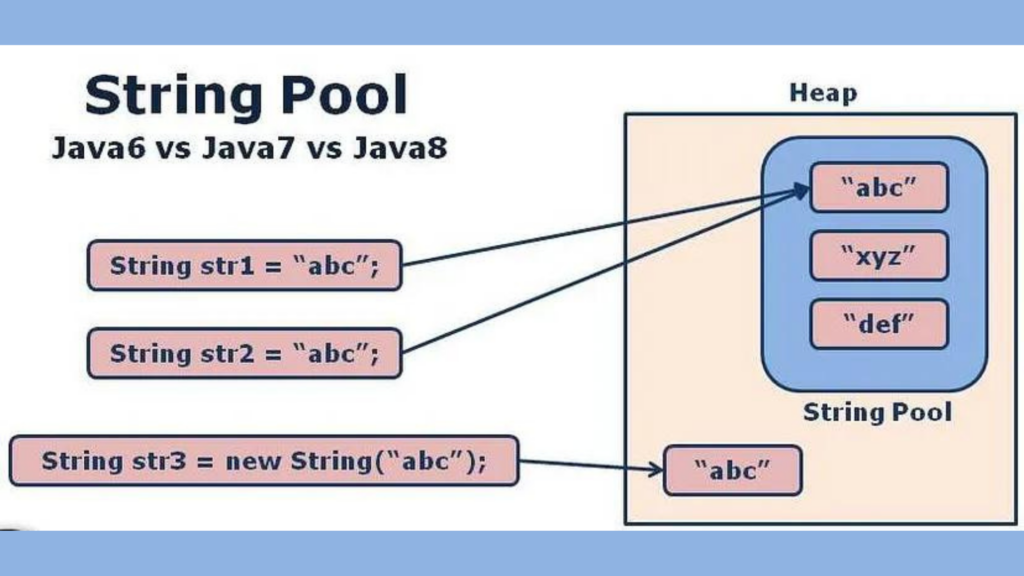
String is a widely used data type in Java, and it has a special place in memory management. In Java, every time a new object is created, it is allocated memory in the heap area. However, Java has a special feature called String Constant Pool (SCP) that allows for efficient memory management of String objects. In this blog post, we will discuss what SCP is, how it works, and how it impacts memory management in Java.
What is Java String Constant Pool (SCP)?
SCP is a special memory area in Java where all String literals are stored. When a String literal is encountered, Java looks for the same String literal in the SCP. If the String literal already exists in SCP, Java uses the existing String literal from SCP. Otherwise, Java creates a new String literal in SCP. This ensures that only one copy of a particular String literal is stored in the memory.
SCP is part of the runtime constant pool, which is a shared pool of constants that are loaded with the class definition. SCP is created when the class is loaded by the JVM and is destroyed when the JVM shuts down. SCP is not accessible by the garbage collector, so even if a String object does not have any references pointing to it, it will not be eligible for garbage collection if it is in SCP.
How SCP works?
SCP works differently for String objects created using the “new” keyword and String literals.
When a String object is created using the “new” keyword, two objects are created: one in the heap area and one in SCP. The reference variable points to the object in the heap area. For example:
String s = new String(“softAai”);
In this case, the String literal “softAai” is created in SCP, and a new String object is created in the heap area. The reference variable “s” points to the String object in the heap area.
On the other hand, when a String literal is encountered, Java checks if the same String literal exists in SCP. If it does, Java uses the existing String literal from SCP. Otherwise, Java creates a new String literal in SCP. For example:
String s = “softAai”;
In this case, the String literal “softAai” is created in SCP, and the reference variable “s” points to the String literal in SCP. No new object is created in the heap area.
Note that object creation in SCP is optional. If a String object with the required content is already present in SCP, the existing object will be reused instead of creating a new object.
Impact on Memory Management
SCP has a significant impact on memory management in Java. When a program uses many String literals, SCP ensures that only one copy of each String literal is stored in the memory, which saves a lot of memory space. This also improves the performance of the program because fewer objects need to be created and garbage collected.
However, if a program creates many String objects using the “new” keyword, SCP does not have a significant impact on memory management. In this case, each String object is created in the heap area, and SCP only contains the String literals.
SCP Limitations
The SCP has some limitations, such as the fact that objects in the SCP cannot be garbage collected, even if they are no longer being used by the program. This can lead to memory leaks if too many objects are created in the SCP. Additionally, because objects in the SCP are shared among all threads in the JVM, changes to these objects made by one thread can affect the behavior of other threads in unpredictable ways.
Despite these limitations, the SCP remains an essential part of Java’s memory management system. Storing string literals and constant values in the SCP can help to reduce memory usage and improve performance by minimizing the number of objects that need to be created. Additionally, the SCP can help to improve string comparison performance by allowing the JVM to compare string objects by reference instead of by value
Conclusion
SCP is a powerful feature of Java that allows for efficient memory management of String objects. By storing String literals in a shared memory area, SCP ensures that only one copy of each String literal is stored in memory, which saves a lot of memory space and improves the performance of the program. While SCP has some limitations, still it remains an essential part of Java’s memory management system.